测试表数据量 152 万条,测试跳过 100 万取 10 条。
测试一:直接使用 limit 跳过 1000000 取 10,耗时 2.4s;
SELECT *
FROM `pre_forum_post`
ORDER BY `pid`
LIMIT 1000000, 10
> OK
> 时间: 2.394s
测试二:先使用 limit 跳过 1000000 取 1,再使用配合 where 条件,使用 limit 取 10,耗时 0.2s。
SELECT *
FROM `pre_forum_post`
WHERE `pid` >= (
SELECT `pid`
FROM `pre_forum_post`
ORDER BY `pid`
LIMIT 1000000, 1
)
ORDER BY `pid`
LIMIT 10
> OK
> 时间: 0.207s
两次测试耗时相差约 10 倍。测试取 100 条或取 1 条,耗时都相差约 10 倍。
但若测试跳过条数较小时,测试一效率更高,因此应按照项目的实际需要选择适当的查询方法。
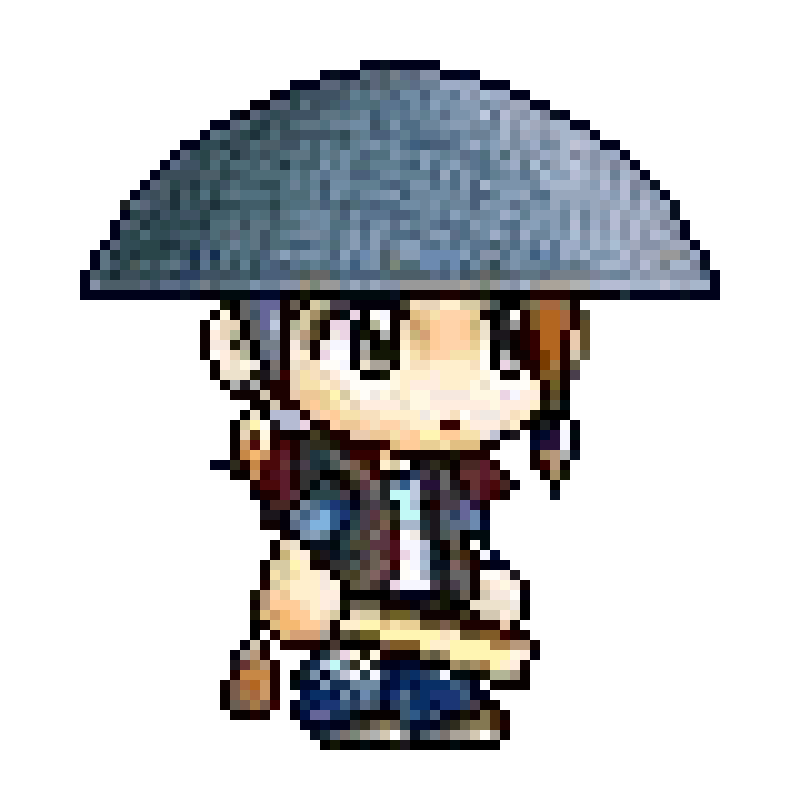
按产品类型查询:费用 - 费用分析,右侧可筛选产品,可按月按天查询,自动合计
按实例查询:费用 - 费用账单,搜索实例ID,按月查询
另:已通过提交工单确认无法查询 OSS 按 Bucket 的账单及 CDN 按域名的账单。
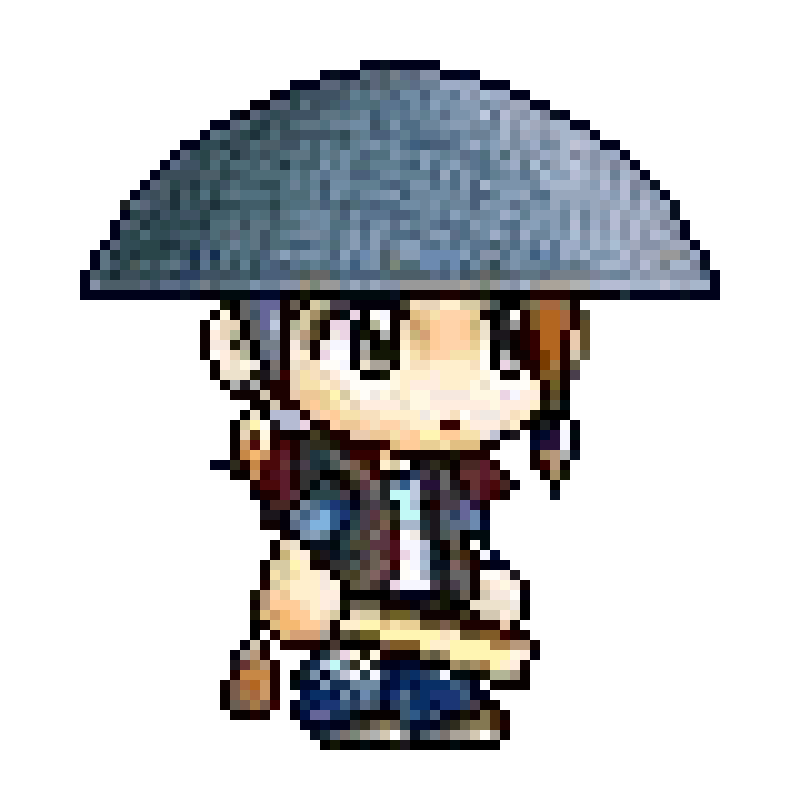
“DatabaseFacade”未包含“ExecuteSqlCommand”的定义,并且找不到可接受第一个“DatabaseFacade”类型参数的可访问扩展方法“ExecuteSqlCommand”(是否缺少 using 指令或程序集引用?)
