HTML:
<script id="tb_content" type="text/plain">{{content}}</script>
JS:
var app = new Vue({
el: "#app",
data: {
content: 'abc',
ue: {},
}, mounted: function () {
this.ue = UE.getEditor('tb_content');
}, methods: {
fn_save: function () {
console.log(this.ue.getContent())
}
}
});
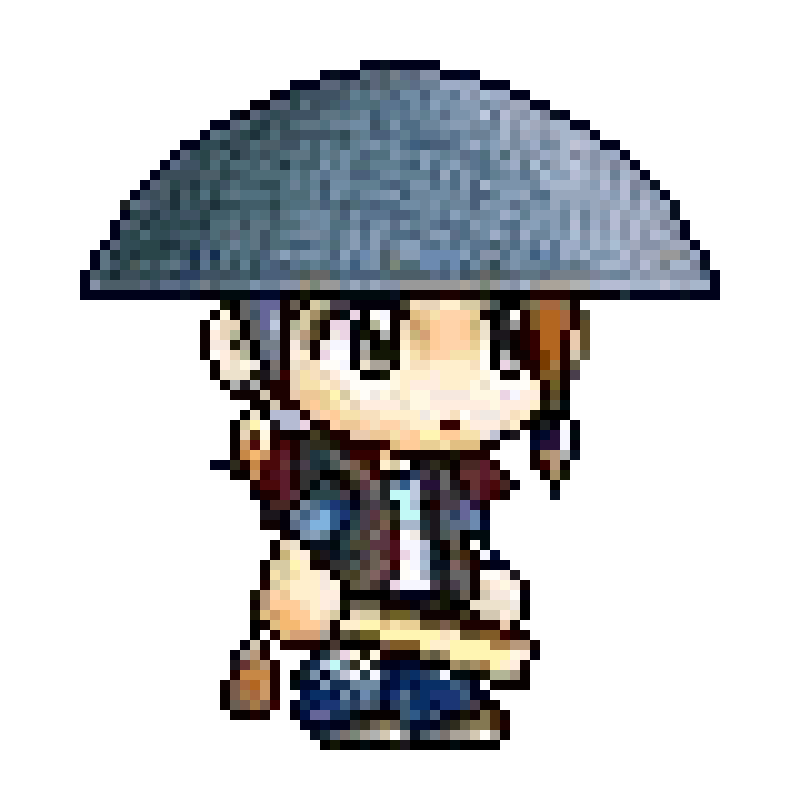
不要使用 CSS 方式设置 canvas 的宽高,应使用属性来设置其尺寸。
直接设置属性:
<canvas id="canvas" width="400" height="300"></canvas>
因属性值是以 px 为单位的,如果项目全程使用 rem 等其它尺寸单位,可通过 JS 来设置
var canvas = document.getElementById('canvas');
coatingW = $('#coating_frame').width();
coatingH = $('#coating_frame').height();
canvas.width = coatingW;
canvas.height = coatingH;
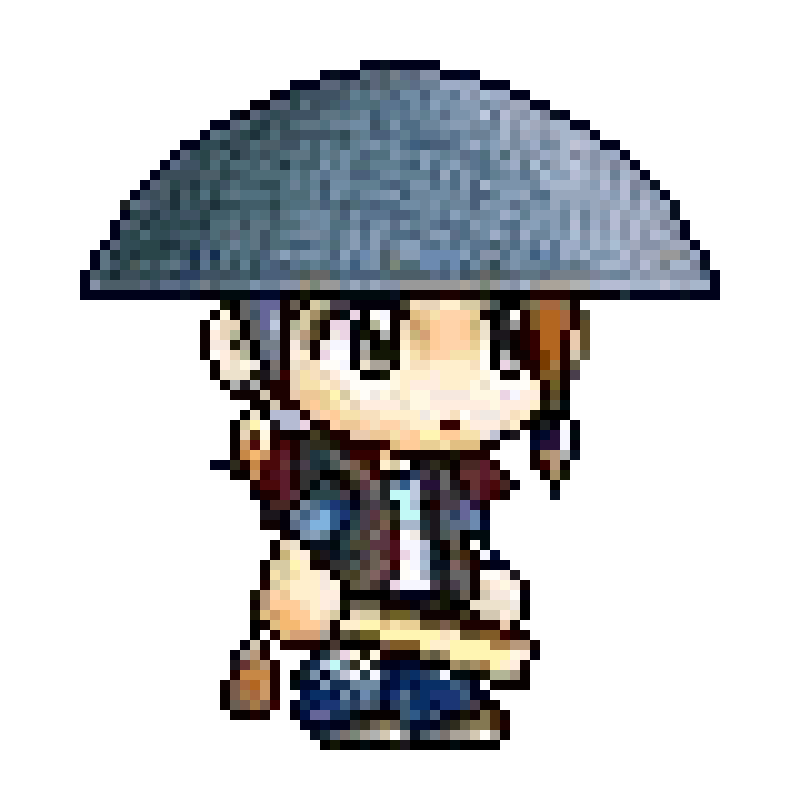
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
namespace xxx.xxx.xxx.Controllers
{
public class DiscuzTinyintViewerController : Controller
{
public IActionResult Index()
{
using var context = new Data.xxx.xxxContext();
var conn = context.Database.GetDbConnection();
conn.Open();
using var cmd = conn.CreateCommand();
cmd.CommandText = "SELECT `TABLE_NAME` FROM information_schema.TABLES WHERE TABLE_SCHEMA = DATABASE();";
Dictionary<string, List<FieldType>?> tables = new();
using var r = cmd.ExecuteReader();
while (r.Read())
{
tables.Add((string)r["TABLE_NAME"], null);
}
conn.Close();
foreach (var table in tables)
{
var conn2 = context.Database.GetDbConnection();
conn2.Open();
using var cmd2 = conn2.CreateCommand();
cmd2.CommandText = "DESCRIBE " + table.Key;
using var r2 = cmd2.ExecuteReader();
List<FieldType> fields = new();
while (r2.Read())
{
if (((string)r2[1]).Contains("tinyint(1)"))
{
fields.Add(new()
{
Field = (string)r2[0],
