获取表名:
using Microsoft.EntityFrameworkCore;
var entityType = _context.Model.FindEntityType(typeof(表实体));
var tableName = entityType.GetTableName();
获取字段名:
using Microsoft.EntityFrameworkCore;
var entityType = _context.Model.FindEntityType(typeof(表实体));
var dic = new Dictionary<string, string>();
foreach (var p in entityType.GetProperties())
{
dic.Add(p.Name, p.GetColumnBaseName());
}
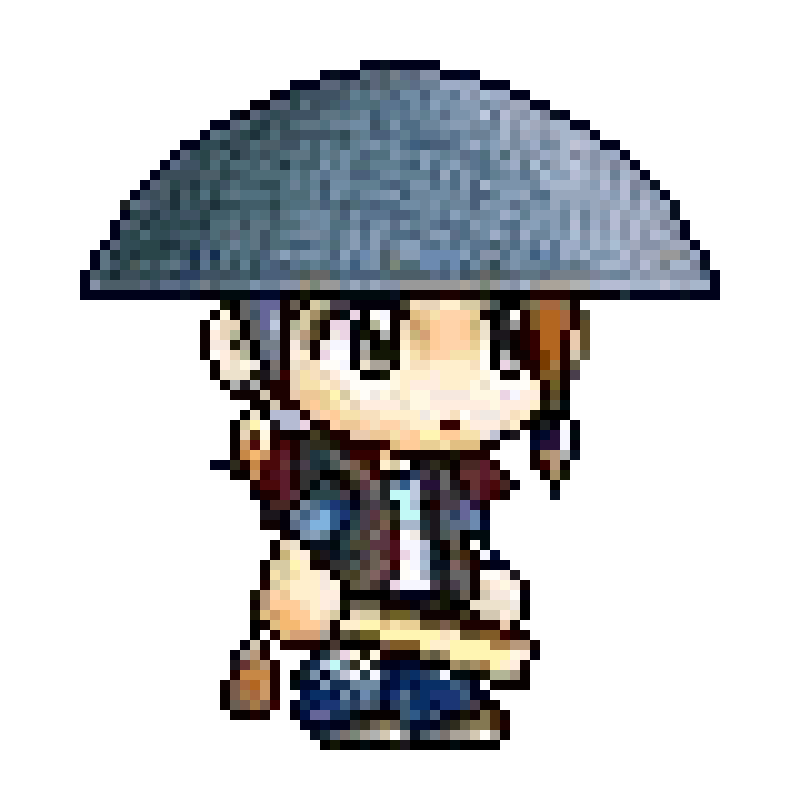
最近,Microsoft 将其针对Web API 的默认序列化从 Newtonsoft JsonConvert 更改为 System.Text.Json JsonSerializer。
using System.Text.Json;
string s = JsonSerializer.Serialize(object);
var obj = JsonSerializer.Deserialize<T>(string);
System.Text.Json 命名空间:https://docs.microsoft.com/zh-cn/dotnet/api/system.text.json?view=net-5.0
如何从 Newtonsoft.Json 迁移到 System.Text.Json:https://docs.microsoft.com/zh-cn/dotnet/standard/serialization/system-text-json-migrate-from-newtonsoft-how-to?pivots=dotnet-5-0
不序列化属性
[System.Text.Json.Serialization.JsonIgnore]
当值为 null 时不序列化
[System.Text.Json.Serialization.JsonIgnore(Condition = JsonIgnoreCondition.WhenWritingNull)]
格式化/美化
System.Text.Json.JsonSerializer.Serialize(Obj, new System.Text.Json.JsonSerializerOptions {
WriteIndented = true
})
