前端引用 jquery、jquery-ui(或 jquery.ui.widget),以及 jquery.fileupload.js
$('#xxx').fileupload({
url: 'FileUpload',
add: function (e, data) {
console.log('add', data);
data.submit();
},
success: function (response, status) {
console.log('success', response);
toastr.success('上传成功!文件名:' + response);
},
error: function (error) {
console.log('error', error);
toastr.error("上传失败:" + error.responseText);
}
});
服务端接收文件:
[HttpPost]
public ActionResult FileUpload([FromForm(Name = "files[]")] IFormFile file)
{
try
{
return Ok(file.FileName);
}
catch(Exception ex)
{
return BadRequest(ex.Message);
}
}
此处 Name = "files[]" 值是由 <input type="file" name="这里定的" />
如果 input 没有指定 name,那么一般为 file[],或者在 Chrome 的开发者工具中,切换到 Network 标签页,选中 post 的目标请求,在 Headers 的 Form Data 中可以看到 name="xxx"。
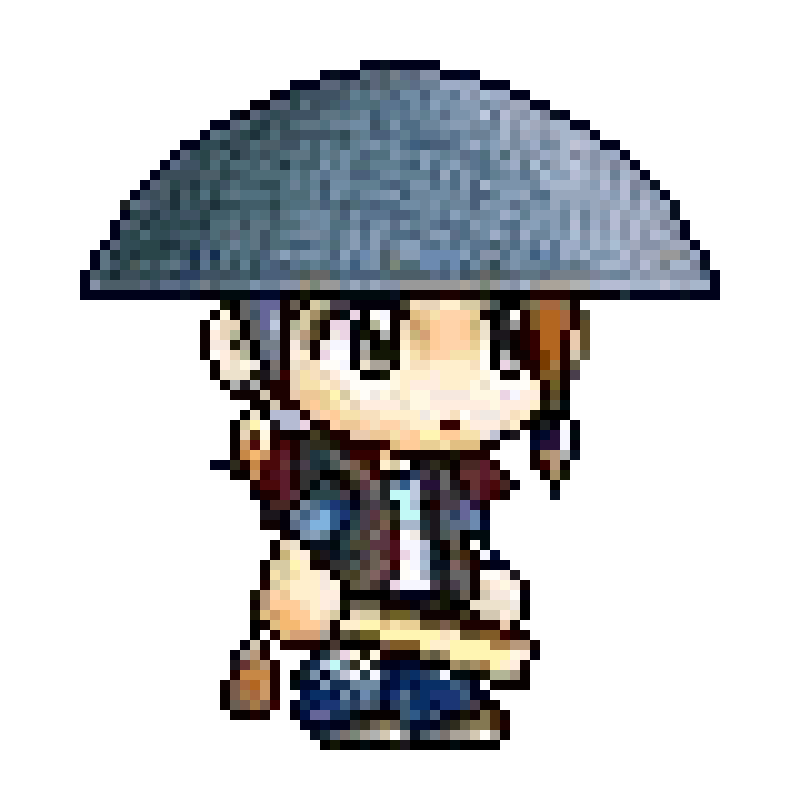
ASP.NET MVC 使用 Authorize 过滤器验证用户登录。Authorize 过滤器首先运行在任何其它过滤器或动作方法之前,主要用来做登录验证或者权限验证。
示例:使用 Authorize 过滤器实现简单的用户登录验证。
1、创建登录控制器 LoginController
/// <summary>
/// 登录控制器
/// </summary>
[AllowAnonymous]
public class LoginController : Controller
{
/// <summary>
/// 登录页面
/// </summary>
public ActionResult Index()
{
return View();
}
/// <summary>
/// 登录
/// </summary>
[HttpPost]
public ActionResult Login(string loginName, string loginPwd)
{
if (loginName == "admin" && loginPwd == "123456")
{
// 登录成功
Session["LoginName"] = loginName;
return RedirectToAction("Index", "Home");
}
else
{
// 登录失败
return RedirectToAction("Index", "Login");
}
}
/// <summary>
/// 注销
/// </summary>
public ActionResult Logout()
{
Session.Abandon();
return RedirectToAction("Index", "Login");
}
}
注意:在登录控制器 LoginController 上添加 AllowAnonymous 特性,该特性用于标记在授权期间要跳过 AuthorizeAttribute 的控制器和操作。
2、创建登录页面
@{
ViewBag.Title = "登录页面";
Layout = null;
}
<h2>登录页面</h2>
<form action='@Url.Action("Login","Login")' id="form1" method="post">
用户:<input type="text" name="loginName" /><br />
密码:<input type="password" name="loginPwd" /><br />
