本文介绍 ASP.NET 的 Swagger 部署,若您使用 ASP.NET Core 应用程序,请移步 ASP.NET Core Web API Swagger 官方文档:
https://github.com/domaindrivendev/Swashbuckle.AspNetCore
安装
NuGet 中搜索安装 Swashbuckle,作者 Richard Morris
访问
http://您的域名/swagger
配置
显示描述
以将描述文件(xml)存放到项目的 bin 目录为例:
打开项目属性,切换到“生成”选项卡
在“配置”下拉框选择“所有配置”
更改“输出路径”为:bin\
勾选“XML 文档文件”:bin\******.xml,(默认以程序集名称命名)
打开文件:App_Start/SwaggerConfig.cs
取消注释:c.IncludeXmlComments(GetXmlCommentsPath());
添加方法:
public static string GetXmlCommentsPath() { return System.IO.Path.Combine( System.AppDomain.CurrentDomain.BaseDirectory, "bin", string.Format("{0}.xml", typeof(SwaggerConfig).Assembly.GetName().Name)); }
其中 Combine 方法的第 2 个参数是项目中存放 xml 描述文件的位置,第 3 个参数即以程序集名称作为文件名,与项目属性中配置一致。
如遇到以下错误,请检查第 2、3、4 步骤中的配置(Debug / Release)
500 : {"Message":"An error has occurred."} /swagger/docs/v1
使枚举类型按实际文本作为参数值(而非转成索引数字)
打开文件:App_Start/SwaggerConfig.cs
取消注释:c.DescribeAllEnumsAsStrings();
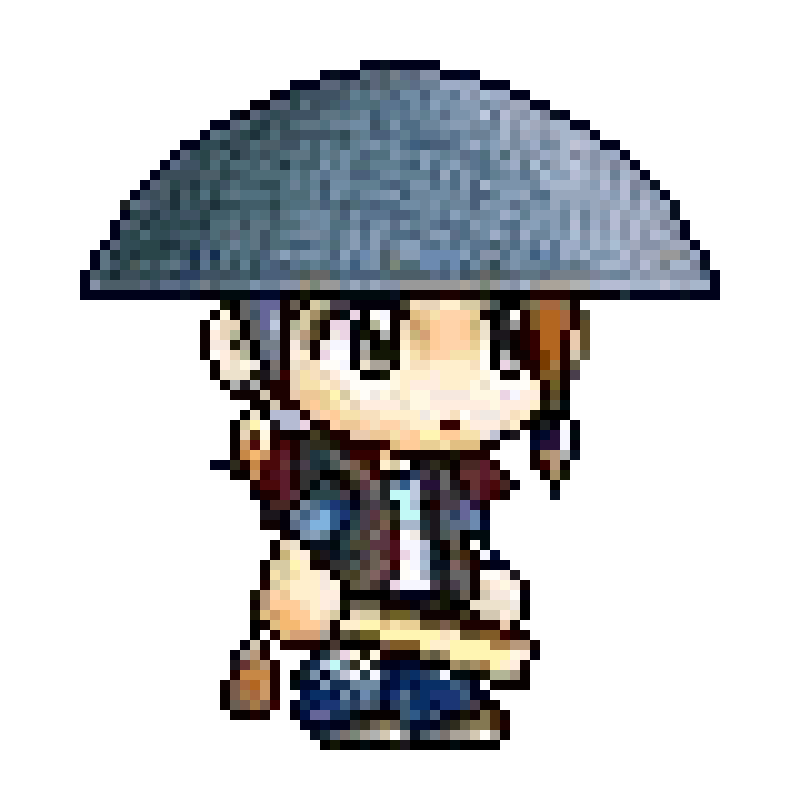
变量名 | 字段名 | 官方描述 |
return_code | 返回状态码 | SUCCESS/FAIL 此字段是通信标识,非交易标识,交易是否成功需要查看 trade_state 来判断 在“统一下单”和“支付结果通知”中,该描述变成了:交易是否成功需要查看 result_code 来判断 |
result_code | 业务结果 | SUCCESS/FAIL |
trade_state | 交易状态 | SUCCESS — 支付成功 REFUND — 转入退款 NOTPAY — 未支付 CLOSED — 已关闭 REVOKED — 已撤销(付款码支付) USERPAYING — 用户支付中(付款码支付) PAYERROR — 支付失败(其他原因,如银行返回失败) |
总的来说,
return_code 是用来判断通信状态的,个人理解在“结果通知”时必为 SUCCESS;
result_code 是用来判断业务结果的,指一次调用接口或回调的动作是否如愿执行成功。如“关闭订单”时关闭成功为 SUCCESS,因参数配置错误、找不到订单号、订单状态不允许关闭等其它关闭失败的情况为 FAIL;
trade_state 是用来判断交易状态的,“交易”是指微信支付订单。
另,在“统一下单”和“支付结果通知”中,return_code 的描述变成了:交易是否成功需要查看 result_code 来判断。不知道是官方笔误,还是真的可以用来判断交易是否成功,因为在调用“统一下单”时是未支付状态,根本没有支付成功的可能。
保险起见,我们在异步收到“结果通知”时,不要相信文档去判断 result_code,应调用“查询订单”,并判断 trade_state。
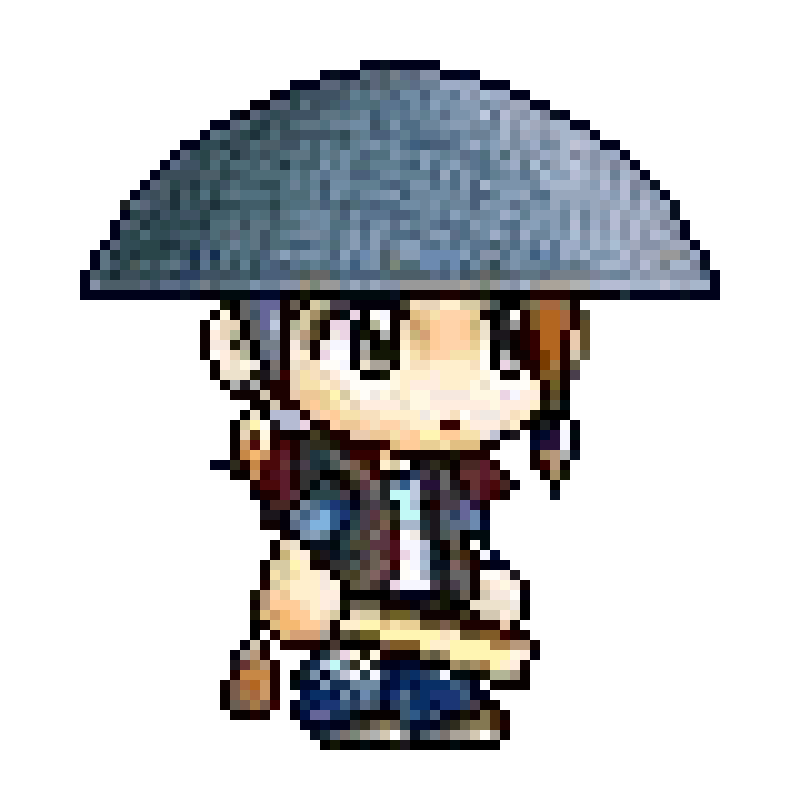
HTTP Error 502.5 - ANCM Out-Of-Process Startup Failure
Common causes of this issue:
The application process failed to start
The application process started but then stopped
The application process started but failed to listen on the configured port
Troubleshooting steps:
Check the system event log for error messages