不编码中文(建议默认需要编码,可防止页面显示乱码,除非需要放在 <pre /> 标签中直接显示,可友好显示中文)
System.Text.Json.JsonSerializer.Serialize(Obj, new System.Text.Json.JsonSerializerOptions {
Encoder = System.Text.Encodings.Web.JavaScriptEncoder.UnsafeRelaxedJsonEscaping
})
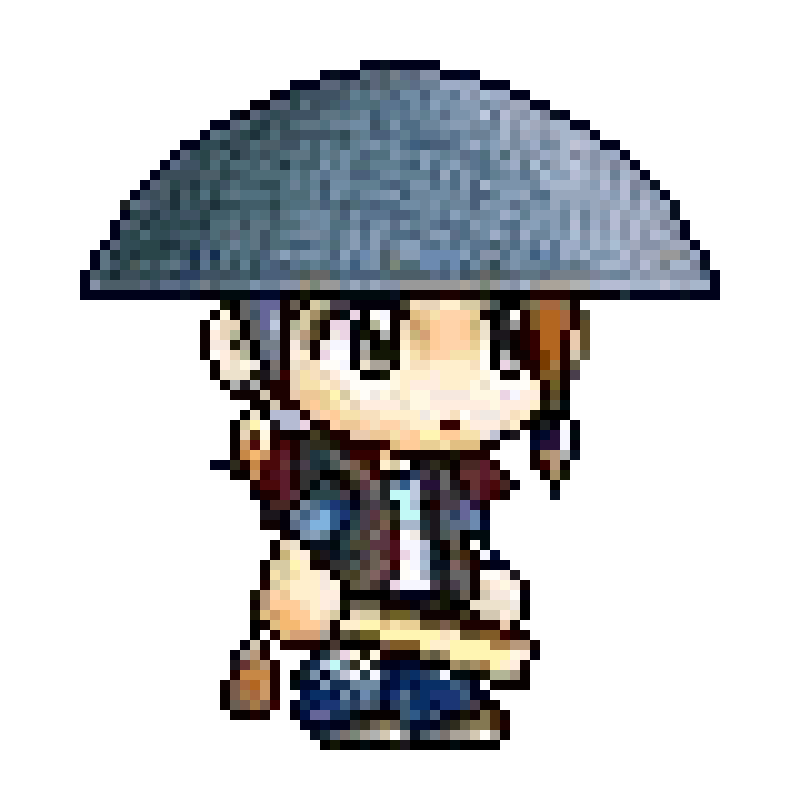
ASP.NET Entity Framework 获取 MySQL 数据库的所有表名:
using (var db = new dbEntities())
{
string sql = $"SELECT table_name FROM information_schema.tables WHERE table_schema = '{db.Database.Connection.Database}';";
var tables = db.Database.SqlQuery<string>(sql).ToList();
}
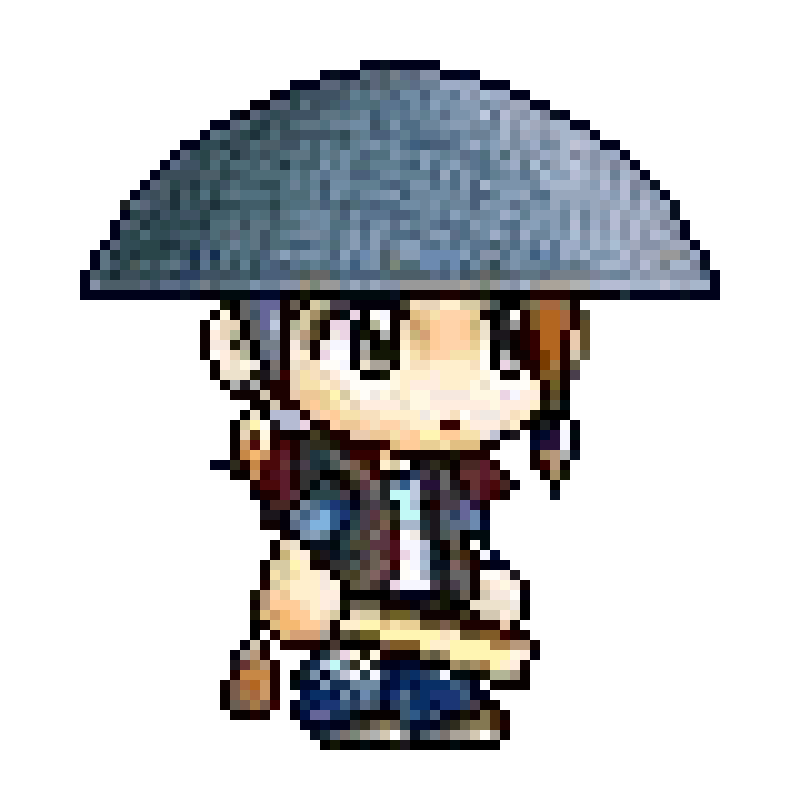
本文适用于 Window,CentOS(Linux) 系统请移步:http://xoyozo.net/Blog/Details/inotify-tools
FileSystemWatcher
关于监视文件系统事件的介绍
https://docs.microsoft.com/zh-cn/previous-versions/visualstudio/visual-studio-2008/ch2s8yd7(v=vs.90)
以控制台应用程序为例:
using System;
using System.IO;
using System.Security.Permissions;
public class Watcher
{
public static void Main()
{
Run();
}
[PermissionSet(SecurityAction.Demand, Name = "FullTrust")]
private static void Run()
{
string[] args = Environment.GetCommandLineArgs();
// If a directory is not specified, exit program.
if (args.Length != 2)
{
// Display the proper way to call the program.