<input type="submit" value="登录">
</form>
效果图:
3、创建主页控制器 LoginController
public class HomeController : Controller
{
public ActionResult Index()
{
// 获取当前登录用户
string loginName = Session["LoginName"].ToString();
ViewBag.Message = "当前登录用户:" + loginName;
return View();
}
}
4、创建主页页面
@{
ViewBag.Title = "Index";
Layout = null;
}
<h2>Index</h2>
<h3>@ViewBag.Message</h3>
<a href="@Url.Action("Logout","Login")">注销</a>
效果图:
5、创建授权过滤器 LoginAuthorizeAttribute 类
创建 Filter 目录,在该目录下创建授权过滤器 LoginAuthorizeAttribute 类,继承 AuthorizeAttribute。
using System.Web.Mvc;
namespace MvcApp.Filter
{
/// <summary>
/// 授权过滤器
/// </summary>
public class LoginAuthorizeAttribute : AuthorizeAttribute
{
public override void OnAuthorization(AuthorizationContext filterContext)
{
// 判断是否跳过授权过滤器
if (filterContext.ActionDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true)
|| filterContext.ActionDescriptor.ControllerDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true))
{
return;
}
// 判断登录情况
if (filterContext.HttpContext.Session["LoginName"] == null || filterContext.HttpContext.Session["LoginName"].ToString()=="")
{
//HttpContext.Current.Response.Write("认证不通过");
//HttpContext.Current.Response.End();
filterContext.Result = new RedirectResult("/Login/Index");
}
}
}
}
通常 Authorize 过滤器也是在全局过滤器上面的,在 App_Start 目录下的 FilterConfig 类的 RegisterGlobalFilters 方法中添加:
using System.Web;
using System.Web.Mvc;
using MvcApp.Filter;
namespace MvcApp
{
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
// 添加全局授权过滤器
filters.Add(new LoginAuthorizeAttribute());
}
}
}
Global.asax 下的代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Optimization;
using System.Web.Routing;
namespace MvcApp
{
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
}
}
}
xoyozo:暂时没有按区域(Area)来统一配置的方法,建议加在 Controller 上。https://stackoverflow.com/questions/2319157/how-can-we-set-authorization-for-a-whole-area-in-asp-net-mvc