思路:(以 Entity Framework Core 5.0 为例)
先借用 SELETE 的 IQueryable 语句,将 SELETE 关键字替换成 DELETE,再使用 FromSqlRaw 执行。这样可准确使用真实的数据库表名和字段名。
如:
using Microsoft.EntityFrameworkCore;
string sql = db.表.Where(c => c.Date < minDate).ToQueryString();
sql = Regex.Replace(sql, @"SELECT\s.*?\sFROM", "DELETE FROM", RegexOptions.Singleline); // SET @__minDate_0 = '2020-01-14'; DELETE FROM `表` AS `d` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"\sAS\s`(.*?)`", "", RegexOptions.Singleline); // SET @__minDate_0 = '2020-01-14'; DELETE FROM `表` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"`([^`]*?)`\.`", "`", RegexOptions.Singleline); // SET @__minDate_0 = '2020-01-14'; DELETE FROM `表` WHERE `Date` < @__minDate_0
db.Database.ExecuteSqlRaw(sql);
以上例子针对 MySQL,且未考虑字符串内容中包含符号 ` 的情况。实例操作中应严格防范 SQL 注入。
封装成方法:
using Microsoft.EntityFrameworkCore;
const RegexOptions ro = RegexOptions.Singleline;
/// <summary>
/// 组装一个 DELETE SQL 语句
/// </summary>
/// <typeparam name="T">数据库表实体类</typeparam>
/// <param name="queryableWithConditions">IQueryable,可带筛选条件</param>
/// <returns></returns>
public static string AssembleDeleteSql<T>(IQueryable<T> queryableWithConditions) where T : class
{
// MySQL 版
string sql = queryableWithConditions.ToQueryString(); // SET @__minDate_0 = '2020-01-14'; SELECT `d`.`Date`, `d`.`Code6` FROM `表` AS `d` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"SELECT\s.*?\sFROM", "DELETE FROM", ro); // SET @__minDate_0 = '2020-01-14'; DELETE FROM `表` AS `d` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"\sAS\s`(.*?)`", "", ro); // SET @__minDate_0 = '2020-01-14'; DELETE FROM `表` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"`([^`]*?)`\.`", "`", ro); // SET @__minDate_0 = '2020-01-14'; DELETE FROM `表` WHERE `Date` < @__minDate_0
return sql;
}
/// <summary>
/// 组装一个 UPDATE SQL 语句
/// </summary>