Type = (string)r2[1],
Null = (string)r2[2],
});
}
}
conn2.Close();
tables[table.Key] = fields;
}
foreach (var table in tables)
{
foreach (var f in table.Value)
{
var conn3 = context.Database.GetDbConnection();
conn3.Open();
using var cmd3 = conn3.CreateCommand();
cmd3.CommandText = $"SELECT {f.Field} as F, COUNT({f.Field}) as C FROM {table.Key} GROUP BY {f.Field}";
using var r3 = cmd3.ExecuteReader();
List<FieldType.ValueCount> vs = new();
while (r3.Read())
{
vs.Add(new() { Value = Convert.ToString(r3["F"]), Count = Convert.ToInt32(r3["C"]) });
}
conn3.Close();
f.groupedValuesCount = vs;
}
}
return Json(tables.Where(c => c.Value != null && c.Value.Count > 0));
}
private class FieldType
{
public string Field { get; set; }
public string Type { get; set; }
public string Null { get; set; }
public List<ValueCount> groupedValuesCount { get; set; }
public class ValueCount
{
public string Value { get; set; }
public int Count { get; set; }
}
public string RecommendedType
{
get
{
if (groupedValuesCount == null || groupedValuesCount.Count < 2)
{
return "无建议";
}
else if (groupedValuesCount.Count == 2 && groupedValuesCount.Any(c => c.Value == "0") && groupedValuesCount.Any(c => c.Value == "1"))
{
return "bool" + (Null == "YES" ? "?" : "");
}
else
{
return "sbyte" + (Null == "YES" ? "?" : "");
}
}
}
}
}
}
[{
"key": "pre_forum_post",
"value": [{
"field": "first",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "0",
"count": 1395501
}, {
"value": "1",
"count": 179216
}],
"recommendedType": "bool"
}, {
"field": "invisible",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "-5",
"count": 9457
}, {
"value": "-3",
"count": 1412
}, {