form 提交 checkbox
<form method="get" asp-action="Index">
<input type="checkbox" name="a" value="1" /> 一
<input type="checkbox" name="a" value="2" /> 二
</form>
get 方式的 url 中会出现多个相同名称的参数
/abc?a=1&a=2
C# 使用数组类型接收参数
public IActionResult Index(string[] a)
{
}
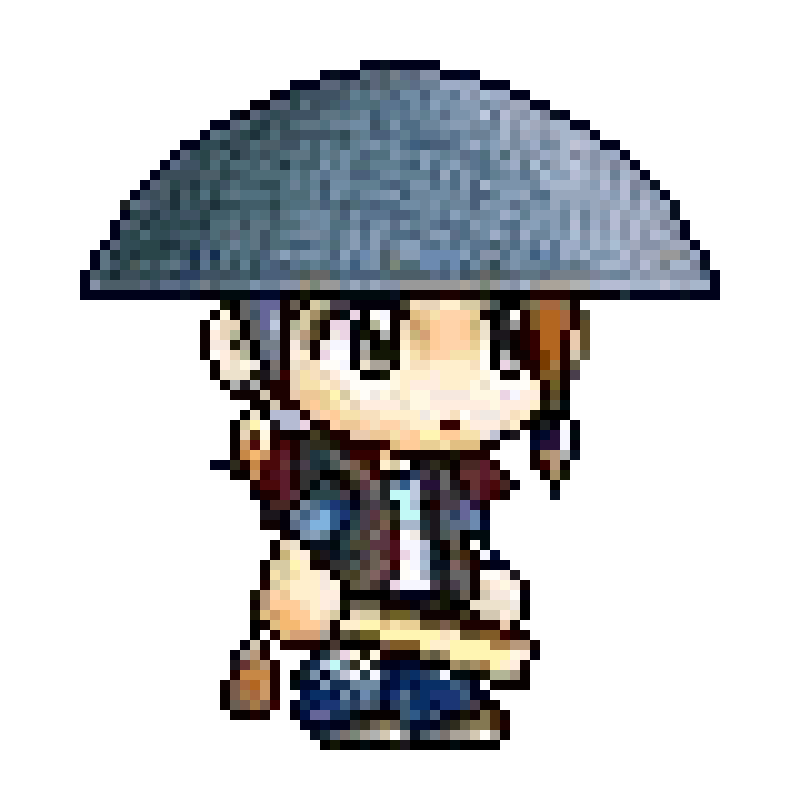
Unify Template 提供三种文件上传样式(如上图),“Plain File Input”和“File input”使用传统 <form /> 提交,“Advanced File input”调用组件实现。
ASP.NET Core 实现传统 <form /> 提交(以“Plain File Input”为例):
<form class="g-brd-around g-brd-gray-light-v4 g-pa-30 g-mb-30" id="form_avatar_upload" asp-action="AvatarUpload" enctype="multipart/form-data" method="post">
<!-- Plain File Input -->
<div class="form-group mb-0">
<p>Plain File Input</p>
<label class="u-file-attach-v2 g-color-gray-dark-v5 mb-0">
<input id="fileAttachment" name="Avatar" type="file" onchange="form_avatar_upload.submit()">
<i class="icon-cloud-upload g-font-size-16 g-pos-rel g-top-2 g-mr-5"></i>
<span class="js-value">Attach file</span>
</label>
</div>
<!-- End Plain File Input -->
</form>
public class AvatarUploadModel
{
public IFormFile Avatar { get; set; }
}
[HttpPost]
public IActionResult AvatarUpload(AvatarUploadModel Item)
{
return RedirectToAction("");
}
ASP.NET Core 调用 HSFileAttachment 组件实现:
<div class="form-group mb-20">
<label class="g-mb-10">上传作品文件(包)</label>
<input class="js-file-attachment_project" type="file" name="" id="file_project">
<div style="display: none;" id="file_project_tip">
<div class="u-file-attach-v3 g-mb-15" id="file_project_tip_content">
<h3 class="g-font-size-16 g-color-gray-dark-v2 mb-0">拖曳文件到此处或者 <span class="g-color-primary">浏览你的电脑</span></h3>
<p class="g-font-size-14 g-color-gray-light-v2 mb-0">
如果您的作品包含多个文件,请添加到压缩包再上传
</p>
<p class="g-font-size-14 g-color-gray-light-v2 mb-0">
支持的文件格式:
<code>zip</code>
<code>rar</code>
<code>7z</code>
<code>psd</code>
<code>ai</code>
<code>cdr</code>
<code>jpg</code>
<code>png</code>