/// <typeparam name="T">数据库表实体类</typeparam>
/// <param name="queryableWithConditions">IQueryable,可带筛选条件</param>
/// <param name="columnsAndValues">需要更改的字段和值</param>
/// <returns></returns>
public static string AssembleUpdateSql<T>(IQueryable<T> queryableWithConditions, Dictionary<string, object> columnsAndValues) where T : class
{
// MySQL 版
string sql = queryableWithConditions.ToQueryString(); // SET @__minDate_0 = '2020-01-14'; SELECT `d`.`Date`, `d`.`Code6` FROM `表` AS `d` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"SELECT\s.*?\sFROM", "UPDATE", ro); // SET @__minDate_0 = '2020-01-14'; UPDATE `表` AS `d` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"\sAS\s`(.*?)`", "", ro); // SET @__minDate_0 = '2020-01-14'; UPDATE `表` WHERE `d`.`Date` < @__minDate_0
sql = Regex.Replace(sql, @"`([^`]*?)`\.`", "`", ro); // SET @__minDate_0 = '2020-01-14'; UPDATE `表` WHERE `Date` < @__minDate_0
string sets = string.Join(",", columnsAndValues.Select(c => $"`{c.Key.Replace("`", @"\`")}` = '{c.Value.ToString().Replace("'", @"\'")}'"));
sql = Regex.Replace(sql, @"`\s*WHERE", $"` SET {sets} WHERE", ro); // SET @__minDate_0 = '2020-01-14'; UPDATE `表` SET `field1` = 'value1', `field2` = 'value2' WHERE `Date` < @__minDate_0
return sql;
}
调用示例:
using Microsoft.EntityFrameworkCore;
// 删除记录
DateTime minDate = DateTime.Today.AddYears(-1).AddMonths(-1);
string sql = AssembleDeleteSql(db.表.Where(c => c.Date < minDate));
db.Database.ExecuteSqlRaw(sql);
// 更新记录
var sets = new Dictionary<string, object>();
foreach (var p in db.Model.FindEntityType(typeof(表)).GetProperties())
{
switch (p.Name)
{
case nameof(表.字段一): sets.Add(p.GetColumnBaseName(), 123); break;
case nameof(表.字段二): sets.Add(p.GetColumnBaseName(), 456); break;
}
}
DateTime minDate = DateTime.Today.AddYears(-1).AddMonths(-1);