"value": "-2",
"count": 1122
}, {
"value": "-1",
"count": 402415
}, {
"value": "0",
"count": 1160308
}, {
"value": "1",
"count": 3
}],
"recommendedType": "sbyte"
}, {
"field": "anonymous",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "0",
"count": 1574690
}, {
"value": "1",
"count": 27
}],
"recommendedType": "bool"
}, {
"field": "usesig",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "0",
"count": 162487
}, {
"value": "1",
"count": 1412230
}],
"recommendedType": "bool"
}, {
"field": "htmlon",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "0",
"count": 1574622
}, {
"value": "1",
"count": 95
}],
"recommendedType": "bool"
}, {
"field": "bbcodeoff",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "-1",
"count": 935448
}, {
"value": "0",
"count": 639229
}, {
"value": "1",
"count": 40
}],
"recommendedType": "sbyte"
}, {
"field": "smileyoff",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "-1",
"count": 1359482
}, {
"value": "0",
"count": 215186
}, {
"value": "1",
"count": 49
}],
"recommendedType": "sbyte"
}, {
"field": "parseurloff",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "0",
"count": 1572844
}, {
"value": "1",
"count": 1873
}],
"recommendedType": "bool"
}, {
"field": "attachment",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "0",
"count": 1535635
}, {
"value": "1",
"count": 2485
}, {
"value": "2",
"count": 36597
}],
"recommendedType": "sbyte"
}, {
"field": "comment",
"type": "tinyint(1)",
"null": "NO",
"groupedValuesCount": [{
"value": "0",
"count": 1569146
}, {
"value": "1",
"count": 5571
}],
"recommendedType": "bool"
}]
}]
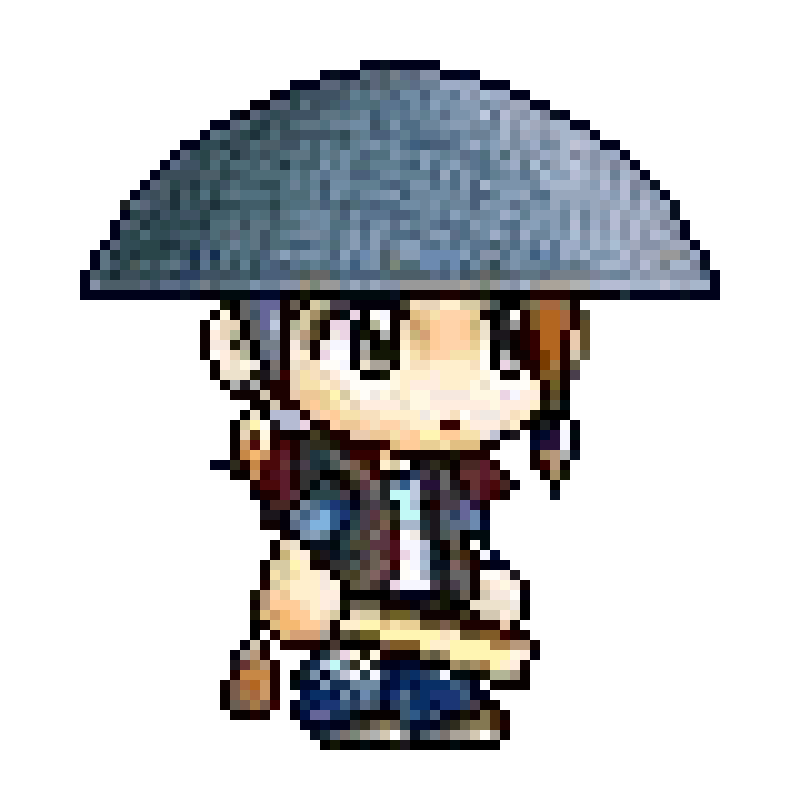
