POST
$.post('AjaxCases', {
following: true,
success: null,
page: 1,
}, function (response) {
console.log(response.data)
}, 'json').fail(function (xhr, status, error) {
console.log(xhr.responseText);
});
[HttpPost]
public IActionResult AjaxCases([FromForm] AjaxCasesRequestModel req)
{
bool? following = req.following;
bool? success = req.success;
int page = req.page;
}
提示:只能传输对象(Object),不能传输数组(Array)。
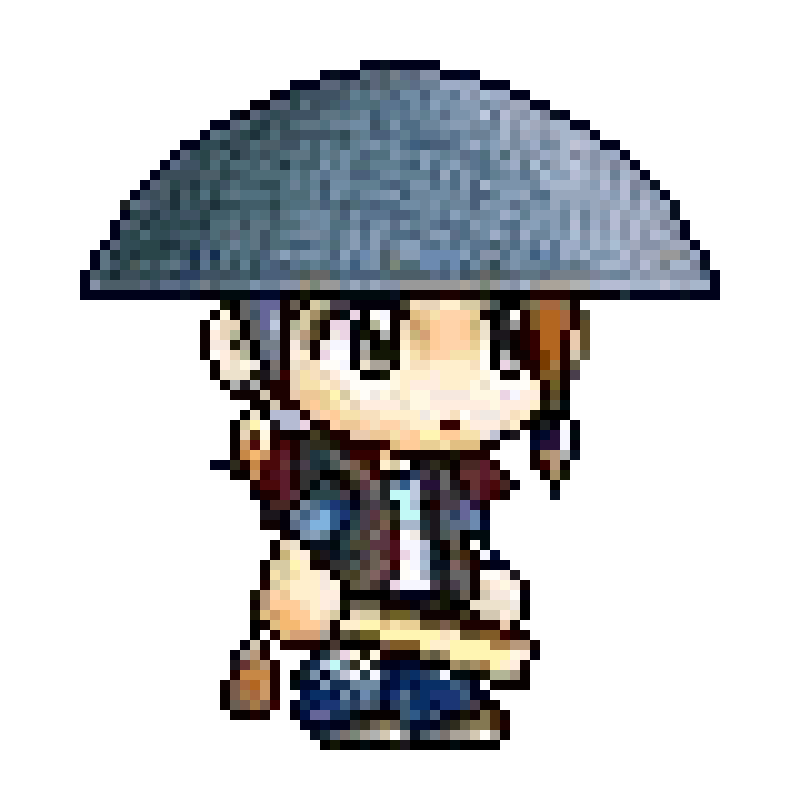
以下是使用定时器每秒钟请求服务器,并在后一个请求的响应比前一个请求的响应先到达客户端时抛弃前一个请求的响应的代码:
(以 $.post 为例,$.ajax 同理)
let previousXHR = null; // 存储前一个请求的 jqXHR 对象
function sendRequest() {
// 取消前一个请求
if (previousXHR) {
previousXHR.abort();
}
// 发送新的请求
const currentXHR = $.post(url, data, function(response) {
// 请求成功的回调函数
console.log(response);
});
// 更新前一个请求的 jqXHR 对象
previousXHR = currentXHR;
}
// 每秒钟发送请求
setInterval(sendRequest, 1000);
如果你用 axios,请参此文。
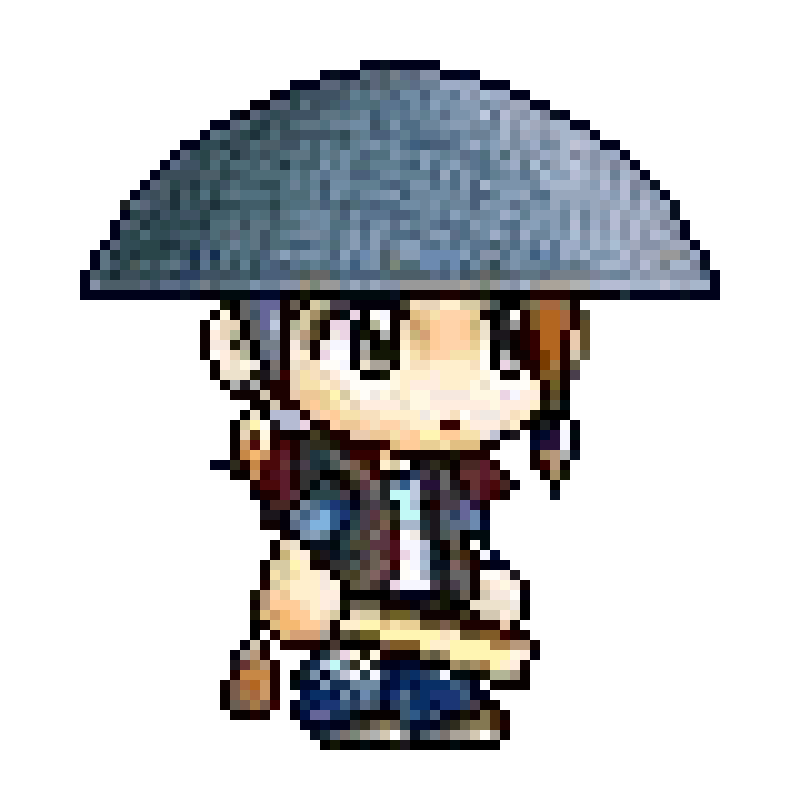
不知道从哪个版本的 Chrome 或 Edge 开始,我们无法通过 ctrl+v 快捷键将时间格式的字符串粘贴到 type 为 date 的 input 框中,我们想办法用 JS 来实现。
方式一、监听 paste 事件:
const input = document.querySelector('input[type="date"]');
input.addEventListener('paste', (event) => {
input.value = event.clipboardData.getData('text');
});
这段代码实现了从页面获取这个 input 元素,监听它的 paste 事件,然后将粘贴板的文本内容赋值给 input。
经测试,当焦点在“年”的位置时可以粘贴成功,但焦点在“月”或“日”上不会触发 paste 事件。
方式二、监听 keydown 事件:
const input = document.querySelector('input[type="date"]');
input.addEventListener('keydown', (event) => {
if ((navigator.platform.match("Mac") ? event.metaKey : event.ctrlKey) && event.key === 'v') {
event.preventDefault();
var clipboardData = (event.clipboardData || event.originalEvent.clipboardData);
input.value = clipboardData.getData('text');
}
});
测试发现报错误:
Uncaught TypeError: Cannot read properties of undefined (reading 'getData')
Uncaught TypeError: Cannot read properties of undefined (reading 'clipboardData')
看来 event 中没有 clipboardData 对象,改为从 window.navigator 获取:
const input = document.querySelector('input[type="date"]');
input.addEventListener('keydown', (event) => {
if ((navigator.platform.match("Mac") ? event.metaKey : event.ctrlKey) && event.key === 'v') {
event.preventDefault();
window.navigator.clipboard.readText().then(text => {
input.value = text;
});
}
});
缺点是需要用户授权:
仅第一次需要授权,如果用户拒绝,那么以后就默认拒绝了。
以上两种方式各有优缺点,选择一种适合你的方案就行。接下来继续完善。
兼容更多时间格式,并调整时区
<input type="date" /> 默认的日期格式是 yyyy-MM-dd,如果要兼容 yyyy-M-d 等格式,那么:
const parsedDate = new Date(text);
if (!isNaN(parsedDate.getTime())) {
input.value = parsedDate.toLocaleDateString('en-GB', { year: 'numeric', month: '2-digit', day: '2-digit' }).split('/').reverse().join('-');
}