string sql = AssembleUpdateSql(db.表.Where(c => c.Date < minDate), sets);
db.Database.ExecuteSqlRaw(sql);
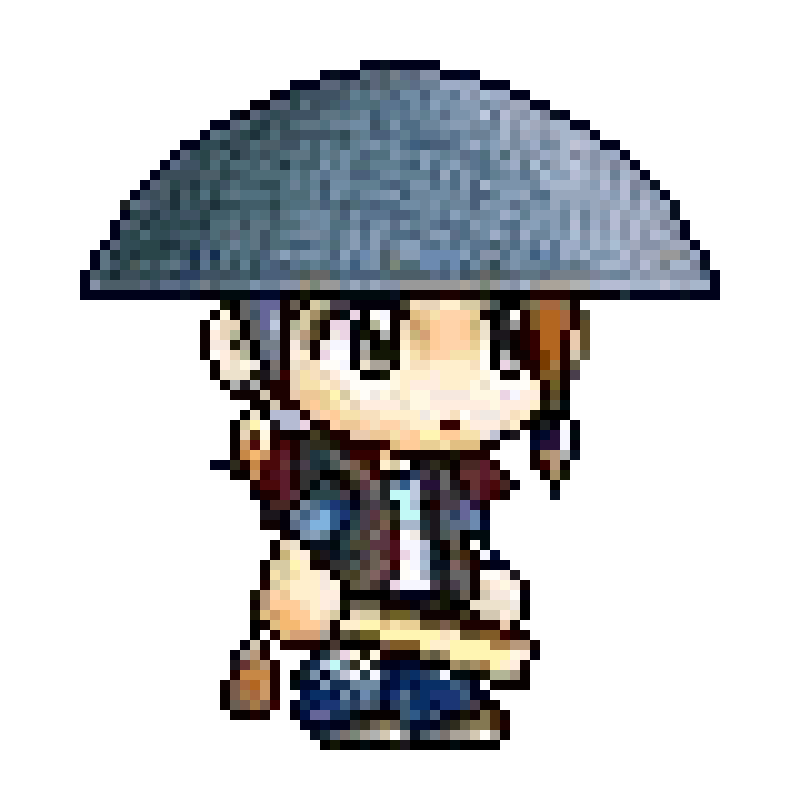
纯真 IP 数据库官方下载地址:http://www.cz88.net/
下载安装纯真 IP,将 qqwry.dat 文件复制到项目下。(本文以放在 /App_Data/ 目录下为例)
在 qqwry.dat 上右键打开属性窗口,将“复制到输出目录”切换到“始终复制”或“如果较新则复制”。
通过 nuget 安装 QQWry,喜欢依赖注入方式的可以选择 QQWry.DependencyInjectio。
var config = new QQWryOptions
{
DbPath = dbPath ?? (AppContext.BaseDirectory + @"App_Data\qqwry.dat")
};
var ipSearch = new QQWryIpSearch(config);
var ipl = ipSearch.GetIpLocation(ip);
return new IpLocation
{
Ip = ipl.Ip,
Country = ipl.Country?.Replace("CZ88.NET", "").Trim(),
Area = ipl.Area?.Replace("CZ88.NET", "").Trim(),
};
github 开源地址:https://github.com/JadynWong/IP_qqwry
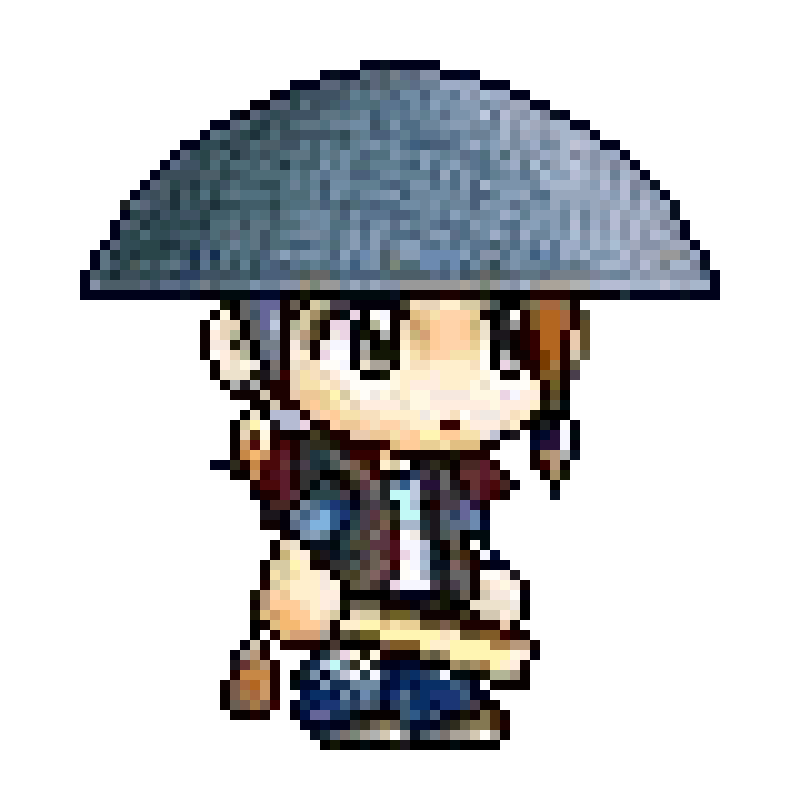
核心文件路径:/theme/html/demo*/src/js/components/core.datatable.js
所有参数的默认值见该文件 3369 行起。
data:
属性 | 功能 | 值 |
type | 数据源类型 | local / remote |
source | 数据源 | 链接或对象(见下方) |
pageSize | 每页项数 | 默认 10 |
saveState | 刷新、重新打开、返回时仍保持状态 | 默认 true |
serverPaging | 是否在服务端实现分页 | 默认 false |
serverFiltering | 是否在服务端实现筛选 | 默认 false |
serverSorting | 是否在服务端实现排序 | 默认 false |
autoColumns | 为远程数据源启用自动列功能 | 默认 false |
attr |
data.source:
属性 | 功能 | 值 |
url | 数据源地址 | |
params | 请求参数 |
|
headers |
自定义请求的头 |
|
map | 数据地图,作用是对返回的数据进行整理和定位 |
|
layout:
属性 | 功能 | 值 |
theme | 主题 | 默认 default |
class | 包裹的 CSS 样式 | |
scroll | 在需要时显示横向或纵向滚动条 | 默认 false |
height | 表格高度 | 默认 null |
minHeight | 表格最小高度 | 默认 null |
footer | 是否显示表格底部 | 默认 false |
header | 是否显示表头 | 默认 true |
customScrollbar | 自定义的滚动条 | 默认 true |
spinner |
Loading 样式 |
|
icons | 表格中的 icon |
|
sortable | 是否支持按列排序 | 默认 true |
resizable |
是否支持鼠标拖动改变列宽 | 默认 false |
filterable | 在列中过滤 | 默认 false |
pagination |
显示分页信息 | 默认 true |
editable |
行内编辑 | 默认 false |
columns |
列 | 见本文下方 |
search |
搜索 |
|
layout.columns:
属性 | 功能 | 解释 |
field | 字段名 | 对应 JSON 的属性名,点击表头时作为排序字段名 |