Enable logging the application process' stdout messages
Attach a debugger to the application process and inspect
For more information visit: https://go.microsoft.com/fwlink/?LinkID=808681
发现装错了东西,服务器上应该安装 dotnet-hosting-*.*.*-win.exe
,而非 dotnet-runtime-*.*.*-win-x64.exe
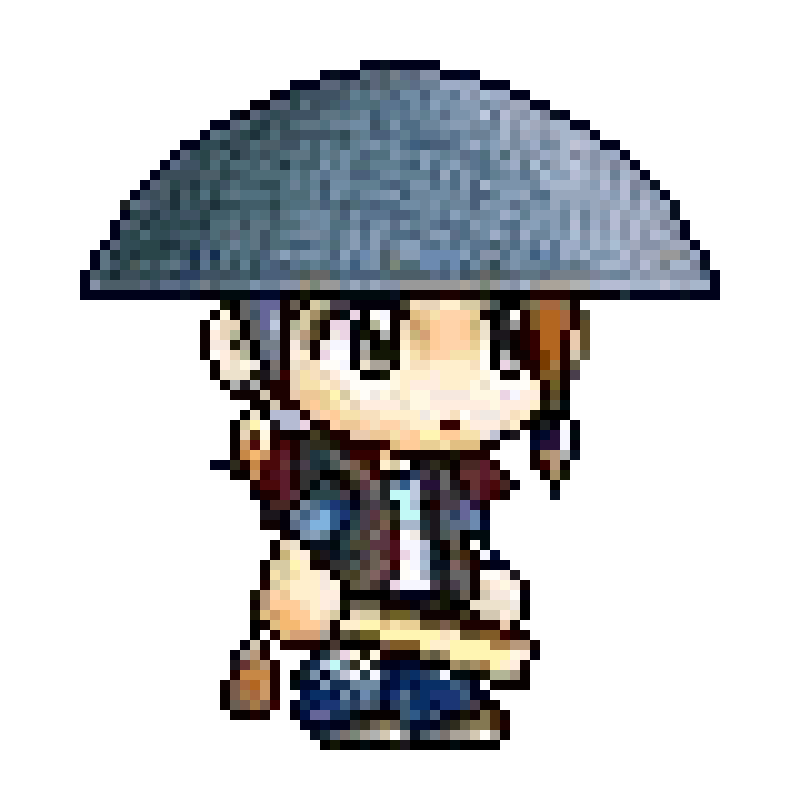
使用以下代码对字符串 s 进行判断:
var s = null;
if (s) {
console.log('true');
} else {
console.log('false');
}
测试当 s 为不同值时的结果如下:
s 的值 | if(s) 的结果 |
s = null; | false |
s = undefined; | false |
s = ''; | false |
s = ""; | false |
s = 0; | false |
s = 1; | true |
s = 2; | true |
s = -1; | true |
s = '0'; | true |
s= ' '; | true |
结论:
当 s 未定义或未赋值时,结果为 false;
当 s 为字符串类型时,长度为 0 即 false,长度大于 0 即 true;
当 s 为数字类型时,等于 0 为 false,不等于 0 为 true。
如果判断 s 的长度:
var s = null;
if (s.length > 0) {
console.log('true');
} else {
console.log('false');
}
那么会有以下结果:
s 的值 | if(s.length > 0) 的结果 |
s = null; | Uncaught TypeError |
s = undefined; | Uncaught TypeError |
s = ''; | false |
s = ""; | false |
s = 0; | false |
s = 1; | false |
s = 2; | false |
s = -1; | false |
s = '0'; | true |
s= ' '; | true |
结论:
当 s 未定义或未赋值时,异常;
当 s 为字符串类型时,长度为 0 即 false,长度大于 0 即 true;
当 s 为数字类型时,永远为 false。
这样的话,if(s.length > 0) 等同于 if(s.length)。
综上所述,判断一个字符串是否“有值且长度大于 0 ”,可以这样写:
var s = null;
if (s && s.length) {
console.log('true');
} else {
console.log('false');
}
相反,是否“无值或长度为 0”,可以这样写:
var s = null;
if (!s || !s.length) {
console.log('true');
} else {
console.log('false');
}
当然,如果要将不为 0 的数字类型也视为“有值”,只要 if(s) 或 if(!s) 即可。
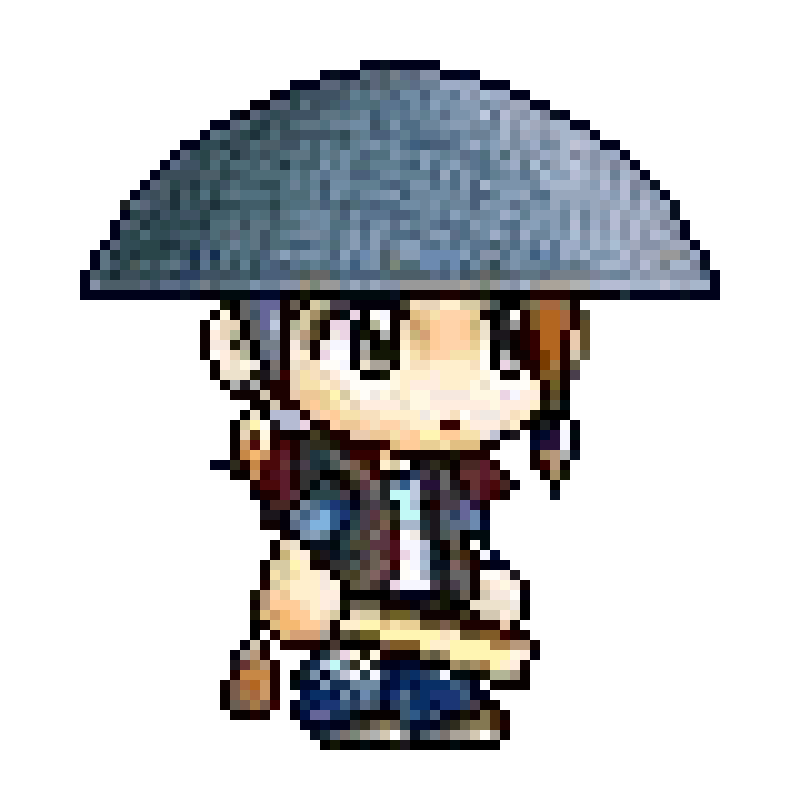
一、显示信息的命令
console.log("normal"); // 用于输出普通信息 console.info("information"); // 用于输出提示性信息 console.error("error"); // 用于输出错误信息 console.warn("warn"); // 用于输出警示信息
二、点位符:字符(%s)、整数(%d或%i)、浮点数(%f)和对象(%o);
console.log("%s","string"); //字符(%s) console.log("%d年%d月%d日",2016,8,29); //整数(%d或%i) console.log("圆周率是%f",3.1415926); //浮点数(%f) var dog = {}; dog.name = "大毛"; dog.color = "黄色"; dog.sex = "母狗"; console.log("%o",dog); //对象(%o)
三、信息分组 (console.group(),console.groupEnd())