<code>gif</code>
<code>tif</code>
<code>webp</code>
</p>
<p class="g-font-size-14 g-color-gray-light-v2 mb-0">文件(包)的大小不能超过 <span>@options.Value.MaxSizeOfSigleFile_MB</span> MB</p>
</div>
</div>
<small class="form-control-feedback">请上传作品文件</small>
</div>
<!-- JS Implementing Plugins -->
<script src="//cdn.***.com/unify/unify-v2.6.2/html/assets/vendor/jquery.filer/js/jquery.filer.min.js"></script>
<!-- JS Unify -->
<script src="//cdn.***.com/unify/unify-v2.6.2/html/assets/js/helpers/hs.focus-state.js"></script>
<script src="//cdn.***.com/unify/unify-v2.6.2/html/assets/js/components/hs.file-attachement.js"></script>
<!-- JS Plugins Init. -->
<script>
$(document).on('ready', function () {
// initialization of forms
$.HSCore.components.HSFileAttachment.init('.js-file-attachment_project', {
changeInput: $('#file_project_tip').html(),
uploadFile: {
url: url_UploadFile,
data: { type: 'project' },
success: function (data, element) {
console.log(data);
if (!data.success) {
var parent = element.find(".u-progress-bar-v1").parent();
element.find(".u-progress-bar-v1").fadeOut("slow", function () {
$("<div class=\"text-error g-px-10\"><i class=\"fa fa-minus-circle\"></i> Error</div>").hide().appendTo(parent).fadeIn("slow");
});
alert(data.err_msg);
} else {
var parent = element.find(".u-progress-bar-v1").parent();
element.find(".u-progress-bar-v1").fadeOut("slow", function () {
$("<div class=\"text-success g-px-10\"><i class=\"fa fa-check-circle\"></i> Success</div>").hide().appendTo(parent).fadeIn("slow");
});
$('#file_project_tip_content').slideUp();
upload_project_id = data.id;
}
}
}
});
$.HSCore.helpers.HSFocusState.init();
});
</script>
public IActionResult UploadFile([FromForm(Name = "[]")]IFormFile file, string type)
{
return Ok(new { id = 1 });
}
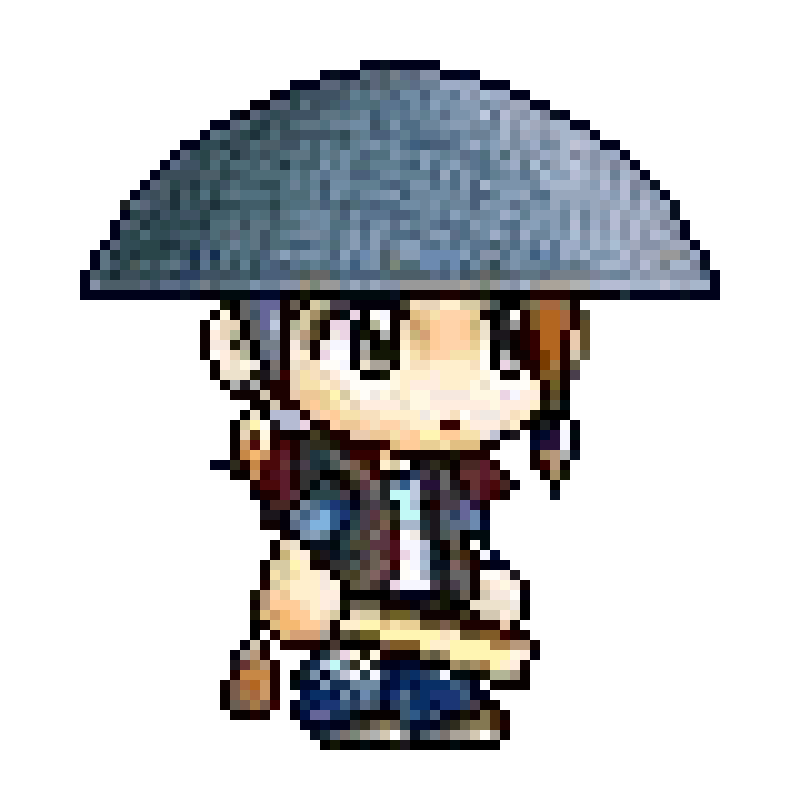
引用
jQuery、moment.js、daterangepicker
例子
$('.x_dates').daterangepicker({