以 text 为“2023-4-20”举例,先转为 Date,如果成功,再转为英国时间格式“20-04-2023”,以“/”分隔,逆序,再以“-”连接,就变成了“2023-04-20”。
当然如果希望支持中文的年月日,可以先用正则表达式替换一下:
text = text.replace(/\s*(\d{4})\s*年\s*(\d{1,2})\s*月\s*(\d{1,2})\s*日\s*/, "$1-$2-$3");
处理页面上的所有 <input type="date" />
const inputs = document.querySelectorAll('input[type="date"]');
inputs.forEach((input) => {
input.addEventListener(...);
});
封装为独立域
避免全局变量污染,使用 IIFE 函数表达式:
(function() {
// 将代码放在这里
})();
或者封装为函数,在 jQuery 的 ready 中,或 Vue 的 mounted 中调用。
在 Vue 中使用
如果将粘贴板的值直接赋值到 input.value,在 Vue 中是不能同步更新 v-model 绑定的变量的,所以需要直接赋值给变量:
<div id="app">
<input type="date" v-model="a" data-model="a" v-on:paste="fn_pasteToDateInput" />
{{a}}
</div>
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
<script>
const app = Vue.createApp({
data: function () {
return {
a: null,
}
},
methods: {
fn_pasteToDateInput: function (event) {
const text = event.clipboardData.getData('text');
const parsedDate = new Date(text);
if (!isNaN(parsedDate.getTime())) {
const att = event.target.getAttribute('data-model');
this[att] = parsedDate.toLocaleDateString('en-GB', { year: 'numeric', month: '2-digit', day: '2-digit' }).split('/').reverse().join('-');
}
},
}
});
const vm = app.mount('#app');
</script>
示例中 <input /> 添加了 data- 属性,值同 v-model,并使用 getAttribute() 获取,利用 this 对象的属性名赋值。
如果你的 a 中还有嵌套对象 b,那么 data- 属性填写 a.b,方法中以“.”分割逐级查找对象并赋值