console.group("第一组信息"); console.log("第一组第一条:我的博客"); console.log("第一组第二条:CSDN"); console.groupEnd(); console.group("第二组信息"); console.log("第二组第一条:程序爱好者QQ群"); console.log("第二组第二条:欢迎你加入"); console.groupEnd();
四、将对象以树状结构展现 (console.dir()可以显示一个对象所有的属性和方法)
var info = { name : "Alan", age : "27", grilFriend : "nothing", getName : function(){ return this.name; } } console.dir(info);
五、显示某个节点的内容 (console.dirxml()用来显示网页的某个节点(node)所包含的html/xml代码)
var node = document.getElementById("info"); node.innerHTML += "<p>追加的元素显示吗</p>"; console.dirxml(node);
六、判断变量是否是真 (console.assert()用来判断一个表达式或变量是否为真,只有表达式为false时,才输出一条相应作息,并且抛出一个异常)
var testObj = false; console.assert(testObj, '当testObj为false时才输出!');
七、计时功能 (console.time()和console.timeEnd(),用来显示代码的运行时间)
console.time("控制台计时器"); for(var i = 0; i < 10000; i++){ for(var j = 0; j < 10000; j++){} } console.timeEnd("控制台计时器");
八、性能分析performance profile (就是分析程序各个部分的运行时间,找出瓶颈所在,使用的方法是console.profile()和console.proileEnd();)
function All(){ // alert(11); for(var i = 0; i < 10; i++){ funcA(100); } funcB(1000); } function funcA(count){ for(var i = 0; i < count; i++){}; } function funcB(count){ for(var i = 0; i < count; i++){}; } console.profile("性能分析器"); All(); console.profileEnd();
详细的信息在chrome控制台里的"profile"选项里查看
九、console.count()统计代码被执行的次数
function myFunction(){ console.count("myFunction 被执行的次数"); } myFunction(); //myFunction 被执行的次数: 1 myFunction(); //myFunction 被执行的次数: 2 myFunction(); //myFunction 被执行的次数: 3
十、keys和values,要在浏览器里输入
十一、console.table表格显示方法
var mytable = [ { name: "Alan", sex : "man", age : "27" }, { name: "Wu", sex : "gril", age : "28" }, { name: "Tao", sex : "man and gril", age : "29" } ] console.table(mytable);
十二、Chrome 控制台中原生支持类jQuery的选择器,也就是说你可以用$加上熟悉的css选择器来选择DOM节。
$("body"); //选择body节点
十三、copy通过此命令可以将在控制台获取到的内容复制到剪贴板
copy(document.body); //复制body copy(document.getElementById("info")); //复制某id元素的的节点
十四、$_命令返回最近一次表达式执行的结果,$0-$4代表了最近5个你选择过的DOM节点
十五、利用控制台输出文字,图片,以%c开头,后面的文字就打印的信息,后面一个参数就是样式属性;
console.log("请在邮件中注明%c 来自:console","font-size:16px;color:red;font-weight:bold;");
在学习和使用 ASP.NET Web API 之前,最好先对 RESTful 有所了解。它是一种软件架构风格、设计风格,而不是标准。
推荐视频教程:https://www.imooc.com/learn/811