Console.WriteLine("Usage: Watcher.exe (directory)");
return;
}
// Create a new FileSystemWatcher and set its properties.
using (FileSystemWatcher watcher = new FileSystemWatcher())
{
watcher.Path = args[1];
// Watch for changes in LastAccess and LastWrite times, and
// the renaming of files or directories.
watcher.NotifyFilter = NotifyFilters.LastAccess
| NotifyFilters.LastWrite
| NotifyFilters.FileName
| NotifyFilters.DirectoryName;
// Only watch text files.
watcher.Filter = "*.txt";
// Add event handlers.
watcher.Changed += OnChanged;
watcher.Created += OnChanged;
watcher.Deleted += OnChanged;
watcher.Renamed += OnRenamed;
// Begin watching.
watcher.EnableRaisingEvents = true;
// Wait for the user to quit the program.
Console.WriteLine("Press 'q' to quit the sample.");
while (Console.Read() != 'q') ;
}
}
// Define the event handlers.
private static void OnChanged(object source, FileSystemEventArgs e) =>
// Specify what is done when a file is changed, created, or deleted.
Console.WriteLine($"File: {e.FullPath} {e.ChangeType}");
private static void OnRenamed(object source, RenamedEventArgs e) =>
// Specify what is done when a file is renamed.
Console.WriteLine($"File: {e.OldFullPath} renamed to {e.FullPath}");
}
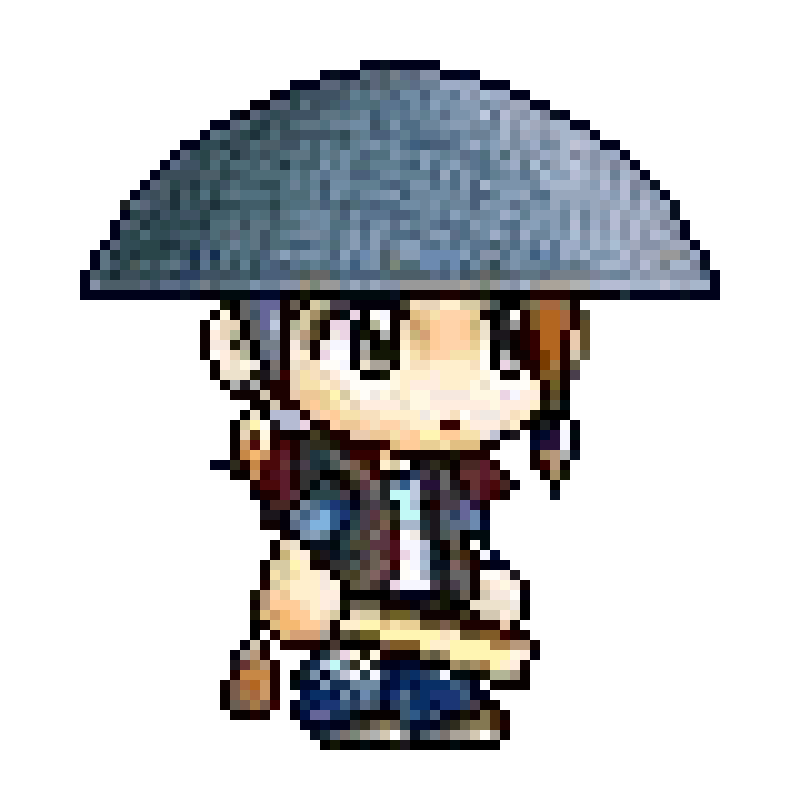
ASP.NET MVC 使用 Authorize 过滤器验证用户登录。Authorize 过滤器首先运行在任何其它过滤器或动作方法之前,主要用来做登录验证或者权限验证。
示例:使用 Authorize 过滤器实现简单的用户登录验证。
1、创建登录控制器 LoginController
/// <summary>
/// 登录控制器
/// </summary>
[AllowAnonymous]
public class LoginController : Controller
{
/// <summary>
/// 登录页面
/// </summary>
public ActionResult Index()
{
return View();
}
/// <summary>
/// 登录
/// </summary>
[HttpPost]
public ActionResult Login(string loginName, string loginPwd)
{
if (loginName == "admin" && loginPwd == "123456")
{
// 登录成功
Session["LoginName"] = loginName;
return RedirectToAction("Index", "Home");
}
else
{
// 登录失败
return RedirectToAction("Index", "Login");
}
}
/// <summary>
/// 注销
/// </summary>
public ActionResult Logout()
{
Session.Abandon();
return RedirectToAction("Index", "Login");
}
}
注意:在登录控制器 LoginController 上添加 AllowAnonymous 特性,该特性用于标记在授权期间要跳过 AuthorizeAttribute 的控制器和操作。
2、创建登录页面
@{
ViewBag.Title = "登录页面";
Layout = null;
}
<h2>登录页面</h2>
<form action='@Url.Action("Login","Login")' id="form1" method="post">
用户:<input type="text" name="loginName" /><br />
密码:<input type="password" name="loginPwd" /><br />
<input type="submit" value="登录">
</form>
效果图:
3、创建主页控制器 LoginController
public class HomeController : Controller
{
public ActionResult Index()
{
// 获取当前登录用户
string loginName = Session["LoginName"].ToString();
ViewBag.Message = "当前登录用户:" + loginName;
return View();
}
}
4、创建主页页面
@{
ViewBag.Title = "Index";
Layout = null;
}
<h2>Index</h2>
<h3>@ViewBag.Message</h3>
<a href="@Url.Action("Logout","Login")">注销</a>
效果图:
5、创建授权过滤器 LoginAuthorizeAttribute 类
创建 Filter 目录,在该目录下创建授权过滤器 LoginAuthorizeAttribute 类,继承 AuthorizeAttribute。
using System.Web.Mvc;
namespace MvcApp.Filter
{
/// <summary>