"timePicker": false, // 是否显示时间
//"dateLimit": {
// "days": 7 // 可选中的最大区间(天)
//},
"ranges": { // 快捷栏
"今天": [moment(), moment()],
"昨天": [moment().subtract(1, 'days'), moment().subtract(1, 'days')],
"最近 7 天": [moment().subtract(6, 'days'), moment()],
"最近 30 天": [moment().subtract(29, 'days'), moment()],
"本月": [moment().startOf('month'), moment().endOf('month')],
"上个月": [moment().subtract(1, 'month').startOf('month'), moment().subtract(1, 'month').endOf('month')]
},
startDate: daterangepicker_startDate, // moment(),
endDate: daterangepicker_endDate, // moment(),
autoUpdateInput: true,
"locale": {
"direction": "ltr",
"format": "YYYY-MM-DD", // YYYY-MM-DD HH:mm
"separator": " 至 ",
"applyLabel": "确定",
"cancelLabel": "取消",
"fromLabel": "From",
"toLabel": "To",
"customRangeLabel": "自定义",
"daysOfWeek": ["日", "一", "二", "三", "四", "五", "六"],
"monthNames": ["一月", "二月", "三月", "四月", "五月", "六月", "七月", "八月", "九月", "十月", "十一月", "十二月"],
"firstDay": 1
}
}, function (start, end, label) {
//console.log('New date range selected: ' + start.format('YYYY-MM-DD') + ' to ' + end.format('YYYY-MM-DD') + ' (predefined range: ' + label + ')');
fn_daterangepicker_changed(start.format('YYYY-MM-DD'), end.format('YYYY-MM-DD'))
});
官网(含配置工具)
http://www.daterangepicker.com/
GitHub
https://github.com/dangrossman/daterangepicker
配置工具
下载的包中的 demo.html
Demo
https://awio.iljmp.com/5/drpdemo
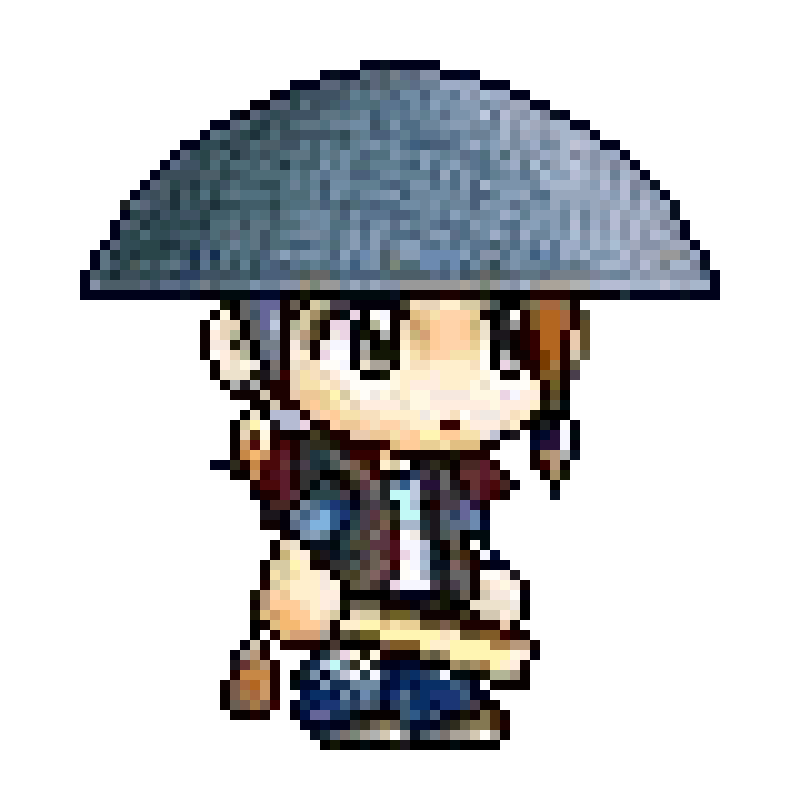
安装 Nuget 包:
项目根目录创建绑定配置文件:bundleconfig.json
示例:
[
{
"outputFileName": "wwwroot/css/site.min.css",
"inputFiles": [
"wwwroot/css/site.css",
"wwwroot/css/custom.css"
]
},
{
"outputFileName": "wwwroot/js/site.min.js",
"inputFiles": [
"wwwroot/js/site.js"
],
"minify": {
"enabled": true,
"renameLocals": true
},
"sourceMap": false
}
]
配置文件中所有路径都相对于项目根目录(而非静态文件根目录 wwwroot),因此配置的路径都要以“wwwroot”开头。
outputFileName 是压缩合并后的文件,inputFiles 是被压缩合并的原始文件集合。
对于 js 配置部分,minify.enabled 配置是否缩小,renameLocals 配置是否修改变量名,sourceMap 配置是否生成 map 映射文件。