let atts = att.split('.');
let target = this;
for (let i = 0; i < atts.length - 1; i++) {
target = target[atts[i]];
}
this.$set(target, atts[atts.length - 1], text);
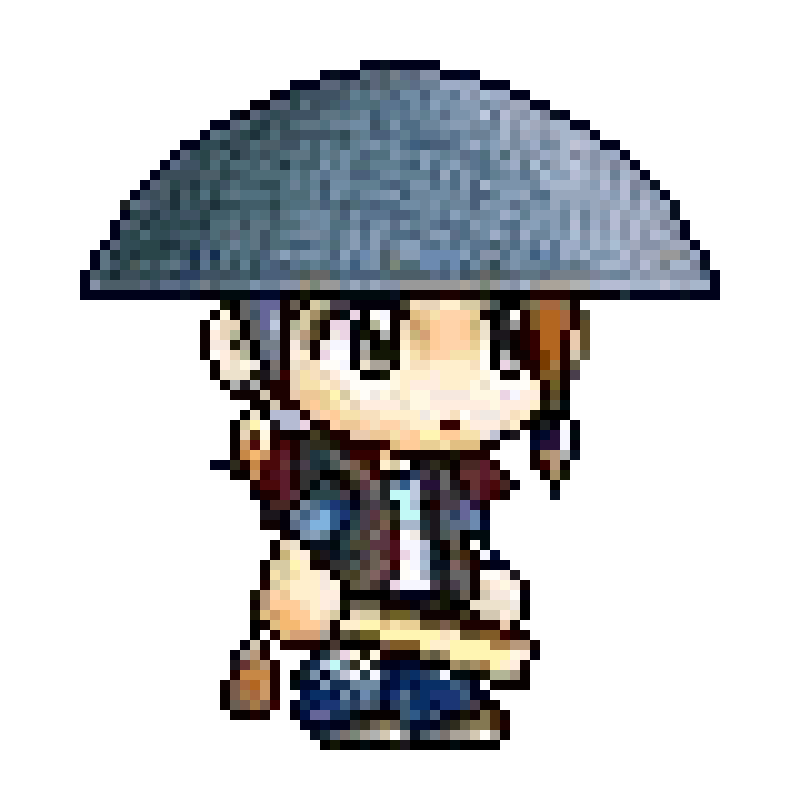
一般我们使用原生 JS 的时候使用 abort() 来取消 fetch 请求。
在使用 axios 发送请求时,如果我们想要取消请求,可以使用 axios 提供的 CancelToken 和 cancel 方法。下面是具体的实现步骤:
// 创建 CacnelToken 实例
const cancelTokenSource = axios.CancelToken.source();
// GET 方式请求
axios.get(url, {
cancelToken: cancelTokenSource.token
}).catch(thrown => {
if (axios.isCancel(thrown)) {
console.log('Request canceled', thrown.message);
} else {
console.log('An error occurred', thrown);
}
});
// POST 方式请求
axios.post(url, data, {
cancelToken: cancelTokenSource.token
});
// 取消请求
cancelTokenSource.cancel('请求被取消');
get 请求的时候,cancelToken 是放在第二个参数里;post 的时候,cancelToken 是放在第三个参数里。axios-0.27.2 中测试成功。
在即时响应的搜索框中可以这样处理:(vue3)
let cancelTokenSource;
const app = Vue.createApp({
methods: {
fn_list: function () {
// 如果已有请求则取消
cancelTokenSource && cancelTokenSource.cancel();
// 创建一个新的请求
cancelTokenSource = axios.CancelToken.source();
axios.post(url, data, {
cancelToken: cancelTokenSource.token
}).then(function (response) {
// 请求成功
}).catch(function (error) {
// 请求失败/取消
});
},
}
});
const vm = app.mount('#app');
如果你用 jQuery,请参此文。
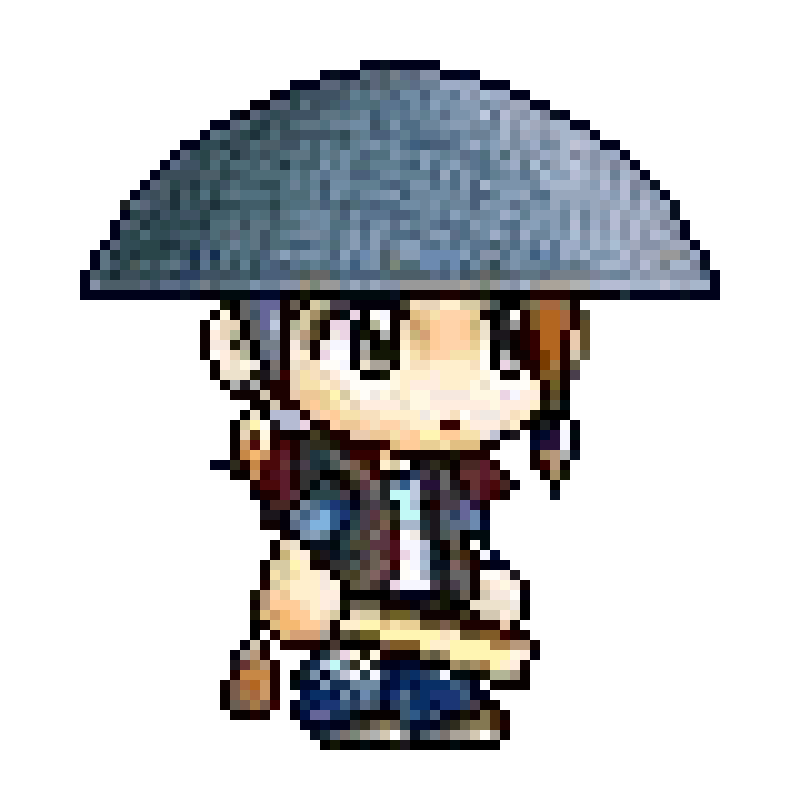