讲师:会飞的鱼Xia
需时:2小时25分
REST API 测试工具:
在 Chrome 网上应用店中搜索:Restlet Client
或网站 https://client.restlet.com
>>> 资源路径
SCHEME :// HOST [ ":" PORT ] [ PATH [ "?" QUERY ]]
其中 PATH 中的资源名称应该使用复数名词,举例:
GET https://xoyozo.net/api/Articles/{id}
POST https://xoyozo.net/api/Articles
>>> HTTP verb(对应 CURD 操作):
方法 | 功能 | ASP.NET Web API 接口返回类型(一般的) |
---|---|---|
GET | 取一个或多个资源 | T 或 IEnumerable<T> |
POST | 增加一个资源 | T |
PUT | 修改一个资源 | T |
DELETE | 删除一个资源 | void |
PATCH | 更新一个资源(资源的部分属性) | |
HEAD | 获取报头 | |
OPTIONS | 查询服务器性能或资源相关选项和需求 |
>>> 过滤信息:
如分页、搜索等
>>> 常用状态码:
200 | OK | 指示请求已成功 |
201 | Created | 资源创建成功(常见用例是一个 PUT 请求的结果) |
202 | Accepted | 该请求已被接收但尚未起作用。它是非承诺的,这意味着HTTP中没有办法稍后发送指示处理请求结果的异步响应。 |
204 | No Content | 成功 PUT(修改)或 DELETE(删除)一个资源后返回空内容(ASP.NET Web API 中将接口返回类型设置为 void 即可) |
400 | Bad Request | 服务器无法理解请求(如必填项未填、内容超长、分页大小超限等),幂等 |
401 | Unauthorized | 未授权(如用户未登录时执行了须要登录才能执行的操作),通俗地讲,是服务器告诉你,“你没有通过身份验证 - 根本没有进行身份验证或验证不正确 - 但请重新进行身份验证并再试一次。”这是暂时的,服务器要求你在获得授权后再试一次。 |
403 | Forbidden | 服务器理解请求但拒绝授权(是永久禁止的并且与应用程序逻辑相关联(如不正确的密码、尝试删除其它用户发表的文章)、IP 被禁止等),通俗地讲,是服务器告诉你,“我很抱歉。我知道你是谁 - 我相信你说你是谁 - 但你只是没有权限访问此资源。” |
404 | Not Found | 找不到请求的资源(指资源不存在,如果找到但无权访问则应返回 403) |
405 | Method Not Allowed | 请求方法被禁用(HTTP 动词) |
500 | Internal Server Error | 服务器遇到阻止它履行请求的意外情况(如数据库连接失败) |
503 | Service Unavailable | 服务器尚未准备好处理请求(常见原因是服务器因维护或重载而停机,这是临时的,可用于在单线程处理事务时遇到被锁定时的响应,如抽奖活动、抢楼活动,防止因并发导致逻辑错误) |
>> 错误处理:
C# 例:throw new HttpResponseException(HttpStatusCode.NotFound);
PHP 例:throw new Exception('文章不存在', 404);
>>> 返回结果:
JSON/XML
不要返回密码等机密字段
>>> 其它:
身份验证窗口(浏览器弹窗,类似 alert,非页面表单),明文传输,安全性不高,不建议使用。实现:
在 Headers 中添加 Authorization:Basic “用户名:密码”的 Base64 编码
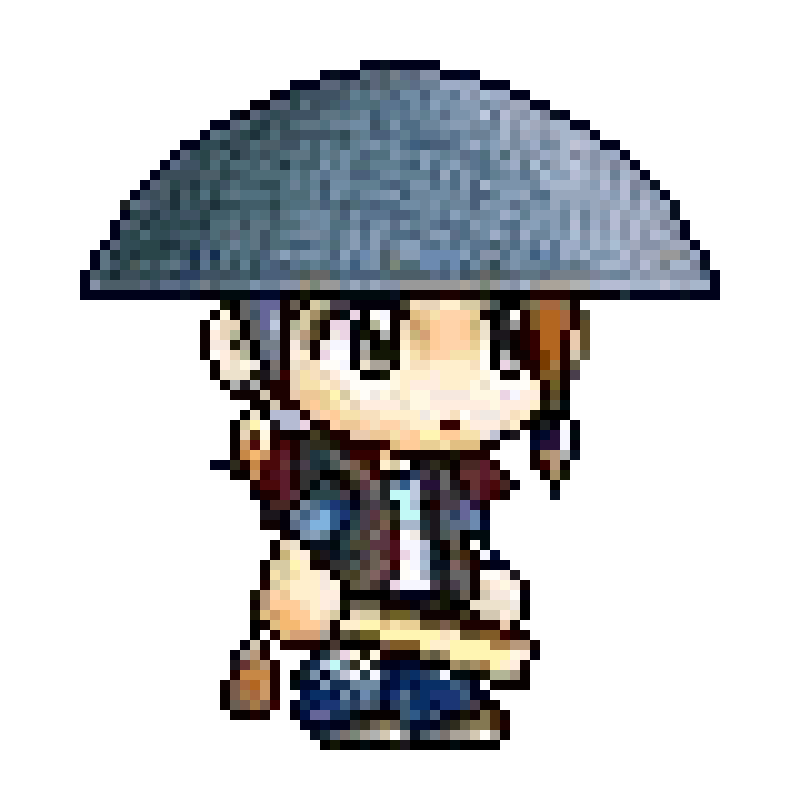
本文不定时更新中……
收集了一些在开发过程中遇到的一些问题的解决方法,适合新手。
异常:
出现脚本错误或者未正确调用 Page()
原因:不小心删了第一行内容:<template>
异常:
模块编译失败:TypeError: Cannot read property 'for' of undefined
at fixDefaultIterator (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4277:24)
at mark (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4306:5)
at markComponent (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4371:5)