引用示例:
<link rel="stylesheet" href="~/css/site.min.css" asp-append-version="true" />
asp-append-version 表示是否在引用路径添加版本参数,可实现在文件有修改时及时在客户端浏览器中生效。
* 注意:有时候“生成”不一定生效,“重新生成”肯定会生效。
更多高级用法请参考官方文档。
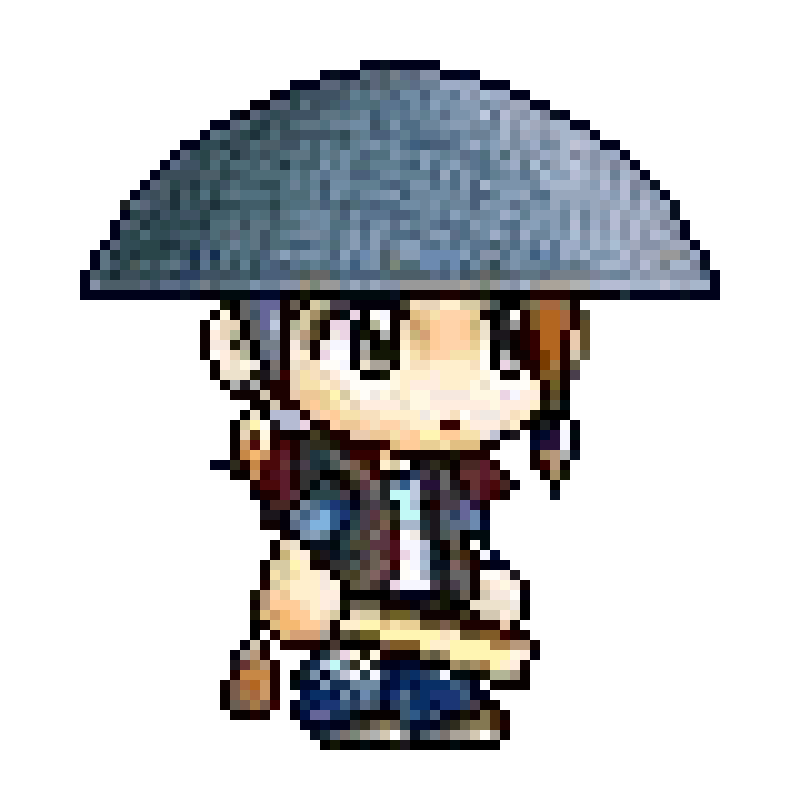
Input string is not a valid interger. Path 'batteryLevel', line 1, position 447.
使用 uni-app 开发小程序的时候,获取系统信息(getSystemInfoSync())在 iPhone X 上报错,原因是电池电量值无法转换为 int 型。出现的情况是偶发性的,但一旦出现,一段时间内会一直出现(可能等电量值更新了才会正常吧)。不知道是 uni-app 的 bug 还是微信小程序的 bug。问了度娘和必应国际,暂时没有找到答案。
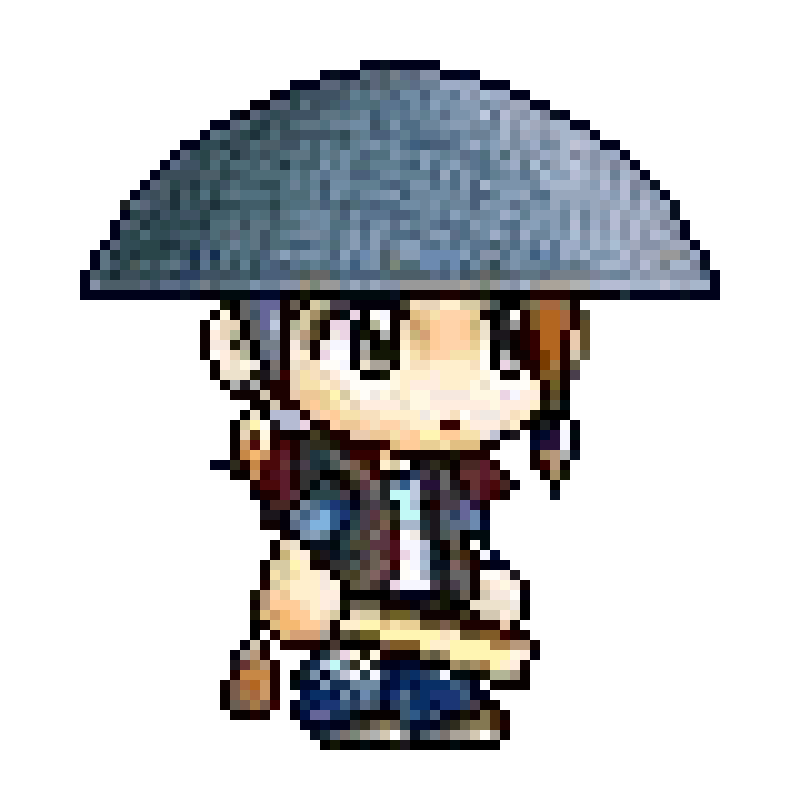
当我们用 ASP.NET Web API 作服务端接口时,如果客户端以 GET 方式请求的 URL 中,由于拼接错误导致“?”和“&”并列出现,会发生 400 Bad Request,那么需要在 IIS 中使用 URL 重写模块来纠正。
请求 URL 如:
http://www.abc.com/api/obj?&foo=bar
需要重写为:
http://www.abc.com/api/obj?foo=bar
打开 IIS 管理器中对应网站的 URL 重写,
添加空白规则。
匹配的 URL 不包含域名,如上述 URL 则匹配的范围是:
api/obj
不包含我们要查找的字符串 ?&,所以此处模式只能匹配所有:
.*
又因为重定向路径中需要包含这部分,所以用括号包裹,即:
(.*)
继续添加条件匹配:
条件输入:{QUERY_STRING},表示匹配查询字符串,
匹配的部分是“?”后面的字符串:
&foo=bar
那么可以填写模式:
^\&.*
因为 & 后面的部分仍然需要使用,所以用括号包裹:
^\&(.*)
“操作”中选择类型“重定向”,URL 填写:
{R:1}?{C:1}
其中 {R:1} 指代 api/obj,{C:1} 指代 foo=bar
取消“附加查询字符串”前面的勾
重定向类型在测试时可以选择 302 或 307,没问题后选择 301。
完成。
最终我们可以在网站的配置文件 web.config 中看到:
<rewrite>
<rules>
<rule name="uni-app-h5-replace-q" stopProcessing="true">
<match url="(.*)" />
<conditions>
<add input="{QUERY_STRING}" pattern="^\&(.*)" />
</conditions>
<action type="Redirect" url="{R:1}?{C:1}" appendQueryString="false" redirectType="Temporary" />
</rule>
</rules>
</rewrite>
我们要把这段代码复制到开发环境源代码的 web.config 中,以免发布网站时将我们设置好的配置覆盖掉。
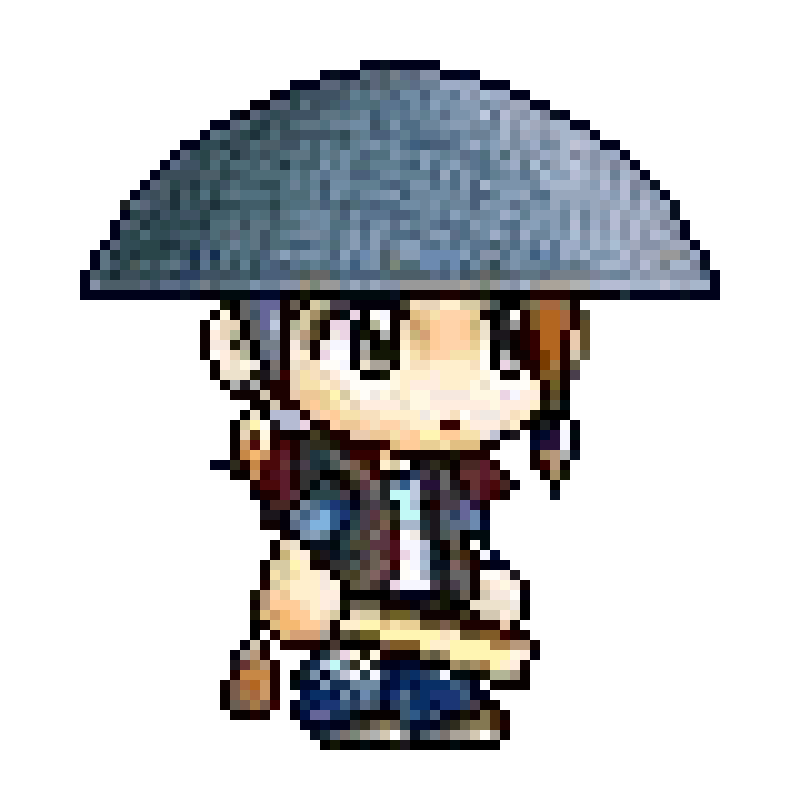
uni-app 的 input,当 type = "text" 时,设置 confirm-type = "next" 可以提供键盘的“下一项”,通过 @confirm 触发设置下一个文本框的焦点,即可实现只操作键盘即可完成表单填写。
我们以从 a 组件跳转到 b 组件为例:
<input v-model="a" type="text" confirm-type="next" @confirm="moveNext('b')" />
uni-app 的组件只有属性和事件,不提供方法,那么我们只能设置提供了 focus 属性的组件的焦点。
<input v-model="b" :focus="focusList['b']" />