以 ASP.NET 6 返回 BadRequestObjectResult 为例。
public IActionResult Post()
{
return BadRequest("友好的错误提示语");
}
axios
请求示例:
axios.post(url, {})
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.log(error.response.data);
});
error 对象:
{
"message": "Request failed with status code 400",
"name": "AxiosError",
"code": "ERR_BAD_REQUEST",
"status": 400,
"response": {
"data": "友好的错误提示语",
"status": 400
}
}
jQuery
请求示例:
$.ajax 对象形式
$.ajax({
url: url,
type: 'POST',
data: {},
success: function (data) {
console.log(data)
},
error: function (jqXHR) {
console.log(jqXHR.responseText)
},
complete: function () {
},
});
$.ajax 事件处理形式(Event Handlers)jQuery 3+
$.ajax({
url: url,
type: 'POST',
data: {},
})
.done(function (data) {
console.log(data)
})
.fail(function (jqXHR) {
console.log(jqXHR.responseText)
})
.always(function () {
});
$.get / $.post / $.delete
$.post(url, {}, function (data) {
console.log(data)
})
.done(function (data) {
console.log(data)
})
.fail(function (jqXHR) {
console.log(jqXHR.responseText)
})
.always(function () {
});
jqXHR 对象:
{
"readyState": 4,
"responseText": "友好的错误提示语",
"status": 400,
"statusText": "error"
}
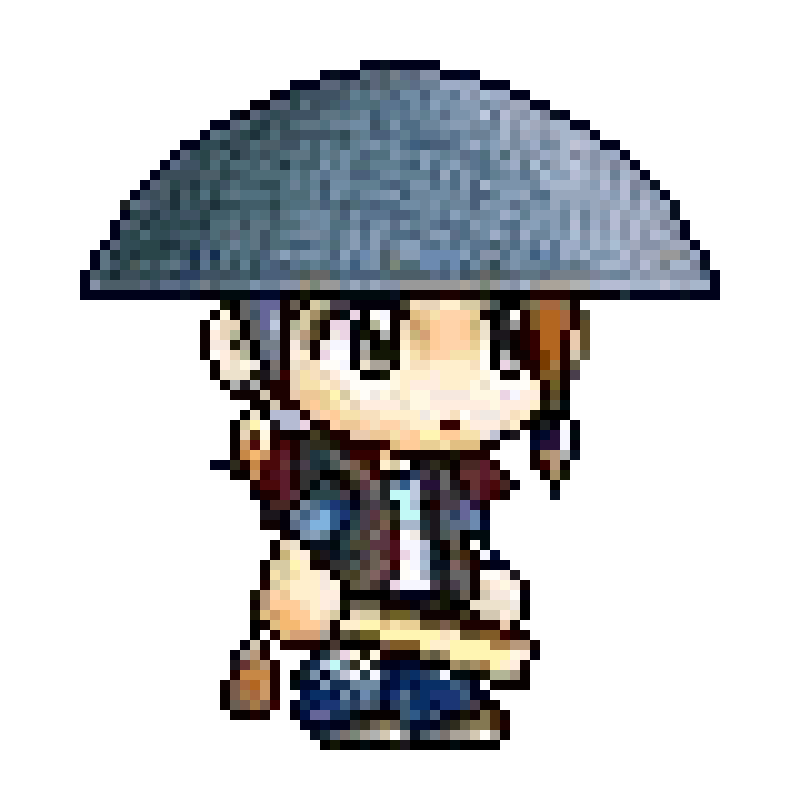
原生判断
window.addEventListener('scroll', scroll)
function scroll() {
const scrollTop = document.documentElement.scrollTop || document.body.scrollTop;