Regex.Escape(String) 方法:
通过替换为转义码来转义最小的字符集(\、*、+、?、|、{、[、(、)、^、$、.、# 和空白)。 这将指示正则表达式引擎按原义解释这些字符而不是解释为元字符。
示例:
string str = @"123\c\d\e";
string r1 = @"\d";
string r2 = Regex.Escape(r1);
return Json(new
{
m1 = Regex.Matches(str, r1).Select(c => c.Value),
m2 = Regex.Matches(str, r2).Select(c => c.Value)
});
结果:
{
"m1":[
"1",
"2",
"3"
],
"m2":[
"\\d"
]
}
一般地,我们使用通配符 a*c 在字符串 abcd 中查找:
string s = @"abcd";
string w = @"a*c";
string r = Regex.Escape(w).Replace(@"\*", @".*?").Replace(@"\?", @".?");
return Content(Regex.Match(s, r).Value);
结果:
abc
同理,使用通配符 \d*\f 在字符串 \a\b\c\d\e\f 中查找:
string s = @"\a\b\c\d\e\f";
string w = @"\d*\f";
string r = Regex.Escape(w).Replace(@"\*", @".*?").Replace(@"\?", @".?");
return Content(Regex.Match(s, r).Value);
结果:
\d\e\f
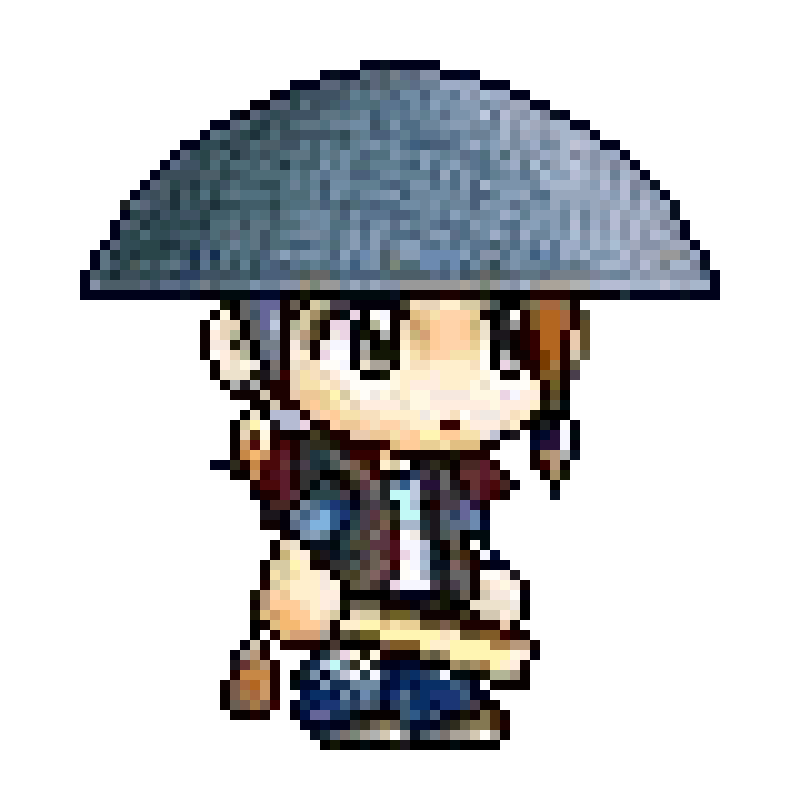
测试在长度为 403 的字符串中查找,特意匹配最后几个字符:
string a = "";
for (int i = 0; i < 100; i++) { a += "aBcD"; }
a += "xYz";
string b = "xyz"; // 特意匹配最后几个字符
Stopwatch sw1 = Stopwatch.StartNew();
bool? r1 = null;
for (int i = 0; i < 10000; i++) { r1 = a.IndexOf(b) >= 0; }
sw1.Stop();
Stopwatch sw2 = Stopwatch.StartNew();
bool? r2 = null;
for (int i = 0; i < 10000; i++) { r2 = a.Contains(b); }
sw2.Stop();
Stopwatch sw3 = Stopwatch.StartNew();
bool? r3 = null;
for (int i = 0; i < 10000; i++) { r3 = a.ToUpper().Contains(b.ToUpper()); }
sw3.Stop();
Stopwatch sw4 = Stopwatch.StartNew();
bool? r4 = null;
for (int i = 0; i < 10000; i++) { r4 = a.ToLower().Contains(b.ToLower()); }
sw4.Stop();
Stopwatch sw5 = Stopwatch.StartNew();
bool? r5 = null;
for (int i = 0; i < 10000; i++) { r5 = a.Contains(b, StringComparison.OrdinalIgnoreCase); }
sw5.Stop();
Stopwatch sw6 = Stopwatch.StartNew();