moveNext() 方法实现:
moveNext(dom){
this.focusList[dom] = true;
}
当然 focusList 必须先定义并初始化,否则设置 focus 为 true 将失效:
data() {
return {
a: '',
b: '',
focusList: {
b: false
}
}
}
几个注意点:
confirm-type = "next" 仅支持微信小程序
“下一项”按钮效果是一次性的,使用一次后再次在 a 上触摸“下一项”将不再跳转到 b,即使在 moveNext() 中先设置为 false 再设置为 true 也没用
其它组件也可以通过自带的方法实现跳转到带 focus 属性的组件(如在 picker 的 @change 中设置 this.focusList['b'] = true)
限制于 confirm-type = "next" 只当 type = "text" 时有效,因此非文本键盘(如数字键盘)就不能提供“下一项”功能
textarea 组件的右下角始终是“换行”,由其右上角的“完成”按钮来实现“下一项”功能
textarea 组件在“下一项”跳转过程中,组件可能会被 tabBar 遮挡(几率很大)
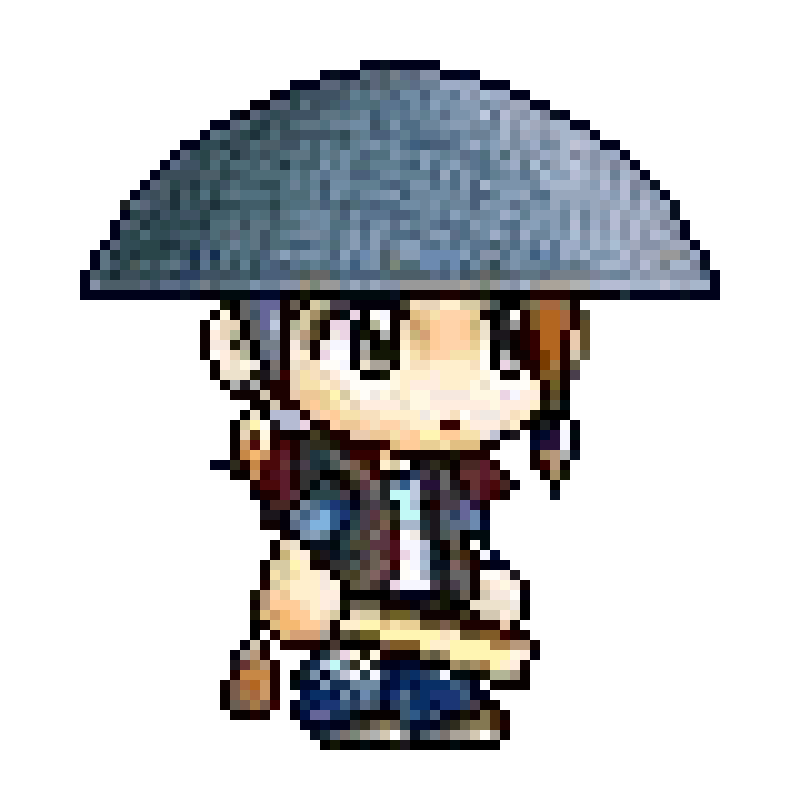
本文不定时更新中……
收集了一些在开发过程中遇到的一些问题的解决方法,适合新手。
异常:
出现脚本错误或者未正确调用 Page()
原因:不小心删了第一行内容:<template>
异常:
模块编译失败:TypeError: Cannot read property 'for' of undefined
at fixDefaultIterator (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4277:24)
at mark (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4306:5)
at markComponent (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4371:5)
at baseCompile (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4384:15)
at compile (D:\HBuilderX\plugins\uniapp\lib\mpvue-template-compiler\build.js:4089:28)
at Object.module.exports (D:\HBuilderX\plugins\uniapp\lib\mpvue-loader\lib\template-compiler\index.js:43:18)
原因:新建的页面(简单模板)只有以下 3 个标签,须在 <template /> 中添加一些代码,如 <view />
<template>
</template>
<script>
</script>
<style>
</style>
异常:
模块编译失败:TypeError: Cannot read property 'toString' of undefined
at Object.preprocess (D:\HBuilderX\plugins\uniapp\lib\preprocess\lib\preprocess.js:56:15)
at Object.module.exports (D:\HBuilderX\plugins\uniapp\lib\preprocessor-loader.js:9:25)
原因:没有原因,纯抽风,HX 关掉再开就好了。
异常:
Cannot set property 'xxx' of undefined;at pages/... onLoad function;at api request success callback function
原因:属性未定义,例如
data() {
return {
item: { }
}
}
而直接赋值 this.item.abc.xxx = '123';
解决:
data() {
return {
item: {
abc: ''
}
}
}
问:page 页面怎样修改 tabBar?