const clientHeight = document.documentElement.clientHeight || document.body.clientHeight;
const scrollHeight = document.documentElement.scrollHeight || document.body.scrollHeight;
if (scrollTop === 0) {
console.log('滚动到顶了');
}
if (scrollTop + clientHeight >= scrollHeight - 100) {
console.log('滚动到底了');
}
}
window.removeEventListener('scroll', scroll) // 移除滚动事件
scrollTop:文档在可视区上沿的位置距离文档顶部的距离
clientHeight:可视区的高度
scrollHeight:文档总高度
100:用于在滚动到底部之前提前加载内容(如瀑布流),提高用户体验,请自行修改数值(建议不小于 2 像素,以规避在部分浏览器上因获得值为小数时可能无法触发的问题)
jQuery 判断
window.addEventListener('scroll', scroll)
function scroll() {
const doc_height = $(document).height();
const scroll_top = $(document).scrollTop();
const window_height = $(window).height();
if (scroll_top === 0) {
console.log('滚动到顶了');
} else if (scroll_top + window_height >= doc_height - 100) {
console.log('滚动到底了');
}
}
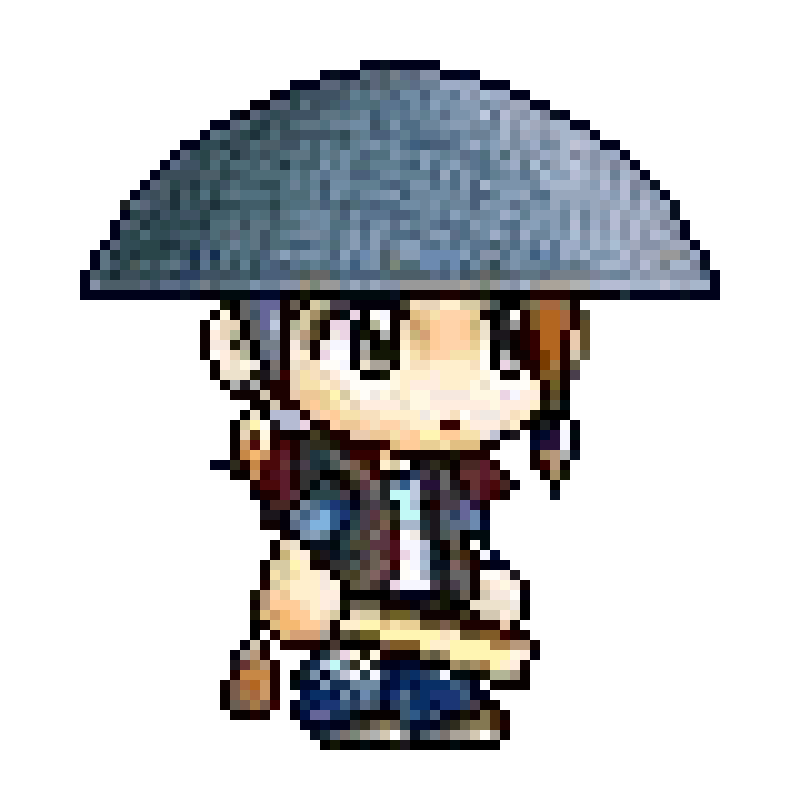
POST
axios.post('AjaxCases', {
following: true,
success: null,
page: 1,
}).then(function (response) {
console.log(response.data)
}).catch(function (error) {
console.log(error.response.data);
});
[HttpPost]
public IActionResult AjaxCases([FromBody] AjaxCasesRequestModel req)
{
bool? following = req.following;
bool? success = req.success;
int page = req.page;
}
GET
axios.get('AjaxCase', {
params: {