at baseCompile (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4384:15)
at compile (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4089:28)
at Object.module.exports (D:\HBuilderX\plugins\uniapp\lib\mpvue-loader\lib\template-compiler\index.js:43:18)
原因:新建的页面(简单模板)只有以下 3 个标签,须在 <template /> 中添加一些代码,如 <view />
<template>
</template>
<script>
</script>
<style>
</style>
异常:
模块编译失败:TypeError: Cannot read property 'toString' of undefined
at Object.preprocess (D:\HBuilderX\plugins\uniapp\lib\preprocess\lib\preprocess.js:56:15)
at Object.module.exports (D:\HBuilderX\plugins\uniapp\lib\preprocessor-loader.js:9:25)
原因:没有原因,纯抽风,HX 关掉再开就好了。
异常:
Cannot set property 'xxx' of undefined;at pages/... onLoad function;at api request success callback function
原因:属性未定义,例如
data() {
return {
item: { }
}
}
而直接赋值 this.item.abc.xxx = '123';
解决:
data() {
return {
item: {
abc: ''
}
}
}
问:page 页面怎样修改 tabBar?
答:官方文档未给出答案,百度了一圈也无果(2018-10-23),但有人说小程序的 setTabBarBadge() 方法设置角标是可以用的。
坑:
VM1694:1 获取 wx.getUserInfo 接口后续将不再出现授权弹窗,请注意升级
参考文档: https://developers.weixin.qq.com/community/develop/doc/0000a26e1aca6012e896a517556c01
填坑:放弃使用 uni.getUserInfo 接口来获取用户信息,uni.login 可返回用于换取 openid / unionid 的 code,参:uni.login、 code2Session
坑:字符搜索(当前目录)(Ctrl+Alt+F)搜不出所有结果
填坑:顾名思义他只搜索当前目录,即当前打开文件所在目录,而非我误认为的整个项目根目录。在“项目管理器”中选中要搜索字符的目录即可。
坑:uni.navigateTo() 或 uni.redirectTo() 没反应
填坑:这两个方法不允许跳转到 tabbar 页面,用 uni.switchTab() 代替。
坑:使用“Ctrl+/”快捷键弹出“QQ五笔小字典”窗口
解决:打开QQ五笔“属性设置”,切换到“快捷键设置”选项卡,把“五笔小字典”前的勾取消(即使该组合键是设置为Ctrl+?)。
坑:<rich-text /> 中的 <img /> 太大,超出屏幕宽度
填坑:用正则表达式给 <img /> 加上最大宽度
data.data.Content = data.data.Content.replace(/\<img/gi, '<img style="max-width:100%;height:auto" ');
坑:无法重命名或删除目录或文件
填坑一:“以管理员身份运行”HBuilder X 后再试。
填坑二:关闭微信开发者工具、各种手机和模拟器后再试。
填坑三:打开“任务管理器”,结束所有“node.exe”进程后再试。
坑:
thirdScriptError
sdk uncaught third Error
(intermediate value).$mount is not a function
TypeError: (intermediate value).$mount is not a function
Page[pages/xxxx/xxxx] not found. May be caused by: 1. Forgot to add page route in app.json. 2. Invoking Page() in async task.