答:官方文档未给出答案,百度了一圈也无果(2018-10-23),但有人说小程序的 setTabBarBadge() 方法设置角标是可以用的。
坑:
VM1694:1 获取 wx.getUserInfo 接口后续将不再出现授权弹窗,请注意升级
参考文档: https://developers.weixin.qq.com/community/develop/doc/0000a26e1aca6012e896a517556c01
填坑:放弃使用 uni.getUserInfo 接口来获取用户信息,uni.login 可返回用于换取 openid / unionid 的 code,参:uni.login、 code2Session
坑:字符搜索(当前目录)(Ctrl+Alt+F)搜不出所有结果
填坑:顾名思义他只搜索当前目录,即当前打开文件所在目录,而非我误认为的整个项目根目录。在“项目管理器”中选中要搜索字符的目录即可。
坑:uni.navigateTo() 或 uni.redirectTo() 没反应
填坑:这两个方法不允许跳转到 tabbar 页面,用 uni.switchTab() 代替。
坑:使用“Ctrl+/”快捷键弹出“QQ五笔小字典”窗口
解决:打开QQ五笔“属性设置”,切换到“快捷键设置”选项卡,把“五笔小字典”前的勾取消(即使该组合键是设置为Ctrl+?)。
坑:<rich-text /> 中的 <img /> 太大,超出屏幕宽度
填坑:用正则表达式给 <img /> 加上最大宽度
data.data.Content = data.data.Content.replace(/\<img/gi, '<img style="max-width:100%;height:auto" ');
坑:无法重命名或删除目录或文件
填坑一:“以管理员身份运行”HBuilder X 后再试。
填坑二:关闭微信开发者工具、各种手机和模拟器后再试。
填坑三:打开“任务管理器”,结束所有“node.exe”进程后再试。
坑:
thirdScriptError
sdk uncaught third Error
(intermediate value).$mount is not a function
TypeError: (intermediate value).$mount is not a function
Page[pages/xxxx/xxxx] not found. May be caused by: 1. Forgot to add page route in app.json. 2. Invoking Page() in async task.
Page is not constructed because it is not found.
填坑:关闭微信开发者工具、各种手机和模拟器后,删除“unpackage”目录。
坑:
Unexpected end of JSON input;at "pages/news/view" page lifeCycleMethod onLoad function
SyntaxError: Unexpected end of JSON input
填坑:给 uni.navigateTo() 的 url 传参时,如果简单地将对象序列化 JSON.stringify(item),那么如果内容中包含“=”等 url 特殊字符,就会发生在接收页面 onLoad() 中无法获取到完整的 json 对象,发生异常。
uni.navigateTo({
url: "../news/view?item=" + JSON.stringify(item)
})
所以应该把参数值编码:
uni.navigateTo({
url: "../news/view?item=" + escape(JSON.stringify(item))
})
如果是一般的 web 服务器来接收,那么会自动对参数进行解码,但 uni-app 不会,如果直接使用:
onLoad(e) {
this.item = JSON.parse(e.item);
}
会发生异常:
Unexpected token % in JSON at position 0;at "pages/news/view" page lifeCycleMethod onLoad function
SyntaxError: Unexpected token % in JSON at position 0
需要解码一次:
onLoad(e) {
this.item = JSON.parse(unescape(e.item));
}
需要注意的是,unescape(undefined) 会变成 'undefined',如果要判断是否 undefined,应是 unescape 之前。
坑:图片变形
填坑:mode="widthFix"
坑:页面如何向 tabBar 传参
填坑:全局或缓存
坑:编译为 H5 后,出现:Access-Control-Allow-Origin
填坑:参阅
坑:编译为 H5 后,GET 请求的 URL 中出现“?&”
填坑 :客户端只求 DCloud 官方能够尽快修复这个 bug,IIS 端可以暂时用 URL 重写来防止报 400 错误,参此文。
坑:[system] errorHandler TypeError: Cannot read property 'forEach' of undefined
填坑:待填
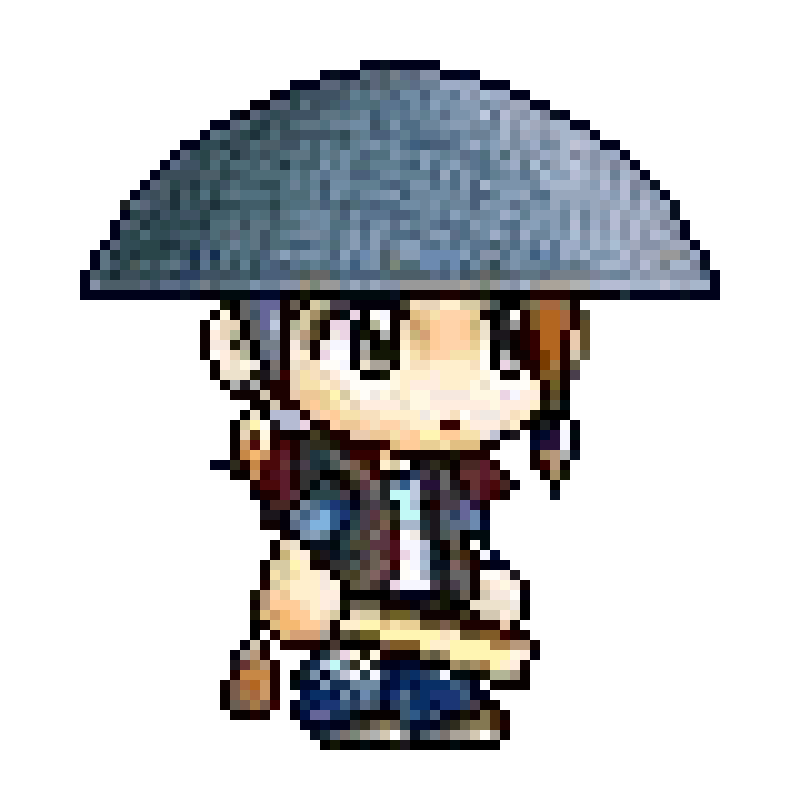