int: 123,
}
}).then(function (response) {
console.log(response.data)
}).catch(function (error) {
console.log(error.response.data);
});
[HttpGet]
public IActionResult AjaxCases([FromQuery] int id)
{
}
DELETE
格式与 GET 类似
* 若服务端获取参数值为 null,可能的情况如下:
请检查相关枚举类型是否已设置 [JsonConverter] 属性,参:C# 枚举(enum)用法笔记,且传入的值不能为空字符串,可以传入 null 值,并将服务端 enum 类型改为可空。可以以 string 方式接收参数后再进行转换。
模型中属性名称不能重复(大小写不同也不行)。
布尔型属性值必须传递布尔型值,即在 JS 中,字符串 "true" 应 JSON.parse("true") 为 true 后再回传给服务端。
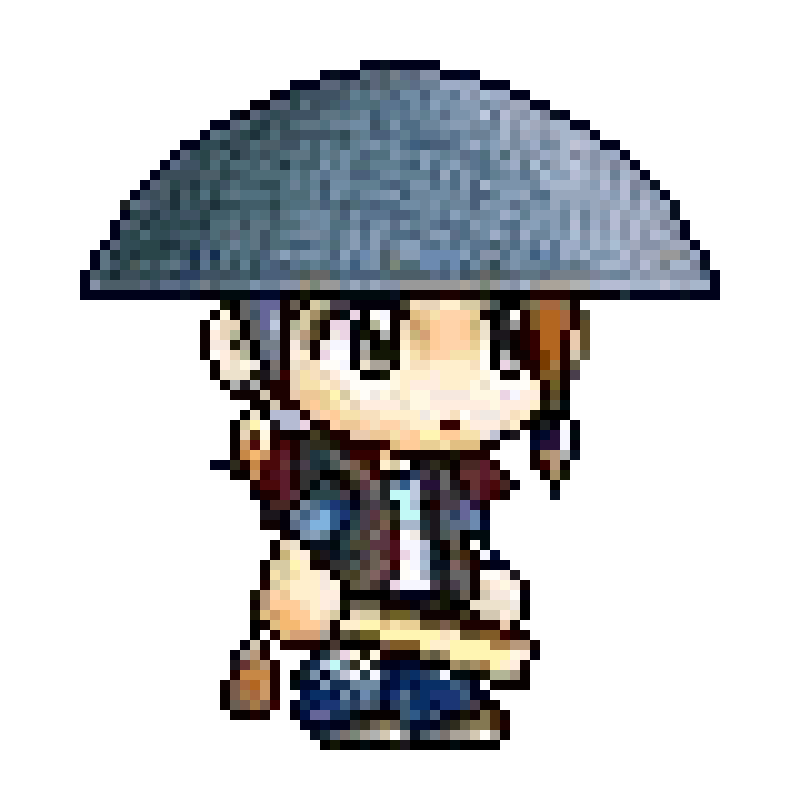
iPhone 或安卓上传的照片,可能因横向或纵向拍摄,导致上传后照片显示成 90 度、180 度、270 度角旋转,使用 C# 处理该照片时同样会遇到相同的问题。
解决的方案:
客户端使用 base64 显示图片,可按正常方向显示,且不通过服务端即时显示。关键代码:
$('#file_input').change(function (e) {
if (e.target.files.length == 0) { return; }
// file 转换为 base64
var reader = new FileReader();
reader.readAsDataURL(e.target.files[0]);
reader.onload = function (event) {
$('#myImg').attr('src', event.target.result).css({ width: 'auto', height: 'auto' });
}
});
$('#myImg').on('load', function () {
console.log('图片加载完成')
});
但 base64 字符串传递到服务端受请求大小限制(一般比直接 POST 文件要小)。所以显示图片的同时应即时使用 POST 方式上传图片(如 jquery.fileupload.js)。
当然如果是在微信中打开网页,使用 JS-SDK 的 wx.chooseImage() 返回的 localId 也可以在 <img /> 中正常显示,然后使用 wx.uploadImage() 上传到微信服务器并获取 serverId,在通过获取临时素材接口取回图片文件。
服务端如果需要处理该图片(缩放、裁切、旋转等),需要先读取照片的拍摄角度,旋正后,再进行处理,相关 C# 代码:
/// <summary>