/// 授权过滤器
/// </summary>
public class LoginAuthorizeAttribute : AuthorizeAttribute
{
public override void OnAuthorization(AuthorizationContext filterContext)
{
// 判断是否跳过授权过滤器
if (filterContext.ActionDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true)
|| filterContext.ActionDescriptor.ControllerDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true))
{
return;
}
// 判断登录情况
if (filterContext.HttpContext.Session["LoginName"] == null || filterContext.HttpContext.Session["LoginName"].ToString()=="")
{
//HttpContext.Current.Response.Write("认证不通过");
//HttpContext.Current.Response.End();
filterContext.Result = new RedirectResult("/Login/Index");
}
}
}
}
通常 Authorize 过滤器也是在全局过滤器上面的,在 App_Start 目录下的 FilterConfig 类的 RegisterGlobalFilters 方法中添加:
using System.Web;
using System.Web.Mvc;
using MvcApp.Filter;
namespace MvcApp
{
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
// 添加全局授权过滤器
filters.Add(new LoginAuthorizeAttribute());
}
}
}
Global.asax 下的代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Optimization;
using System.Web.Routing;
namespace MvcApp
{
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
}
}
}
xoyozo:暂时没有按区域(Area)来统一配置的方法,建议加在 Controller 上。https://stackoverflow.com/questions/2319157/how-can-we-set-authorization-for-a-whole-area-in-asp-net-mvc
在数据库连接字符串可设置是否将 tinyint(1) 映射为 bool,否则为 sbyte:
TreatTinyAsBoolean=false/true
tinyint(1) 一般用于表示 bool 型字段,存储内容为 0 或 1,但有时候也用来存储其它数字。
以 Discuz! 的表 pre_forum_post 为例,字段 first 和 invisible 都是 tinyint(1),但 first 只储存 0 和 1,invisible 却有 -1、-5 之类的值。
因此我们一般设置 TreatTinyAsBoolean=false,程序中 first 与 invisible 均以 sbyte 处理。
设置 TreatTinyAsBoolean=true 后,EF 或 EF Core 自动将该类型映射为 bool,方便在程序中作进一步处理。
一旦设置 TreatTinyAsBoolean=true,那么所有查询结果中 tinyint(1) 字段的返回值永远只有 1 和 0(即 True/False),即使真实值为 -1,也返回 1。
因为我们必须在确保所有的 tinyint(1) 类型字段都仅表示布尔值是才设置 TreatTinyAsBoolean=true。
一旦部分 tinyint(1) 类型字段用于存放 0 和 1 以外的数,那么就应该设置 TreatTinyAsBoolean=false。
在数据库优先的项目中,以 TreatTinyAsBoolean=false 生成数据模型后,可将明确为布尔类型的字段改为 bool。列出 MySQL 数据库中所有表所有字段中类型为 tinyint(1) 的字段值
以 .edmx 为例:
在项目中搜索该字段名,在搜索结果中找到 .edmx 文件中的两处。
.edmx 文件中的注释已经表明其包含 SSDL、CSDL、C-S mapping 三块内容,
在 SSDL content 下方找到该字段:
<Property Name="字段名" Type="tinyint" Nullable="***" />
改为
<Property Name="字段名" Type="bool" Nullable="***" />
在 CSDL content 下方找到该属性:
<Property Name="属性名" Type="SByte" Nullable="***" />
改为
<Property Name="属性名" Type="Boolean" Nullable="***" />
在解决方案管理器中展开 .edmx ->库名.tt -> 表名.cs 文件,
将模型类中的属性
public sbyte invisible { get; set; }
改为
public bool invisible { get; set; }
或 sbyte? 改为 bool?。
在 EF Core 中,直接打开对应数据表的 .cs 文件,更改属性类型即可。
相关报错:
错误: 指定的成员映射无效。类型中的成员的类型“Edm.SByte[Nullable=False,DefaultValue=]”与类型中的成员的“MySql.bool[Nullable=False,DefaultValue=]”不兼容。
InvalidOperationException: The property '***' is of type 'sbyte' which is not supported by the current database provider. Either change the property CLR type, or ignore the property using the '[NotMapped]' attribute or by using 'EntityTypeBuilder.Ignore' in 'OnModelCreating'.