Page is not constructed because it is not found.
填坑:关闭微信开发者工具、各种手机和模拟器后,删除“unpackage”目录。
坑:
Unexpected end of JSON input;at "pages/news/view" page lifeCycleMethod onLoad function
SyntaxError: Unexpected end of JSON input
填坑:给 uni.navigateTo() 的 url 传参时,如果简单地将对象序列化 JSON.stringify(item),那么如果内容中包含“=”等 url 特殊字符,就会发生在接收页面 onLoad() 中无法获取到完整的 json 对象,发生异常。
uni.navigateTo({
url: "../news/view?item=" + JSON.stringify(item)
})
所以应该把参数值编码:
uni.navigateTo({
url: "../news/view?item=" + escape(JSON.stringify(item))
})
如果是一般的 web 服务器来接收,那么会自动对参数进行解码,但 uni-app 不会,如果直接使用:
onLoad(e) {
this.item = JSON.parse(e.item);
}
会发生异常:
Unexpected token % in JSON at position 0;at "pages/news/view" page lifeCycleMethod onLoad function
SyntaxError: Unexpected token % in JSON at position 0
需要解码一次:
onLoad(e) {
this.item = JSON.parse(unescape(e.item));
}
需要注意的是,unescape(undefined) 会变成 'undefined',如果要判断是否 undefined,应是 unescape 之前。
坑:图片变形
填坑:mode="widthFix"
坑:页面如何向 tabBar 传参
填坑:全局或缓存
坑:编译为 H5 后,出现:Access-Control-Allow-Origin
填坑:参阅
坑:编译为 H5 后,GET 请求的 URL 中出现“?&”
填坑 :客户端只求 DCloud 官方能够尽快修复这个 bug,IIS 端可以暂时用 URL 重写来防止报 400 错误,参此文。
坑:[system] errorHandler TypeError: Cannot read property 'forEach' of undefined
填坑:待填
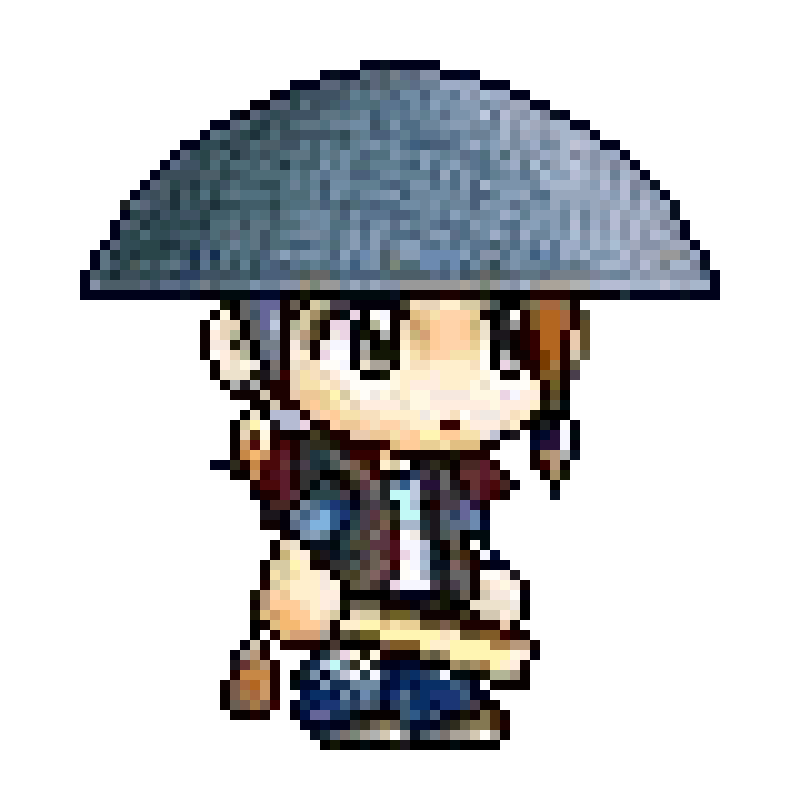
制作类似下图中的拖拽排序功能:
1. 首先数据库该表中添加字段 sort,类型为 double(MySQL 中为 double(0, 0))。
2. 页面输出绑定数据(以 ASP.NET MVC 控制器为例):
public ActionResult EditSort()
{
if (!zConsole.Logined) { return RedirectToAction("", "SignIn", new { redirect = Request.Url.OriginalString }); }
db_auto2018Entities db = new db_auto2018Entities();
return View(db.dt_dealer.Where(c => c.enabled).OrderBy(c => c.sort));
}
这里可以加条件列出,即示例中 enabled == true 的数据。
3. 前台页面引用 jQuery 和 jQuery UI。
4. 使用 <ul /> 列出数据:
<ul id="sortable" class="list-group gutter list-group-lg list-group-sp">
@foreach (var d in Model)
{
<li class="list-group-item" draggable="true" data-id="@d.id">
<span class="pull-left"><i class="fa fa-sort text-muted fa m-r-sm"></i> </span>
<div class="clear">
【id=@d.id】@d.name_full
</div>
</li>
}
</ul>
5. 初始化 sortable,当拖拽结束时保存次序:
<script>
var url_SaveSort = '@Url.Action("SaveSort")';
</script>
<script>
$("#sortable").sortable({
stop: function (event, ui) {
// console.log('index: ' + $(ui.item).index())
// console.log('id: ' + $(ui.item).data('id'))
// console.log('prev_id: ' + $(ui.item).prev().data('id'))
$.post(url_SaveSort, {
id: $(ui.item).data('id'),
prev_id: $(ui.item).prev().data('id')
}, function (json) {
if (json.result.success) {
// window.location.reload();
} else {
toastr["error"](json.result.info);
}
}, 'json');
}
});
$("#sortable").disableSelection();
</script>
这里回传到服务端的参数为:当前项的 id 值、拖拽后其前面一项的 prev_id 值(若移至首项则 prev_id 为 undefined)。
不使用 $(ui.item).index() 是因为,在有筛选条件的结果集中排序时,使用该索引值配合 LINQ 的 .Skip 会引起取值错误。
6. 控制器接收并保存至数据库:
[HttpPost]
public ActionResult SaveSort(int id, int? prev_id)
{
if (!zConsole.Logined)
{
return Json(new { result = new { success = false, msg = "请登录后重试!" } }, JsonRequestBehavior.AllowGet);
}
db_auto2018Entities db = new db_auto2018Entities();
dt_dealer d = db.dt_dealer.Find(id);
// 拖拽后其前项 sort 值(若无则 null)(此处不需要加 enabled 等筛选条件)
double? prev_sort = prev_id.HasValue
? db.dt_dealer.Where(c => c.id == prev_id).Select(c => c.sort).Single()
: null as double?;
// 拖拽后其后项 sort 值(若无前项则取首项作为后项)(必须强制转化为 double?,否则无后项时会返回 0,导致逻辑错误)
double? next_sort = prev_id.HasValue
? db.dt_dealer.Where(c => c.sort > prev_sort && c.id != id).OrderBy(c => c.sort).Select(c => (double?)c.sort).FirstOrDefault()