/// 调整照片的原始拍摄旋转角度
/// </summary>
/// <param name="img"></param>
/// <returns></returns>
private Image ImageRotateFlip(Image img)
{
foreach (var prop in img.PropertyItems)
{
if (prop.Id == 0x112)
{
switch (prop.Value[0])
{
case 6: img.RotateFlip(RotateFlipType.Rotate90FlipNone); break;
case 3: img.RotateFlip(RotateFlipType.Rotate180FlipNone); break;
case 8: img.RotateFlip(RotateFlipType.Rotate270FlipNone); break;
}
prop.Value[0] = 1;
}
}
return img;
}
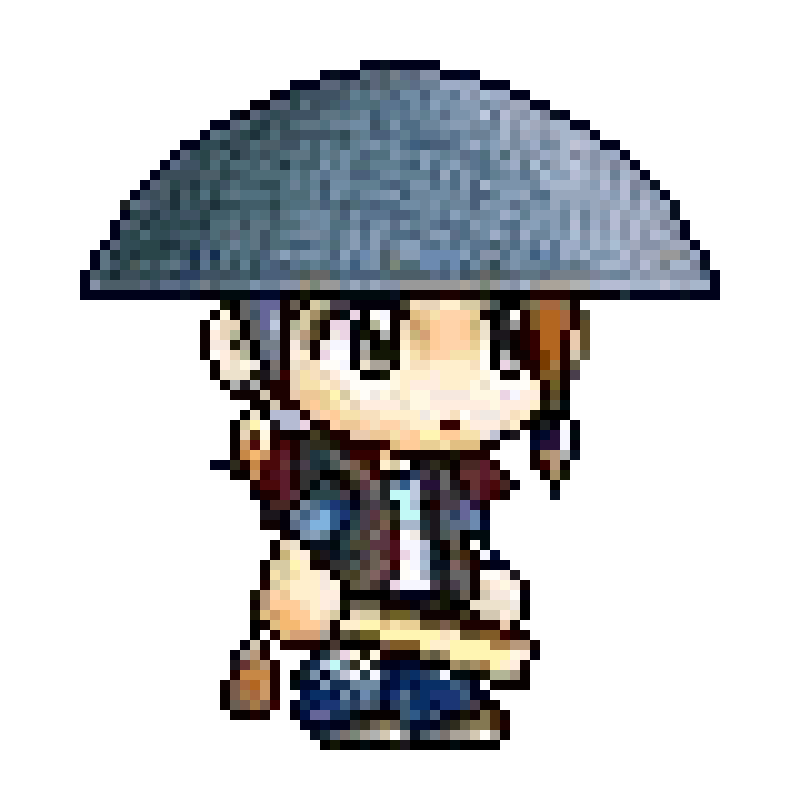
前端引用 jquery、jquery-ui(或 jquery.ui.widget),以及 jquery.fileupload.js
$('#xxx').fileupload({
url: 'FileUpload',
add: function (e, data) {
console.log('add', data);
data.submit();
},
success: function (response, status) {
console.log('success', response);
toastr.success('上传成功!文件名:' + response);
},
error: function (error) {
console.log('error', error);
toastr.error("上传失败:" + error.responseText);
}
});
服务端接收文件:
[HttpPost]
public ActionResult FileUpload([FromForm(Name = "files[]")] IFormFile file)
{
try
{
return Ok(file.FileName);
}
catch(Exception ex)
{
return BadRequest(ex.Message);
}
}
此处 Name = "files[]" 值是由 <input type="file" name="这里定的" />
如果 input 没有指定 name,那么一般为 file[],或者在 Chrome 的开发者工具中,切换到 Network 标签页,选中 post 的目标请求,在 Headers 的 Form Data 中可以看到 name="xxx"。
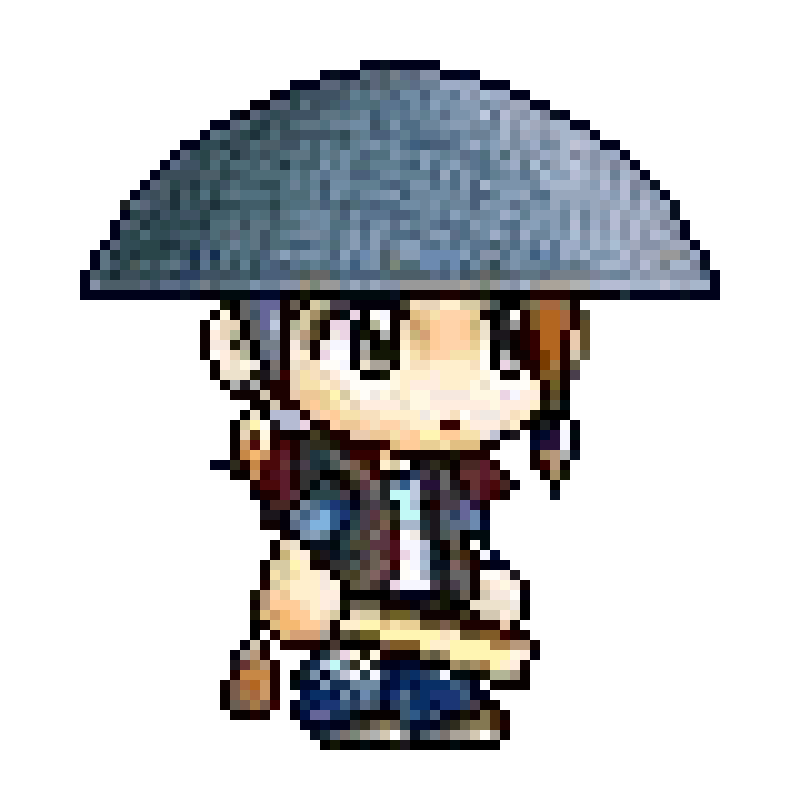