尝试先连接一次能解决此问题(概率),非常的莫名其妙:
using Data.Discuz.db_bbs2021Context dbd = new();
var conn = dbd.Database.GetDbConnection();
conn.Open();
conn.Close();
参考:
https://mysqlconnector.net/connection-options/
https://stackoverflow.com/questions/6656511/treat-tiny-as-boolean-and-entity-framework-4
2023年1月注:本文适用于 Pomelo.EntityFrameworkCore.MySql 6.0,升级到 7.0 后会出现:
System.InvalidOperationException:“The 'sbyte' property could not be mapped to the database type 'tinyint(1)' because the database provider does not support mapping 'sbyte' properties to 'tinyint(1)' columns. Consider mapping to a different database type or converting the property value to a type supported by the database using a value converter. See https://aka.ms/efcore-docs-value-converters for more information. Alternately, exclude the property from the model using the '[NotMapped]' attribute or by using 'EntityTypeBuilder.Ignore' in 'OnModelCreating'.”
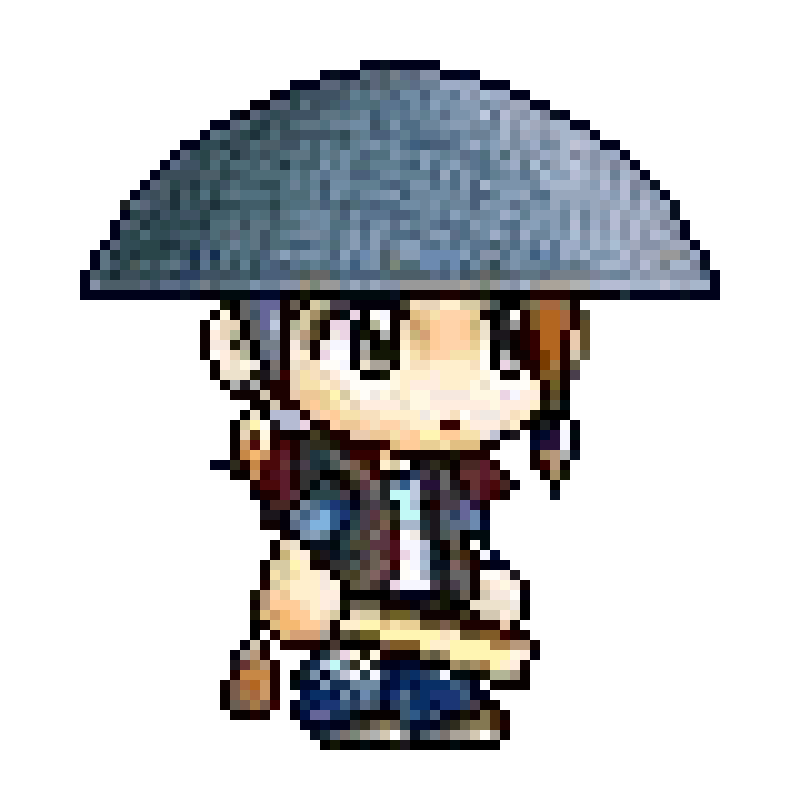
Nuget 安装:X.PagedList.Mvc.Core
控制器:
using X.PagedList;
public IActionResult Index(int page = 1)
{
……
return View(q.ToPagedList(page, size));
}
视图:
@using X.PagedList
@using X.PagedList.Mvc.Core
@model IPagedList<xxx>
@Html.PagedListPager(Model, page => Url.Action("Index", new { page }))
自定义(options 默认值):
@Html.PagedListPager(
Model,
page => Url.Action("Index", new { page }),
new X.PagedList.Mvc.Common.PagedListRenderOptionsBase
{
HtmlEncoder = HtmlEncoder.get_Default(),
DisplayLinkToFirstPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToLastPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToPreviousPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToNextPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToIndividualPages = true,
DisplayPageCountAndCurrentLocation = false, // 显示总页数和当前页码