bool? r6 = null;
for (int i = 0; i < 10000; i++) { r6 = a.Contains(b, StringComparison.CurrentCultureIgnoreCase); }
sw6.Stop();
Stopwatch sw7 = Stopwatch.StartNew();
bool? r7 = null;
for (int i = 0; i < 10000; i++) { r7 = Regex.IsMatch(a, b); }
sw7.Stop();
Stopwatch sw8 = Stopwatch.StartNew();
bool? r8 = null;
for (int i = 0; i < 10000; i++) { r8 = Regex.IsMatch(a, b, RegexOptions.IgnoreCase); }
sw8.Stop();
return Json(new
{
IndexOf_________________ = sw1.Elapsed + " " + r1,
Contains________________ = sw2.Elapsed + " " + r2,
ToUpper_________________ = sw3.Elapsed + " " + r3,
ToLower_________________ = sw4.Elapsed + " " + r4,
OrdinalIgnoreCase_______ = sw5.Elapsed + " " + r5,
CurrentCultureIgnoreCase = sw6.Elapsed + " " + r6,
IsMatch_________________ = sw7.Elapsed + " " + r7,
IsMatchIgnoreCase_______ = sw8.Elapsed + " " + r8,
});
结果参考:
{
"indexOf_________________": "00:00:00.1455812 False",
"contains________________": "00:00:00.0003791 False",
"toUpper_________________": "00:00:00.0038182 True",
"toLower_________________": "00:00:00.0026113 True",
"ordinalIgnoreCase_______": "00:00:00.0096550 True",
"currentCultureIgnoreCase": "00:00:00.1596517 True",
"isMatch_________________": "00:00:00.0053627 False",
"isMatchIgnoreCase_______": "00:00:00.0084132 True"
}
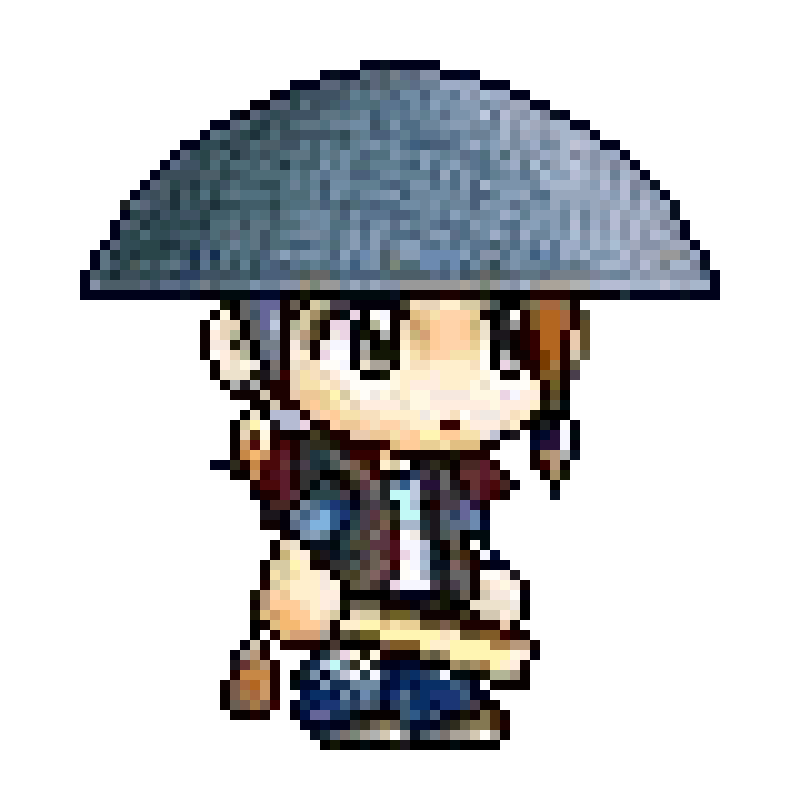
SelectMany() 的作用与 GroupBy() 相反,例:
List<List<int>> list = new() { new() { 1, 2, 3 }, new() { 4, 5, 6 } };
return Json(list.SelectMany(c => c));
输出:
[1,2,3,4,5,6]
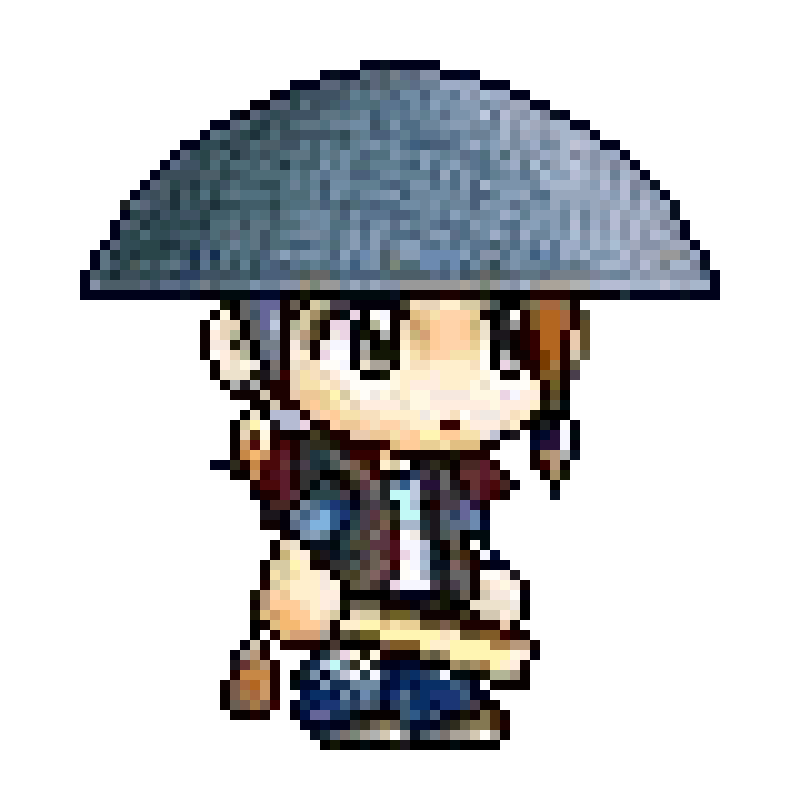
System.NotSupportedException: Serialization and deserialization of 'System.DateOnly' instances are not supported.