title | 表头名 | 显示在表格头部 |
sortable | 默认排序方式 | 可选:'asc' / 'desc' |
width | 单元格最小宽度 | 值与 CSS 值一致,填数字时默认单位 px |
type | 数据类型 | 'number' / 'date' 等,与本地排序有关 |
format | 数据格式化 | 例格式化日期:'YYYY-MM-DD' |
selector | 是否显示选择框 | 布尔值或对象,如:{ class: '' } |
textAlign | 文字对齐方式 | 'center' |
overflow | 内容超过单元格宽度时是否显示 | 'visible':永远显示 |
autoHide | 自适应显示/隐藏 | 布尔值 |
template | 用于显示内容的 HTML 模板 | function(row) { return row.Id; } |
sortCallback | 排序回调 | 自定义排序方式,参 local-sort.js |
其它:
属性 | 功能 | 解释 |
translate | 翻译 |
参 core.datatable.js 3512 行,简体中文示例:
|
extensions |
暂时没有找到对字符串内容进行自动 HTML 编码的属性,这可能带来 XSS 攻击风险,在 remote 方式中必须在服务端预先 HtmlEncode。即使在 layout.columns.template 中进行处理也是无济于事,恶意代码会在 ajax 加载完成后立即执行。
方法和事件:待完善。
更多信息请查询官方文档:https://keenthemes.com/keen/?page=docs§ion=html-components-datatable
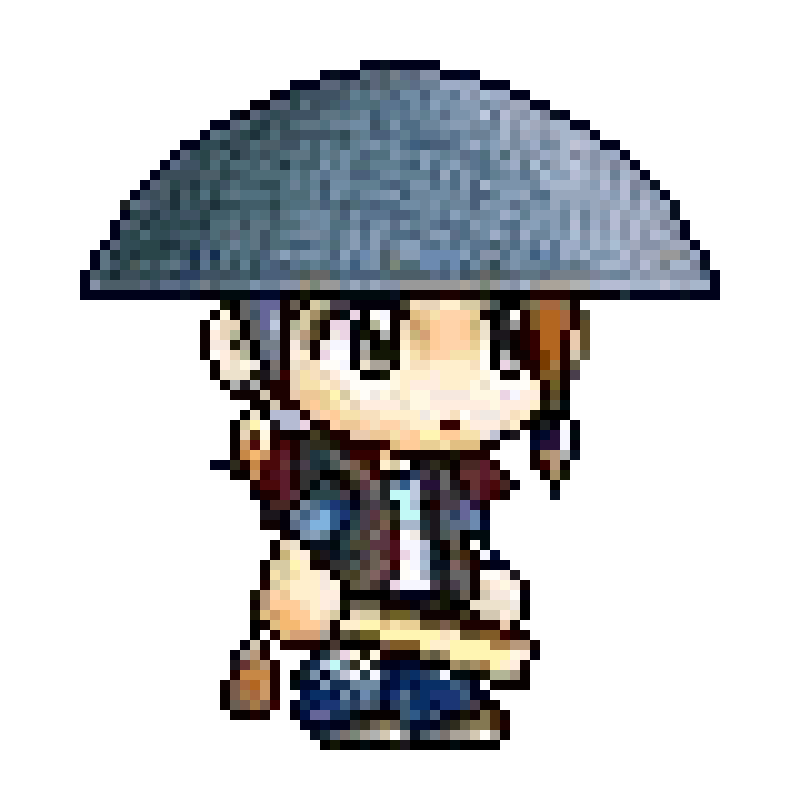
The cast to value type 'System.Int32' failed because the materialized value is null. Either the result type's generic parameter or the query must use a nullable type.
在 EF 查询数据库时发生:
var list = db.TableA.Select(a => new
{
a.Id,
bSum = a.TableB.Sum(b => b.Num),
}).ToList();
本例中 TableB 有外键关联到 TableA.Id,TableB.Num 为 int,而非 int?。
原因是 EF 以为 Sum 的结果可能为 Nullable<int>,将代码修改如下即可正常:
var list = db.TableA.Select(a => new
{
a.Id,
bSum = (int?)a.TableB.Sum(b => b.Num) ?? 0,
}).ToList();
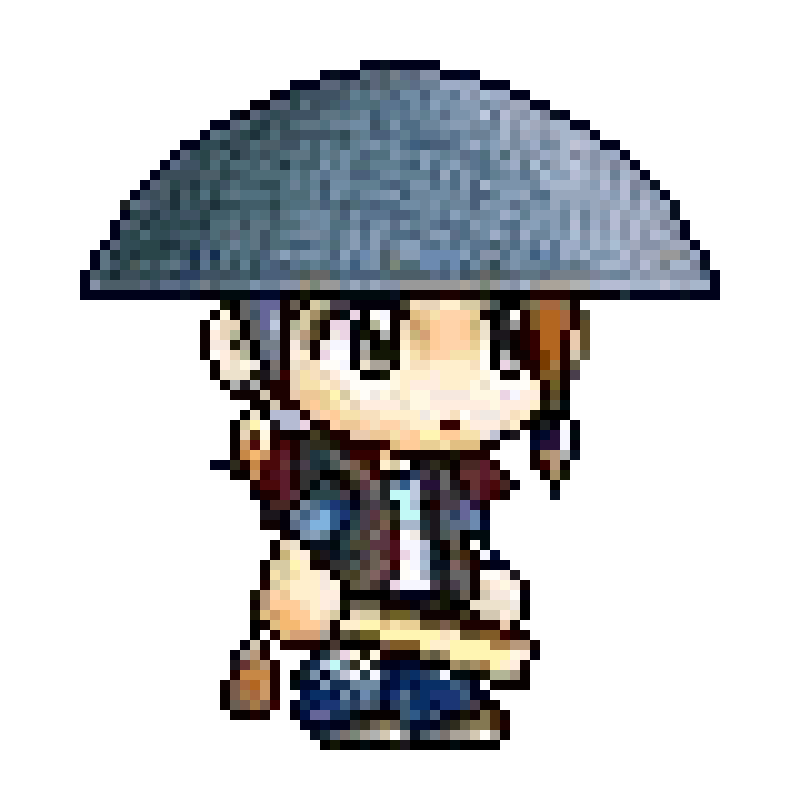
关键词:prefers-color-scheme
方案一:CSS
媒体查询为开发者提供了系统主题检测的属性:prefers-color-scheme
,有三个可用的值:no-preference
、light
和 dark
。
/* light mode */
@media (prefers-color-scheme: light) {
body {
background-color: #f0f0f0;
}
}
/* dark mode */
@media (prefers-color-scheme: dark) {
body {
background-color: #3e3e3e;
color: #fff;
}
}
方案二:JS
if (window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches) {
// dark mode
}
经 2020.02.22 提交工单咨询回复,阿里云控制台暂无法针对 CDN 的域名或 OSS 的 Bucket 来区分费用账单,但 ECS、RDS 可根据实例 ID 来查询:
虽然不能准确查询 CDN 各域名的消费情况,但我们可以在 CDN 的统计分析中查询域名排行,从而可以按流量占比再结合 CDN 的总体费用来计算各域名的大致费用:
当然,能把 HTTPS 等增值服务和资源包等因素考虑进去会更加合理。
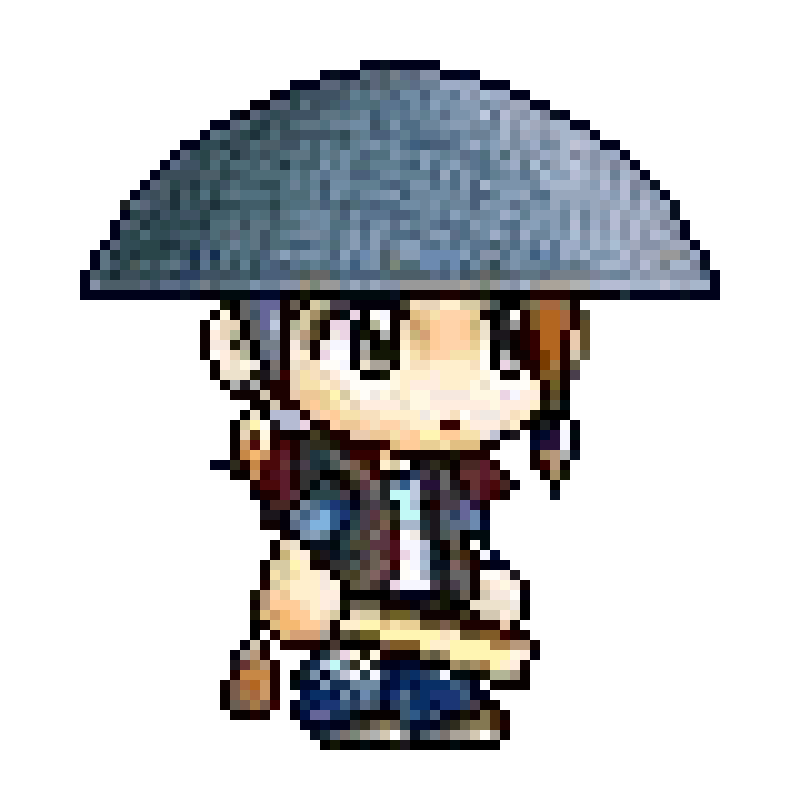
在数据库连接字符串可设置是否将 tinyint(1) 映射为 bool,否则为 sbyte:
TreatTinyAsBoolean=false/true
tinyint(1) 一般用于表示 bool 型字段,存储内容为 0 或 1,但有时候也用来存储其它数字。
以 Discuz! 的表 pre_forum_post 为例,字段 first 和 invisible 都是 tinyint(1),但 first 只储存 0 和 1,invisible 却有 -1、-5 之类的值。
因此我们一般设置 TreatTinyAsBoolean=false,程序中 first 与 invisible 均以 sbyte 处理。
设置 TreatTinyAsBoolean=true 后,EF 或 EF Core 自动将该类型映射为 bool,方便在程序中作进一步处理。
一旦设置 TreatTinyAsBoolean=true,那么所有查询结果中 tinyint(1) 字段的返回值永远只有 1 和 0(即 True/False),即使真实值为 -1,也返回 1。
因为我们必须在确保所有的 tinyint(1) 类型字段都仅表示布尔值是才设置 TreatTinyAsBoolean=true。
一旦部分 tinyint(1) 类型字段用于存放 0 和 1 以外的数,那么就应该设置 TreatTinyAsBoolean=false。
在数据库优先的项目中,以 TreatTinyAsBoolean=false 生成数据模型后,可将明确为布尔类型的字段改为 bool。列出 MySQL 数据库中所有表所有字段中类型为 tinyint(1) 的字段值
以 .edmx 为例:
在项目中搜索该字段名,在搜索结果中找到 .edmx 文件中的两处。
.edmx 文件中的注释已经表明其包含 SSDL、CSDL、C-S mapping 三块内容,
在 SSDL content 下方找到该字段:
<Property Name="字段名" Type="tinyint" Nullable="***" />
改为
<Property Name="字段名" Type="bool" Nullable="***" />
在 CSDL content 下方找到该属性:
<Property Name="属性名" Type="SByte" Nullable="***" />
改为
<Property Name="属性名" Type="Boolean" Nullable="***" />
在解决方案管理器中展开 .edmx ->库名.tt -> 表名.cs 文件,
将模型类中的属性
public sbyte invisible { get; set; }
改为
public bool invisible { get; set; }
或 sbyte? 改为 bool?。
在 EF Core 中,直接打开对应数据表的 .cs 文件,更改属性类型即可。
相关报错:
错误: 指定的成员映射无效。类型中的成员的类型“Edm.SByte[Nullable=False,DefaultValue=]”与类型中的成员的“MySql.bool[Nullable=False,DefaultValue=]”不兼容。
InvalidOperationException: The property '***' is of type 'sbyte' which is not supported by the current database provider. Either change the property CLR type, or ignore the property using the '[NotMapped]' attribute or by using 'EntityTypeBuilder.Ignore' in 'OnModelCreating'.