MaximumPageNumbersToDisplay = 10, // 最多显示页码数
DisplayEllipsesWhenNotShowingAllPageNumbers = true,
EllipsesFormat = "…",
LinkToFirstPageFormat = "<<",
LinkToPreviousPageFormat = "<",
LinkToIndividualPageFormat = "{0}",
LinkToNextPageFormat = ">",
LinkToLastPageFormat = ">>",
PageCountAndCurrentLocationFormat = "Page {0} of {1}.",
ItemSliceAndTotalFormat = "Showing items {0} through {1} of {2}.",
FunctionToDisplayEachPageNumber = null,
ClassToApplyToFirstListItemInPager = null,
ClassToApplyToLastListItemInPager = null,
ContainerDivClasses = new string[1]
{
"pagination-container"
},
UlElementClasses = new string[1]
{
"pagination"
},
LiElementClasses = Enumerable.Empty<string>(),
PageClasses = Enumerable.Empty<string>(),
UlElementattributes = null,
ActiveLiElementClass = "active",
EllipsesElementClass = "PagedList-ellipses",
PreviousElementClass = "PagedList-skipToPrevious",
NextElementClass = "PagedList-skipToNext",
})
保留地址栏参数:
@{
string query = Context.Request.QueryString.Value;
}
@Html.PagedListPager(Model, page => Regex.IsMatch(query, @"[?&]page=\d+")
? Regex.Replace(query, @"([?&])page=\d+", $"$1page={page}")
: (query.StartsWith("?") ? $"{query}&page={page}" : $"{query}?page={page}"),
new X.PagedList.Web.Common.PagedListRenderOptionsBase
{
DisplayPageCountAndCurrentLocation = true,
MaximumPageNumbersToDisplay = 5,
})
这里从查询字符串中判断并替换 page 值,如果有更简单的方法敬请告知。比如 Webdiyer 的分页组件会自动携带所有参数。
更多使用方式参官方文档:https://github.com/dncuug/X.PagedList
附应用于 Unify Template(一款基于 Bootstrap 的 HTML 模板)中的配置:
<style>
.u-pagination-v1-1--active .u-pagination-v1-1 { color: #fff; border-color: #72c02c; }
.PagedList-pageCountAndLocation { float: right !important; }
</style>
@{
string query = Context.Request.QueryString.Value;
}
@Html.PagedListPager(Model, page => Regex.IsMatch(query, @"[?&]page=\d+")