The unsupported member type is located on type 'System.Nullable`1[System.DateOnly]'.
解决方法:
https://www.nuget.org/packages/DateOnlyTimeOnly.AspNet/
builder.Services
.AddControllers(options => options.UseDateOnlyTimeOnlyStringConverters())
.AddJsonOptions(options => options.UseDateOnlyTimeOnlyStringConverters());
POST
axios.post('AjaxCases', {
following: true,
success: null,
page: 1,
}).then(function (response) {
console.log(response.data)
}).catch(function (error) {
console.log(error.response.data);
});
[HttpPost]
public IActionResult AjaxCases([FromBody] AjaxCasesRequestModel req)
{
bool? following = req.following;
bool? success = req.success;
int page = req.page;
}
GET
axios.get('AjaxCase', {
params: {
int: 123,
}
}).then(function (response) {
console.log(response.data)
}).catch(function (error) {
console.log(error.response.data);
});
[HttpGet]
public IActionResult AjaxCases([FromQuery] int id)
{
}
DELETE
格式与 GET 类似
* 若服务端获取参数值为 null,可能的情况如下:
请检查相关枚举类型是否已设置 [JsonConverter] 属性,参:C# 枚举(enum)用法笔记,且传入的值不能为空字符串,可以传入 null 值,并将服务端 enum 类型改为可空。可以以 string 方式接收参数后再进行转换。
模型中属性名称不能重复(大小写不同也不行)。
布尔型属性值必须传递布尔型值,即在 JS 中,字符串 "true" 应 JSON.parse("true") 为 true 后再回传给服务端。
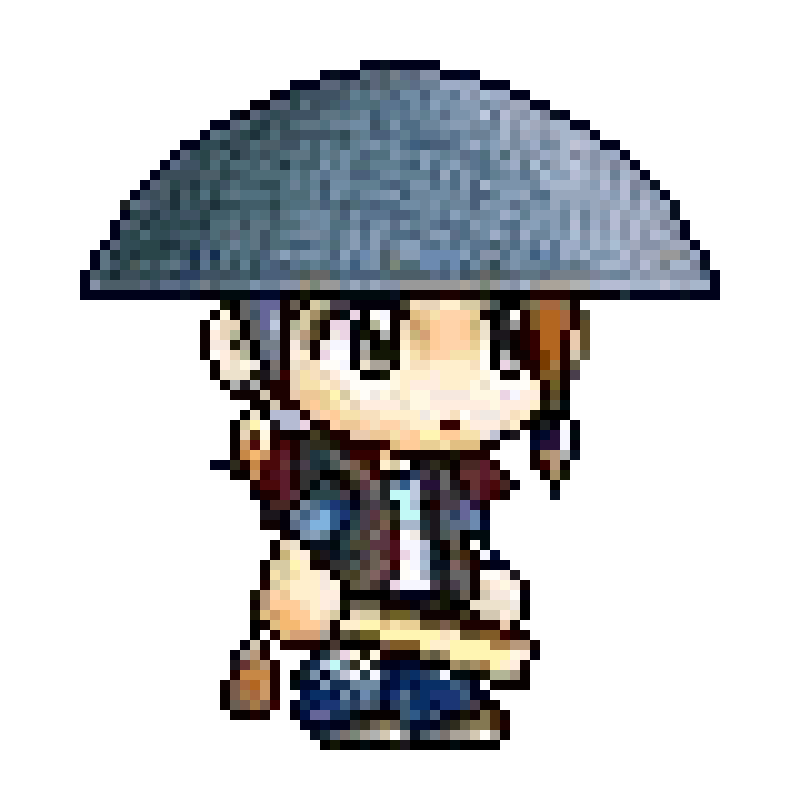