尝试先连接一次能解决此问题(概率),非常的莫名其妙:
using Data.Discuz.db_bbs2021Context dbd = new();
var conn = dbd.Database.GetDbConnection();
conn.Open();
conn.Close();
参考:
https://mysqlconnector.net/connection-options/
https://stackoverflow.com/questions/6656511/treat-tiny-as-boolean-and-entity-framework-4
2023年1月注:本文适用于 Pomelo.EntityFrameworkCore.MySql 6.0,升级到 7.0 后会出现:
System.InvalidOperationException:“The 'sbyte' property could not be mapped to the database type 'tinyint(1)' because the database provider does not support mapping 'sbyte' properties to 'tinyint(1)' columns. Consider mapping to a different database type or converting the property value to a type supported by the database using a value converter. See https://aka.ms/efcore-docs-value-converters for more information. Alternately, exclude the property from the model using the '[NotMapped]' attribute or by using 'EntityTypeBuilder.Ignore' in 'OnModelCreating'.”
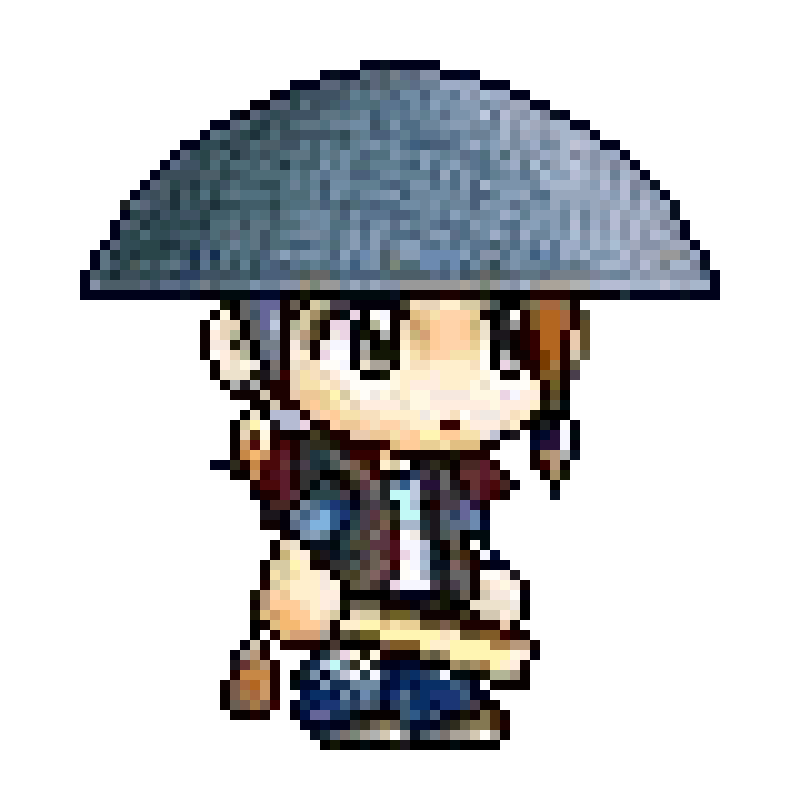
Nuget 安装:X.PagedList.Mvc.Core
控制器:
using X.PagedList;
public IActionResult Index(int page = 1)
{
……
return View(q.ToPagedList(page, size));
}
视图:
@using X.PagedList
@using X.PagedList.Mvc.Core
@model IPagedList<xxx>
@Html.PagedListPager(Model, page => Url.Action("Index", new { page }))
自定义(options 默认值):
@Html.PagedListPager(
Model,
page => Url.Action("Index", new { page }),
new X.PagedList.Mvc.Common.PagedListRenderOptionsBase
{
HtmlEncoder = HtmlEncoder.get_Default(),
DisplayLinkToFirstPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToLastPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToPreviousPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToNextPage = PagedListDisplayMode.IfNeeded,
DisplayLinkToIndividualPages = true,
DisplayPageCountAndCurrentLocation = false, // 显示总页数和当前页码
MaximumPageNumbersToDisplay = 10, // 最多显示页码数
DisplayEllipsesWhenNotShowingAllPageNumbers = true,
EllipsesFormat = "…",
LinkToFirstPageFormat = "<<",
LinkToPreviousPageFormat = "<",
LinkToIndividualPageFormat = "{0}",
LinkToNextPageFormat = ">",
LinkToLastPageFormat = ">>",
PageCountAndCurrentLocationFormat = "Page {0} of {1}.",