? Regex.Replace(query, @"([?&])page=\d+", $"$1page={page}")
: (query.StartsWith("?") ? $"{query}&page={page}" : $"{query}?page={page}"),
new X.PagedList.Web.Common.PagedListRenderOptionsBase
{
DisplayPageCountAndCurrentLocation = true,
MaximumPageNumbersToDisplay = 5,
UlElementClasses = new string[] { "list-inline" },
LiElementClasses = new string[] { "list-inline-item" },
PageClasses = new string[] { "u-pagination-v1__item", "u-pagination-v1-1", "g-pa-7-14" },
ActiveLiElementClass = "u-pagination-v1-1--active",
EllipsesElementClass = "g-pa-7-14",
})
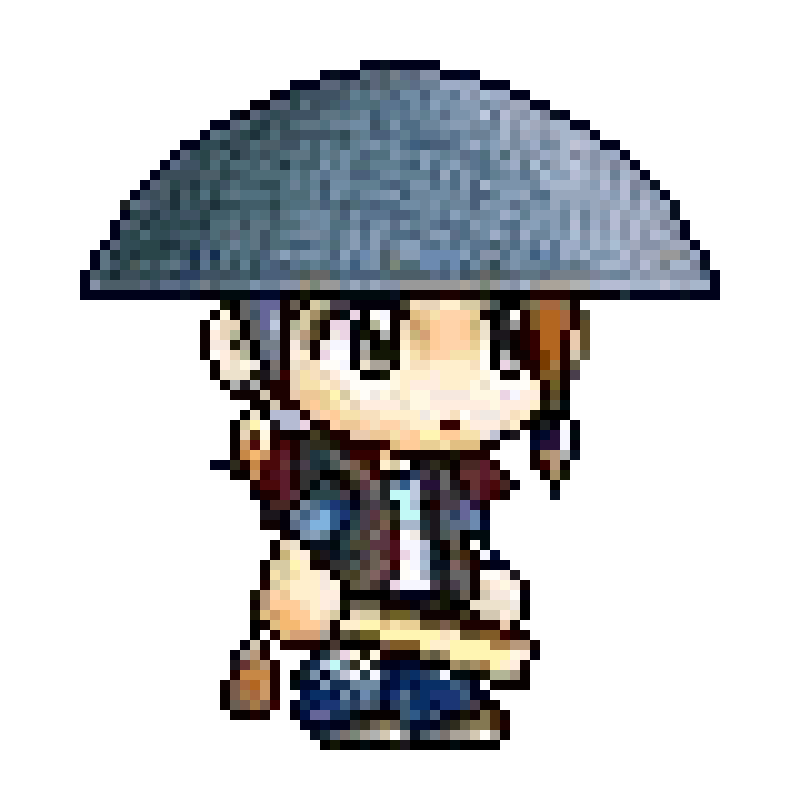
打开 appsettings.json,添加一项配置(如下方示例中的“SiteOptions”项)
* 注意,如需配置开发环境与生产环境不同的值,可单独在 appsettings.Development.json 文件中配置不同项,格式层次须一致;
C# 中习惯用强类型的方式来操作对象,那么在项目根目录添加类(类名以 SiteOptions为例),格式与 appsettings.json 中保持一致:
public class SiteOptions
{
public ERPOptions ERP { get; set; }
public WeixinOpenOptions WeixinOpen { get; set; }
public WeixinMPOptions WeixinMP { get; set; }
public SMSOptions SMS { get; set; }
public AliyunOSSOptions AliyunOSS { get; set; }
/// <summary>
/// 单个文件上传的最大大小(MB)
/// </summary>
public int MaxSizeOfSigleFile_MB { get; set; }
/// <summary>
/// 单个文件上传的最大大小(字节)
/// </summary>
public int MaxSizeOfSigleFile_B => MaxSizeOfSigleFile_MB * 1024 * 1024;
public class ERPOptions
{
public int ChannelId { get; set; }
public string AppKey { get; set; }
}
public class WeixinOpenOptions
{
public string AppId { get; set; }
public string AppSecret { get; set; }
}
public class WeixinMPOptions
{
public string AppId { get; set; }
public string AppSecret { get; set; }
}
public class SMSOptions
{
public string AppKey { get; set; }
}
public class AliyunOSSOptions
{
public string Endpoint { get; set; }
public string AccessKeyId { get; set; }