iPhone 或安卓上传的照片,可能因横向或纵向拍摄,导致上传后照片显示成 90 度、180 度、270 度角旋转,使用 C# 处理该照片时同样会遇到相同的问题。
解决的方案:
客户端使用 base64 显示图片,可按正常方向显示,且不通过服务端即时显示。关键代码:
$('#file_input').change(function (e) {
if (e.target.files.length == 0) { return; }
// file 转换为 base64
var reader = new FileReader();
reader.readAsDataURL(e.target.files[0]);
reader.onload = function (event) {
$('#myImg').attr('src', event.target.result).css({ width: 'auto', height: 'auto' });
}
});
$('#myImg').on('load', function () {
console.log('图片加载完成')
});
但 base64 字符串传递到服务端受请求大小限制(一般比直接 POST 文件要小)。所以显示图片的同时应即时使用 POST 方式上传图片(如 jquery.fileupload.js)。
当然如果是在微信中打开网页,使用 JS-SDK 的 wx.chooseImage() 返回的 localId 也可以在 <img /> 中正常显示,然后使用 wx.uploadImage() 上传到微信服务器并获取 serverId,在通过获取临时素材接口取回图片文件。
服务端如果需要处理该图片(缩放、裁切、旋转等),需要先读取照片的拍摄角度,旋正后,再进行处理,相关 C# 代码:
/// <summary>
/// 调整照片的原始拍摄旋转角度
/// </summary>
/// <param name="img"></param>
/// <returns></returns>
private Image ImageRotateFlip(Image img)
{
foreach (var prop in img.PropertyItems)
{
if (prop.Id == 0x112)
{
switch (prop.Value[0])
{
case 6: img.RotateFlip(RotateFlipType.Rotate90FlipNone); break;
case 3: img.RotateFlip(RotateFlipType.Rotate180FlipNone); break;
case 8: img.RotateFlip(RotateFlipType.Rotate270FlipNone); break;
}
prop.Value[0] = 1;
}
}
return img;
}
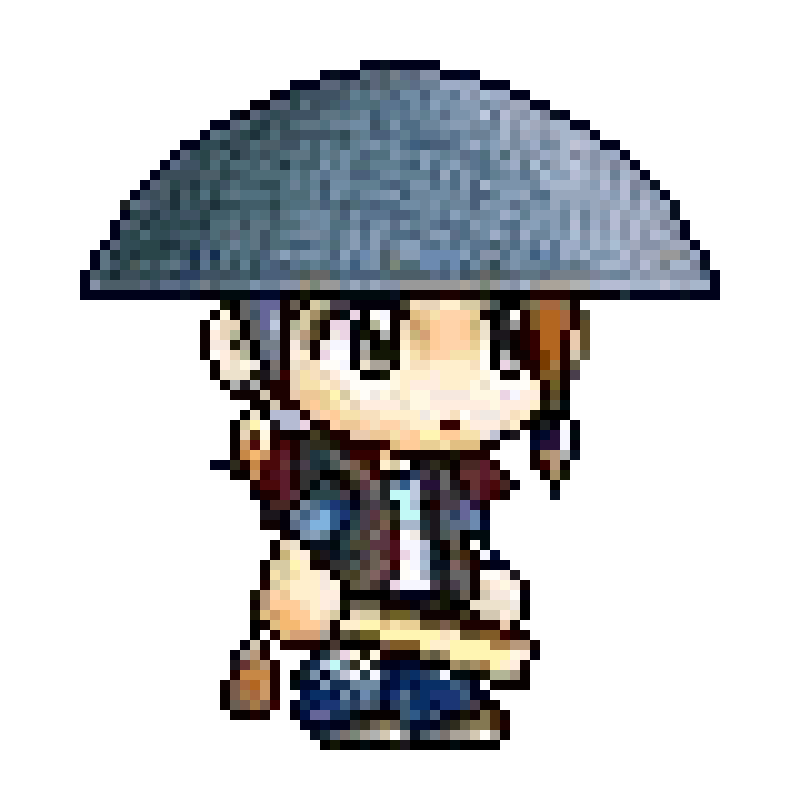