: db.dt_dealer.Where(c => c.id != id).OrderBy(c => c.sort).Select(c => (double?)c.sort).FirstOrDefault();
if (prev_sort.HasValue && next_sort.HasValue)
{
d.sort = (prev_sort.Value + next_sort.Value) / 2;
}
if (prev_sort == null && next_sort.HasValue)
{
d.sort = next_sort.Value - 1;
}
if (prev_sort.HasValue && next_sort == null)
{
d.sort = prev_sort.Value + 1;
}
db.SaveChanges();
return Json(new { item = new { id = d.id }, result = new { success = true } });
}
需要注意的是,当往数据库添加新项时,必须将 sort 值设置为已存在的最大 sort 值 +1 或最小 sort 值 -1。
var d = new dt_dealer
{
name_full = "新建项",
sort = (db.dt_dealer.Max(c => (double?)c.sort) ?? 0) + 1,
};
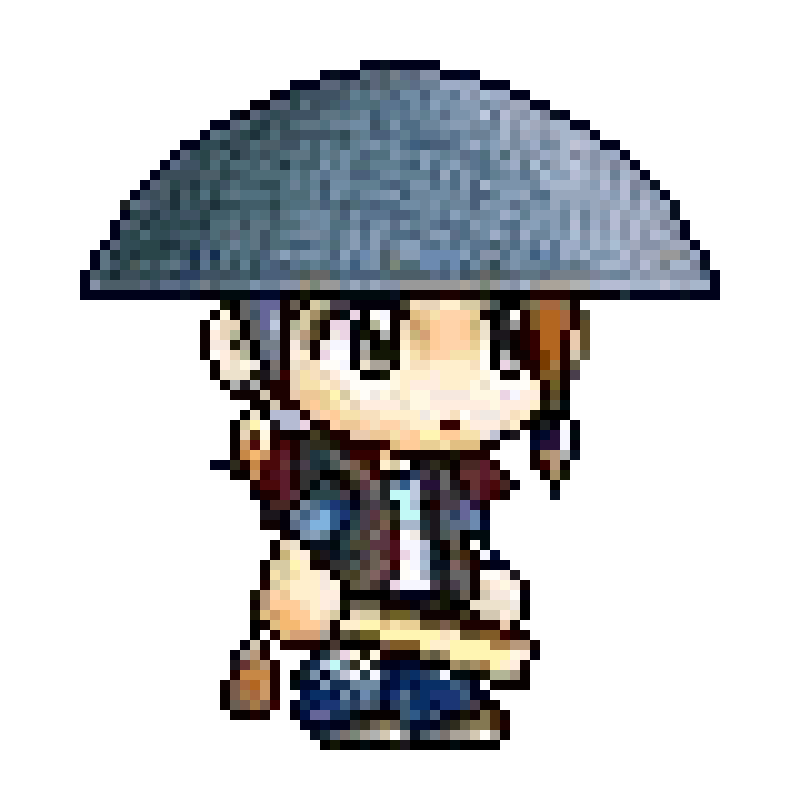
当我们初始化一个 Swiper 时,
var mySwiper = new Swiper ('.swiper-container');
如果页面上只有一个 .swiper-container,那么 mySwiper 是一个对象,如果有多个 .swiper-container,则 mySwiper 是一个数组。
处理不当会报以下错误:
Uncaught TypeError: Cannot read property 'appendSlide' of undefined
Uncaught TypeError: Cannot read property 'prependSlide' of undefined
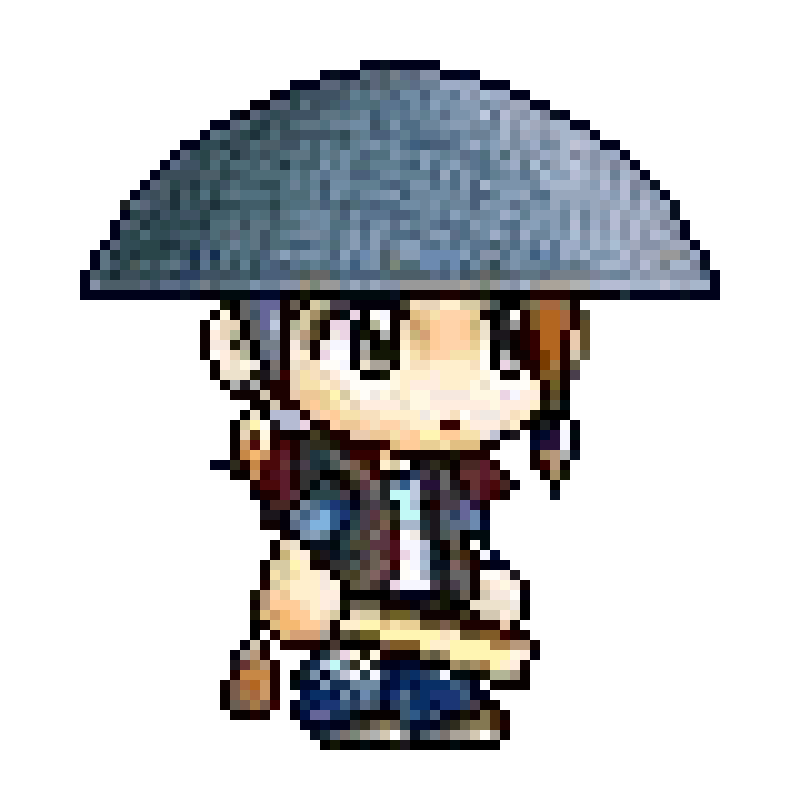
如果 .NET Core 项目发布到服务器上报以下错误:
HTTP Error 502.5 - Process Failure
Common causes of this issue:
The application process failed to start
The application process started but then stopped
The application process started but failed to listen on the configured port
Troubleshooting steps:
Check the system event log for error messages
Enable logging the application process' stdout messages
Attach a debugger to the application process and inspect
For more information visit: https://go.microsoft.com/fwlink/?LinkID=808681
在服务器上安装最新的 .NET Core Runtime 即可(Windows Server 选择 Hosting Bundle Installer)。
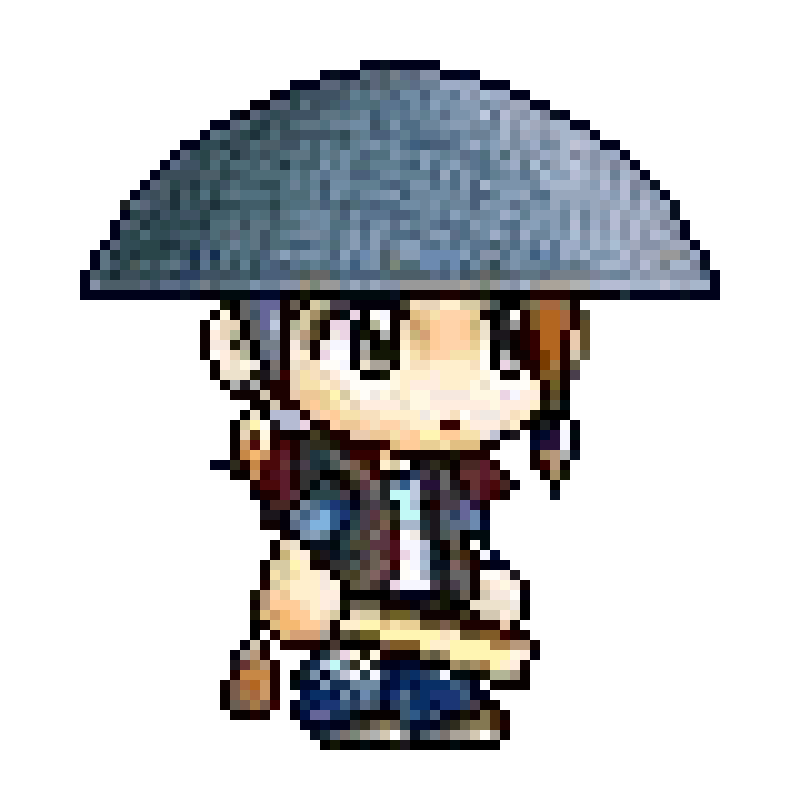