public string AccessKeySecret { get; set; }
public string BucketName { get; set; }
/// <summary>
/// 格式://域名/
/// </summary>
public string CdnUrl { get; set; }
}
}
在 Startup 中注入 IConfiguration,并在 ConfigureServices() 方法中添加服务(注意使用 GetSection() 映射到自命名的“SiteOptions”项)
在控制器中使用:
在控制器类中键入“ctor”,并按两次 Tab 键,创建构造函数
在构造函数中注入“IOptions”,并在 Action 中使用
using Microsoft.Extensions.Options;
public class TestController : Controller
{
private readonly IOptions<SiteOptions> options;
public TestController(IOptions<SiteOptions> options)
{
this.options = options;
}
public IActionResult Index()
{
return View(options.Value.ERP.ChannelId.ToString());
}
}
在视图中使用:
@using Microsoft.Extensions.Options
@inject IOptions<SiteOptions> options
@options.Value.ERP.ChannelId
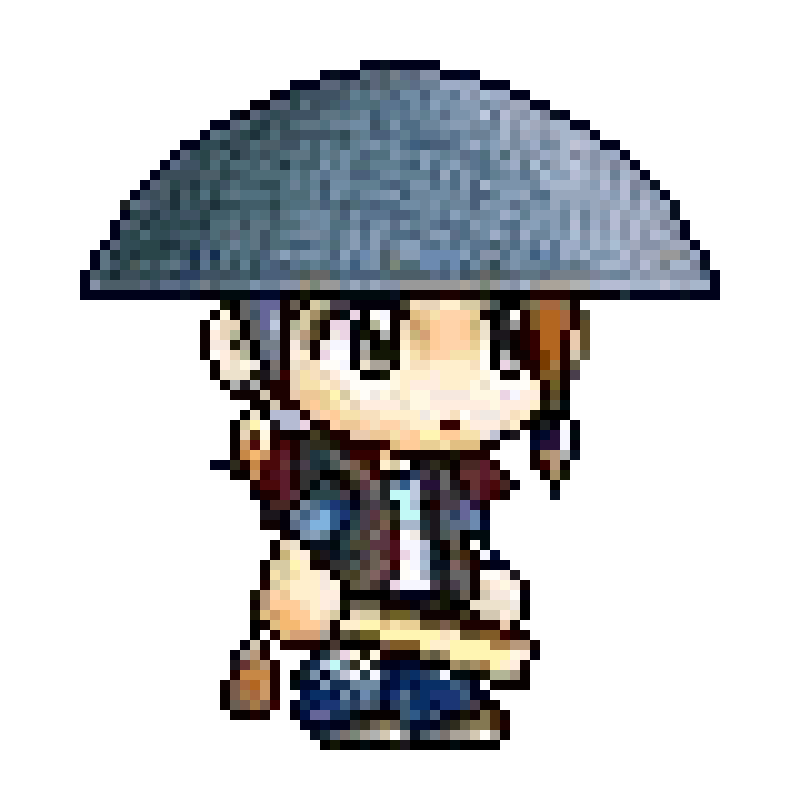
* 本文凡使用变量 httpContextAccessor 或 _httpContextAccessor 的地方都需要需要注入 Microsoft.AspNetCore.Http.IHttpContextAccessor
* 在视图(view)中应以 ViewContext 类引出
获取当前页面网址:
using Microsoft.AspNetCore.Http.Extensions;
string url = Request.GetDisplayUrl();
获取当前访问的域名和端口:
获取来源:
Request.GetTypedHeaders().Referer
判断 Scheme 是否为 https:
Request.IsHttps
获取客户端IP和端口、服务器IP和端口:
获取浏览器用户代理(UserAgent):
httpContextAccessor.HttpContext.Request.Headers[HeaderNames.UserAgent];
获取当前请求的唯一标识:
httpContextAccessor.HttpContext.TraceIdentifier
返回结果:80000564-0002-f700-b63f-84710c7967bb
用途:可作为生成随机数的种子。
获取客户端IP地址:
httpContextAccessor.HttpContext.Connection.RemoteIpAddress
获取当前项目根目录磁盘路径:
AppContext.BaseDirectory // 以“\”结尾
注意:此路径到项目根目录,而非网站根目录,网站根目录请自行追加,默认为 wwwroot\
编码/解码:(参数和返回值均为 string?)
using System.Net;
WebUtility.HtmlEncode(myString)
WebUtility.HtmlDecode(myString)
WebUtility.UrlEncode(myString)
WebUtility.UrlDecode(myString)
更多:https://xoyozo.net/Blog/Index/Core
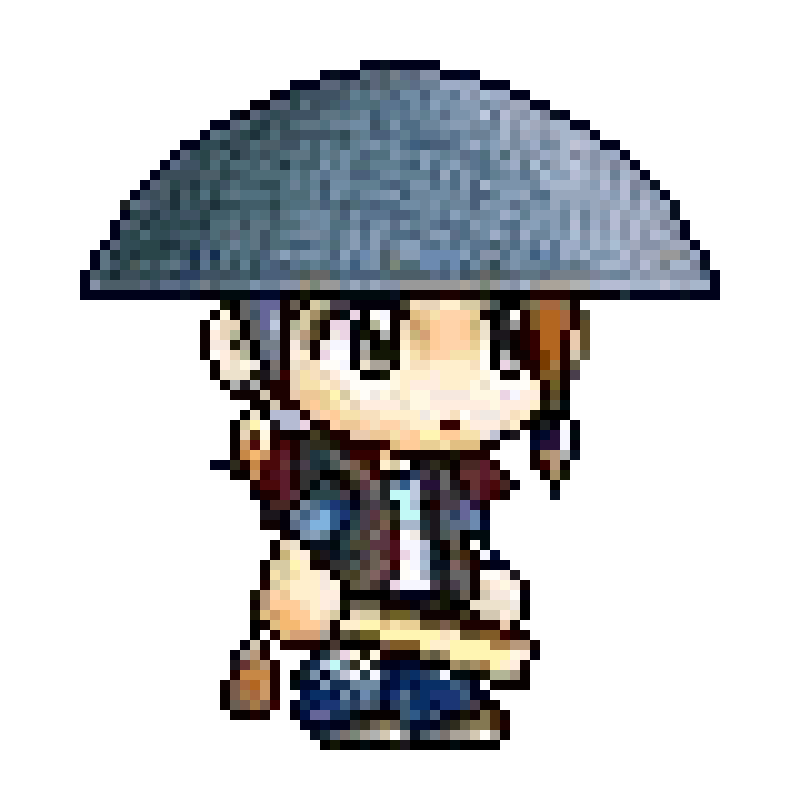