ItemSliceAndTotalFormat = "Showing items {0} through {1} of {2}.",
FunctionToDisplayEachPageNumber = null,
ClassToApplyToFirstListItemInPager = null,
ClassToApplyToLastListItemInPager = null,
ContainerDivClasses = new string[1]
{
"pagination-container"
},
UlElementClasses = new string[1]
{
"pagination"
},
LiElementClasses = Enumerable.Empty<string>(),
PageClasses = Enumerable.Empty<string>(),
UlElementattributes = null,
ActiveLiElementClass = "active",
EllipsesElementClass = "PagedList-ellipses",
PreviousElementClass = "PagedList-skipToPrevious",
NextElementClass = "PagedList-skipToNext",
})
保留地址栏参数:
@{
string query = Context.Request.QueryString.Value;
}
@Html.PagedListPager(Model, page => Regex.IsMatch(query, @"[?&]page=\d+")
? Regex.Replace(query, @"([?&])page=\d+", $"$1page={page}")
: (query.StartsWith("?") ? $"{query}&page={page}" : $"{query}?page={page}"),
new X.PagedList.Web.Common.PagedListRenderOptionsBase
{
DisplayPageCountAndCurrentLocation = true,
MaximumPageNumbersToDisplay = 5,
})
这里从查询字符串中判断并替换 page 值,如果有更简单的方法敬请告知。比如 Webdiyer 的分页组件会自动携带所有参数。
更多使用方式参官方文档:https://github.com/dncuug/X.PagedList
附应用于 Unify Template(一款基于 Bootstrap 的 HTML 模板)中的配置:
<style>
.u-pagination-v1-1--active .u-pagination-v1-1 { color: #fff; border-color: #72c02c; }
.PagedList-pageCountAndLocation { float: right !important; }
</style>
@{
string query = Context.Request.QueryString.Value;
}
@Html.PagedListPager(Model, page => Regex.IsMatch(query, @"[?&]page=\d+")
? Regex.Replace(query, @"([?&])page=\d+", $"$1page={page}")
: (query.StartsWith("?") ? $"{query}&page={page}" : $"{query}?page={page}"),
new X.PagedList.Web.Common.PagedListRenderOptionsBase
{
DisplayPageCountAndCurrentLocation = true,
MaximumPageNumbersToDisplay = 5,
UlElementClasses = new string[] { "list-inline" },
LiElementClasses = new string[] { "list-inline-item" },
PageClasses = new string[] { "u-pagination-v1__item", "u-pagination-v1-1", "g-pa-7-14" },
ActiveLiElementClass = "u-pagination-v1-1--active",
EllipsesElementClass = "g-pa-7-14",
})
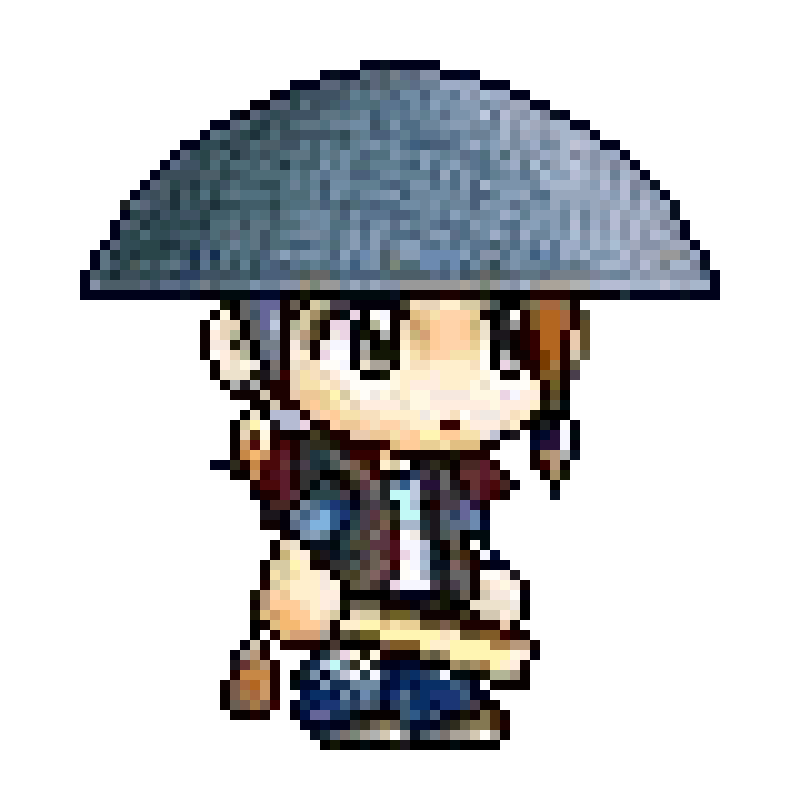